Question
Introduction to C Programming Objectives Assignment #7 Introduction to C Programming COP 3223 1. To learn how to write functions given specifications 2. To learn
Introduction to C Programming
Objectives
Assignment #7 Introduction to C Programming COP 3223
1. To learn how to write functions given specifications 2. To learn how to the use pass by value and pass by reference variables 3. Review previous concepts like if-statements and loops.
Introduction: Ninja Academy
Ninjas are awesome! Your friend has not stopped talking about how cool ninjas and how they would like to become a ninja. To amuse your friend, you have decided to create a series of programs about ninjas.
Problem: Codebreaking! (codebreaker.c)
You are almost ready to graduate from the Ninja Academy. Only one set of lessons remains: code breaking. You will need to learn how to break numerical codes of varying lengths and difficulties.
The basic idea is this:
1) You begin by selecting a length and difficulty for the code. A set of numbers will be generated.
2) You will guess the magnitude and placement of each of the numbers.
3) Anything exactly correct will be revealed.
4) Anything with the correct value will be noted, but not revealed.
5) You can guess as many times as you want.
When the correct code is entered, the program will end.
A scaffold for this program has been provided on the webcourse. You must fill in the eight functions that are currently empty. After you write each function, you should test it before moving on. The main function should not be modified for the final submission (you may modify it during testing, as long as you return it to its initial form).
Descriptions of each function are given in the scaffold along with the function Pre- and Post-conditions. The output samples at the end of this document show the wording you should use and how the program should run when completed.
Deliverables
One source file: codebreaker.c for your solution to the given problem submitted over WebCourses.
Restrictions
Although you may use other compilers, your program must compile and run using Code::Blocks. Your program should include a header comment with the following information: your name, course number, section number, assignment title, and date. Also, make sure you include comments throughout your code describing the major steps in solving the problem.
Grading Details
Your programs will be graded upon the following criteria:
1) Your correctness
2) Your programming style and use of white space. Even if you have a plan and your program works perfectly, if your programming style is poor or your use of white space is poor, you could get 10% or 15% deducted from your grade.
3) Compatibility You must submit C source files that can be compiled and executed in a standard C Development Environment. If your program does not compile, you will get a sizable deduction from your grade.
Output Sample #1 Welcome to Codebreaker Training! You will be presented with a set of hidden values. Your job is to guess these values and their correct order. After each guess you will be given feedback.
Values that are correct in magnitude and in the correct location will be revealed. These are perfect matches.
Thenyouwillbetoldhowmanyothernumbersarecorrectinmagnitudeonly. Theseareimperfect matches. No additional information will be revealed.
Choose a length for the code (5-10 numbers).
6
Choose a difficulty (1, 2, or 3).
1
There are 6 numbers ranging from 1 to 3.
------ Enter your guess: 111111 You have 1 perfect matches and 0 imperfect matches.
----1- Enter your guess: 232112 You have 1 perfect matches and 2 imperfect matches.
----1- Enter your guess: 222113 You have 3 perfect matches and 0 imperfect matches.
-2--13 Enter your guess: 323313 You have 6 perfect matches and 0 imperfect matches.
Congratulations! You guessed all the values!
Output Sample #2 Welcome to Codebreaker Training! You will be presented with a set of hidden values. Your job is to guess these values and their correct order. After each guess you will be given feedback.
Values that are correct in magnitude and in the correct location will be revealed. These are perfect matches.
Thenyouwillbetoldhowmanyothernumbersarecorrectinmagnitudeonly. Theseareimperfect matches. No additional information will be revealed.
Choose a length for the code (5-10 numbers).
3
Choose a length for the code (5-10 numbers).
11
Choose a length for the code (5-10 numbers).
5
Choose a difficulty (1, 2, or 3).
0
Choose a difficulty (1, 2, or 3).
5
Choose a difficulty (1, 2, or 3).
3
There are 5 numbers ranging from 1 to 9.
----- Enter your guess: 12345 You have 2 perfect matches and 1 imperfect matches.
12--- Enter your guess: 12434 You have 3 perfect matches and 0 imperfect matches.
