Question
Is my final code compiling correctly for Python? In this assignment, you will work with arrays and linked lists of nodes. Begin with the useArraysAndLinkedNodes.py
Is my final code compiling correctly for Python?
In this assignment, you will work with arrays and linked lists of nodes.
Begin with the "useArraysAndLinkedNodes.py" starter file. This file contains comment instructions that tell you where to add your code to do various tasks that use and manipulate arrays and linked lists of nodes.
Make sure you read the Guidance" document.
Make sure your output matches the " Expected Output" document.
File: useArraysAndLinkedNodes.py
This program exercises arrays and linked nodes.
The following files must be in the same folder: arrays.py node.py """
#
# Replace
from arrays import Array from node import Node
# Part 1: # This function prints a linked list of nodes. # Parameters: # head - a reference to the first node in the linked list. def printLinkedList(head): # Print the linked list with each nodes's data on a separate line. # You must use some form of a loop to print the linked list. #
# Here is the array: theArray = Array(10) for i in range(len(theArray)): theArray[i] = i + 1
# Print the array: print("The array:") print(theArray) print() # blank line
# You must use these variables in your code: head = Node(theArray[0], None) tail = head
# Part 2: # Copy the array items to a linked list of nodes: # The linked list must consist of Node class items. # You must use some form of a loop to create the linked list. #
print("After creating the list from the array items:") printLinkedList(head) print() # blank line
# Part 3: # Add a node with the value 99 to the end of the list: #
print("After adding 99 to the end of the list:") printLinkedList(head) print() # blank line
# Part 4: # Add a node with the value 55 after the node with the value 5: # You must go through the list until you arrive at the 5, # then link in the new node correctly. #
print("After adding 55 after the node with the value 5:") printLinkedList(head)
Programming Activity 2 - Guidance As you should do every week, make sure you study this week's programming examples. The starting contents of a data structure includes both its size and item values. The expected output is based on the starting contents of theArray. You are NOT allowed to hard-code an answer to any part. Two variables are initialized for you in the starter code. They are named head and tail. head always references the first node in a linked list. tail always references the last node in a linked list. You must use these variables in your code. Part 1 ------ Start at head and traverse (loop) through the linked nodes. Print each node's data value as you encounter it. Use a variable named currentNode as you traverse the list. In your loop, to print the current node's value, use: print(currentNode.data) As you loop, you must update currentNode to reference the next item. Part 2 ------ Use tail to add nodes to the end of the list, one-by-one in a loop. As they are added, you must update tail so it always references the last node. The variable head should not be used during this step. Part 3 ------ Create the new node. Then, change tail's next link to reference it. Part 4 ------ Read the comments in the starter code. Use a variable named currentNode as you traverse the list. To link the new node in correctly: the new node's next link must be set to currentNode's next link, then currentNode's next link must be set to reference the new node.
Expected Output:
The array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] After creating the list from the array items: 1 2 3 4 5 6 7 8 9 10 After adding 99 to the end of the list: 1 2 3 4 5 6 7 8 9 10 99 After adding 55 after the node with the value 5: 1 2 3 4 5 55 6 7 8 9 10 99
Final code:
from arrays import Array
from node import Node
def printLinkedList(head):
while head:
print(head.dataval)
head=head.nextval
theArray=Array(10)
for i in range(len(theArray)):
theArray[i]=i+1
print("The array:")
print(theArray)
print()
head=Node(theArray[0],None)
tail=head
for i in range(1,len(theArray)):
temp=Node(theArray[i],None)
tail.nextval=temp
tail=temp
print("After creating the list from the array iteams:")
printLinkedList(head)
print()
temp=Node(99,None)
tail.nextval=temp
tail=temp
print("After adding 99 to the end of the list:")
printLinkedList(head)
print()
a=head
while a:
if a.datval==5:
temp=Node(55,None)
b=a.nextval
a.nextval=temp
temp.nextval=b
if !b:
tail=temp
print("After adding 55 after the node with the value 5:")
printLinkedList(head)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
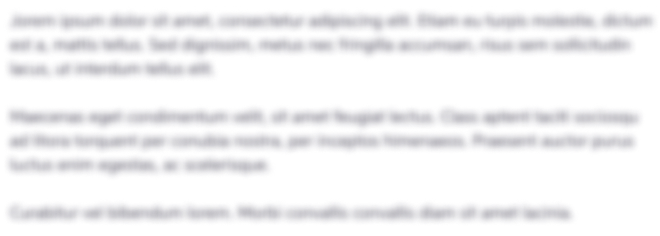
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started