Question
//is there a more efficient way to write out these functions in c++ ? without using include //Looking at the methods I have, can you
//is there a more efficient way to write out these functions in c++ ? without using include
//Looking at the methods I have, can you show me a different way of doing it.
//Like can you do ListItr itr1.current=head->next; all in one line?
//note: the list holds integers. there must be a List Itr and Listnode (i've copy the code from the list itr file so you can get a better understanding)
// List c plus plus file
void List::makeEmpty(){ ListNode *temp=head->next; while(temp!=tail){ temp=temp->next; delete temp->previous; temp->next=temp->next; temp->previous->next=temp->next; } count=0; }
ListItr List::first(){ ListItr itr1; itr1.current=head->next; return itr1; }
ListItr List::last(){ ListItr itr1; itr1.current=tail->previous; return itr1; }
void List::insertAfter(int x, ListItr position){ ListNode *pos=position.current; ListNode *newNode=new ListNode; ListNode *nextNode=pos->next; newNode->next=nextNode; newNode->previous=pos; newNode->value=x; pos->next=newNode; nextNode->previous=newNode; count++; }
void List::insertBefore(int x, ListItr position){ ListNode *pos=position.current; ListNode *newNode=new ListNode; ListNode *prevNode=pos->previous; newNode->next=pos; newNode->previous=prevNode; newNode->value=x; prevNode->next=newNode; pos->previous=newNode; count++; }
void List::insertAtTail(int x){ ListNode *newNode=new ListNode; ListNode *prevNode=tail->previous; newNode->next=tail; newNode->value=x; prevNode->next=newNode; tail->previous=newNode; newNode->previous=prevNode; count++; }
void List::remove(int x){ ListItr itr1=find(x); ListNode *pos=itr1.current; ListNode *prevNode=pos->previous; ListNode *nextNode=pos->next; prevNode->next=nextNode; nextNode->previous=prevNode; count--; }
ListItr List::find(int x){ ListItr itr1=first(); while(!itr1.isPastEnd()){ if(itr1.current->value==x){ return itr1;} else{ itr1.moveForward(); } } return itr1; }
int List::size() const{ return count; }
void printList(List& theList, bool forward){ ListItr itr1=theList.first(); ListItr itr2=theList.last(); if (forward){ while(!itr1.isPastEnd()){ cout << itr1.retrieve(); itr1.moveForward();} } else{ while(!itr2.isPastBeginning()){ cout << itr2.retrieve(); itr2.moveBackward();}} }
__________________________________________________________________
// This is the List iterator c plus plus file #include #include #include #include #include "ListItr.h" using namespace std;
//constructor ListItr::ListItr(){ current=new ListNode(); }
// One parameter constructor ListItr::ListItr(ListNode* theNode){ current=theNode; }
//returns if the itr is past the end bool ListItr::isPastEnd() const{ return (current->next==NULL); }
//returns if the itr is past the beginning bool ListItr::isPastBeginning() const{ return (current->previous==NULL); }
//moves the itr forward void ListItr::moveForward(){ if(this->isPastEnd()!=true){ current=current->next; }}
//moves the itr backwards void ListItr::moveBackward(){ if(this->isPastBeginning()!=true){ current=current->previous;} }
//returns value at the itr's current node int ListItr::retrieve() const{ return current->value; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
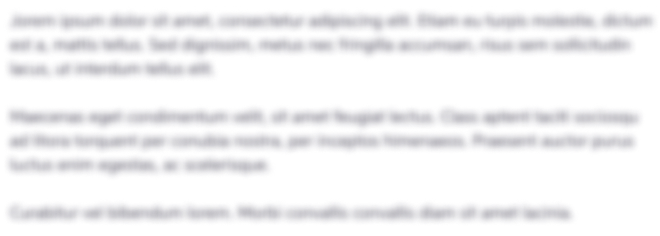
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started