124-- Enter your guess: 12467 You have 3 perfect matches and 1 imperfect matches.
124-- Enter your guess: 12476 You have 4 perfect matches and 0 imperfect matches.
124-6
Enter your guess: 12486 You have 4 perfect matches and 0 imperfect matches.
124-6 Enter your guess: 12496 You have 5 perfect matches and 0 imperfect matches.
Congratulations! You guessed all the values!
//pre-processor directives
#include
#include
#include
//Function Prototypes - do not change these
void greeting();
void setParameters(int * length, int * difficulty);
int * createHideList(int length, int difficulty);
int * createShowList(int length);
void display(int showlist[], int length);
void getGuess(int guesss[], int length);
void processGuess(int hidelist[], int showlist[], int length, int guess[]);
int solved(int hidelist[], int guess[], int length);
int PerfectMatches(int hidelist[], int guess[], int length);
int ImperfectMatches(int hidelist[], int guess[], int length);
void copyArray(int dest[], int source[], int length);
//Main function - This is the final version of main. Any changes you make while
//creating the functions should be removed prior to submission.
int main() {
//Length determines the number of hidden values that must be guessed
//Difficulty determines the range of the hidden values
int length, difficulty;
//Answer, revealed, and guess are all integer arrays
//Since length is undefined at this point, no sizes have been assigned.
//This will be done in the create...List functions.
//Answer is the correct answer of the hidden values
//Revealed is the list that is shown to the user
//Guess is the user's guess
int * answer, * revealed, * guess;
//seed the random number generator
srand(time(0));
//Begin the program by showing the initial greeting to the user
greeting();
//Ask the user for the length and difficulty
setParameters(&length, &difficulty);
//Create the initial arrays of size length
answer = createHideList(length, difficulty);
revealed = createShowList(length);
guess = createShowList(length);
printf(" There are %d numbers ranging from 1 to %d. ", length, difficulty*3);
//Loop until the user solves the puzzle
while (!solved(answer, guess, length)) {
//Show the information gathered so far and prompt the user for their next guess
display(revealed, length);
getGuess(guess, length);
processGuess(answer, revealed, length, guess);
}
printf("Congratulations! You guessed all the values! ");
//These functions are necessary because we are using memory allocation functions in create...List
free(answer);
free(revealed);
free(guess);
return 0;
}
//Functions
//Pre-conditions: none
//Post-conditions: Prints a welcome message to the screen
void greeting() {
printf("Welcome to Codebreaker Training! ");
printf("You will be presented with a set of hidden values. Your job is to guess these ");
printf("values and their correct order. After each guess you will be given feedback. ");
printf("Values that are correct in magnitude and in the correct location will be revealed. These are ");
printf("perfect matches. Then you will be told how many other numbers are correct in magnitude only. ");
printf("These are imperfect matches. No additional information will be revealed. ");
return;
}
//Pre-conditions: length and difficulty are valid values established by the setParameters function
//Post-conditions: create an array of size length and populate it with random
// values based on the difficulty
int * createHideList(int length, int difficulty) {
//i is a counter
//array is an integer array, allocated "length" integers
int i;
int * array = (int *)malloc(sizeof(int) * length);
//traverse the valid indices of the array
//assign each index a random value from 1 - difficulty*3
for(i=0; i array[i] = rand() % (difficulty*3) + 1; //return the beginning of the array to the main function return array; } //Pre-conditions: length has a valid value established by the setParameters function //Post-conditions: create an array of size length and initialize to zero int * createShowList(int length){ //i is a counter //array is an integer array, allocated "length" integers int i; int * array = (int *)malloc(sizeof(int) * length); //traverse the valid indices of the array //assign each index an initial value of 0 for(i=0; i array[i] = 0; //return the beginning of the array to the main function return array; } //Pre-conditions: length and difficulty are integer pointers //Post-conditions: length and difficulty will be set by the user // Function should verify that these values are valid //What to do in this function: Prompt the user for the length of the code. Remember //that the parameter length is a pointer, so it cannot store an integer value. You will need additional //variables. Ensure that the user gives you a value between 5 and 10, inclusive. If their //value is invalid, continue to prompt them until they provide a valid one. Assign length the location //of the valid integer. Repeat this process for the difficulty parameter. Difficulty //must be 1, 2, or 3 to continue. void setParameters(int * length, int * difficulty) { } //Pre-conditions: showlist is an integer array of size length //Post-conditions: displays the contents of showlist using - for 0 //What to do in this function: Traverse the showlist array and print a - whenever the value is 0 //If the value is not zero, print the actual value. For example, if the code is 12311 and the //user has identified the location of the 1s, the showlist/revealed array will contain 10011 //and you should display 1--11 void display(int showlist[], int length) { } //Pre-conditions: guess is an integer array of size length //Post-conditions: reads in the guess from a user as a string and converts it to an integer array //What to do in this function: The user will enter their values all together. For example: 12311 //Read this in as a string and then store each individual integer value in the guess array. //Since the elements of the input will be characters, you will need to convert to integers. For example //you would need to convert from '1' to 1. Character representations of digits start with '0' with ASCII value 48. void getGuess(int guess[], int length) { } //Pre-conditions: both hidelist and guess are integer arrays of size length // hidelist represents the answer the user is looking for // guess represents the user's current guess //Post-conditions: determines if the user has found all of the hidden values //What to do in this function: Traverse the arrays and check to see if all of the values //in hidelist are the same as the values in guess. If they are return 1 for true, //return 0 for false otherwise. int solved(int hidelist[], int guess[], int length) { } //Pre-conditions: hidelist, showlist, and guess are integer arrays of size length // hidelist represents the answer the user is looking for // showlist shows the values the user has already discovered // guess is the user's current guess //Post-conditions: evaluates the current guess and updates showlist //What to do in this function: Compare hidelist to guess. If any values match exactly, //update the showlist to reflect the information the user has discovered. Then, print out //the number of perfect matches the user has by calling the PerfectMatches function. Print //out the number of imperfect matches the user has by calling the ImperfectMatches function. //Use the statement from the samples for your printf control string. void processGuess(int hidelist[], int showlist[], int length, int guess[]) { } //Pre-conditions: hidelist and guess are integer arrays of size length // hidelist represents the answer the user is looking for // guess is the user's current guess //Post-conditions: evaluates the current guess and returns the number of perfect matches //What to do in this function: Compare hidelist to guess. Return the number of times //the value in guess exactly matches the corresponding value in hidelist. int PerfectMatches(int hidelist[], int guess[], int length) { } //Pre-conditions: hidelist and guess are integer arrays of size length // hidelist represents the answer the user is looking for // guess is the user's current guess //Post-conditions: evaluates the current guess and returns the number of imperfect matches //What to do in this function: Create temporary copies of both arrays by calling the copyArray function //twice. This is necessary because we'll need to make changes to both arrays without modifying the //originals. Mark out the spots on the arrays that match exactly. They will be counted in the perfect //matches instead. Use any value that won't be present in the hidelist. //Go through the hidelist array and look for matches in the guess array. Anytime a value matches, //regardless of location, count it as an imperfect match and mark it so it won't get counted again. //Return the number of imperfect matches //Hint: you will need a nested for loop. int ImperfectMatches(int hidelist[], int guess[], int length) { } //Pre-conditions: dest and source are integer arrays of size length // source represents a source array: an array to be copied // dest represents a desitnation array: the location of the copy //Post-conditions: dest will contain a copy of source //What to do in this function: travers the source array and copy each element into //the corresponding location of the dest array void copyArray(int dest[], int source[], int length) { }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
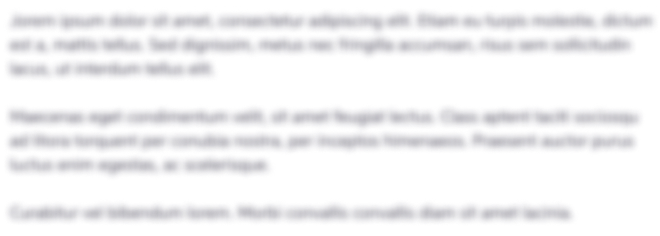
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started