Question
Is there a way to implement Stack to perform calculations with this code? import java.awt.EventQueue; import javax.swing.JFrame; import javax.swing.JTextField; import javax.swing.JButton; import java.awt.Font; import java.awt.event.ActionListener;
Is there a way to implement Stack to perform calculations with this code?
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.JButton;
import java.awt.Font;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.SwingConstants;
public class Calculator {
private JFrame frame;
private JTextField textField;
double input1;
double input2;
double result;
String operation;
String answer;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Calculator window = new Calculator();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public Calculator() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 246, 362);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
textField = new JTextField();
textField.setHorizontalAlignment(SwingConstants.RIGHT);
textField.setBounds(6, 6, 232, 36);
frame.getContentPane().add(textField);
textField.setColumns(10);
//Row 1
JButton btn7 = new JButton("7");
btn7.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn7.getText();
textField.setText(input);
}
});
btn7.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn7.setBounds(6, 44, 50, 50);
frame.getContentPane().add(btn7);
JButton btn8 = new JButton("8");
btn8.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn8.getText();
textField.setText(input);
}
});
btn8.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn8.setBounds(68, 44, 50, 50);
frame.getContentPane().add(btn8);
JButton btn9 = new JButton("9");
btn9.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn9.getText();
textField.setText(input);
}
});
btn9.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn9.setBounds(128, 44, 50, 50);
frame.getContentPane().add(btn9);
JButton btnPlus = new JButton("+");
btnPlus.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
input1 = Double.parseDouble(textField.getText());
textField.setText("");;
operation = "+";
}
});
btnPlus.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnPlus.setBounds(188, 44, 50, 50);
frame.getContentPane().add(btnPlus);
//Row 1
//Row 2
JButton btn4 = new JButton("4");
btn4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn4.getText();
textField.setText(input);
}
});
btn4.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn4.setBounds(6, 104, 50, 50);
frame.getContentPane().add(btn4);
JButton btn5 = new JButton("5");
btn5.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn5.getText();
textField.setText(input);
}
});
btn5.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn5.setBounds(68, 104, 50, 50);
frame.getContentPane().add(btn5);
JButton btn6 = new JButton("6");
btn6.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn6.getText();
textField.setText(input);
}
});
btn6.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn6.setBounds(128, 104, 50, 50);
frame.getContentPane().add(btn6);
JButton btnSub = new JButton("-");
btnSub.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
input1 = Double.parseDouble(textField.getText());
textField.setText("");;
operation = "-";
}
});
btnSub.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnSub.setBounds(188, 104, 50, 50);
frame.getContentPane().add(btnSub);
//Row 2
//Row 3
JButton btn1 = new JButton("1");
btn1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn1.getText();
textField.setText(input);
}
});
btn1.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn1.setBounds(6, 164, 50, 50);
frame.getContentPane().add(btn1);
JButton btn2 = new JButton("2");
btn2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn2.getText();
textField.setText(input);
}
});
btn2.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn2.setBounds(68, 164, 50, 50);
frame.getContentPane().add(btn2);
JButton btn3 = new JButton("3");
btn3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn3.getText();
textField.setText(input);
}
});
btn3.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn3.setBounds(128, 164, 50, 50);
frame.getContentPane().add(btn3);
JButton btnM = new JButton("*");
btnM.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
input1 = Double.parseDouble(textField.getText());
textField.setText("");;
operation = "*";
}
});
btnM.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnM.setBounds(188, 164, 50, 50);
frame.getContentPane().add(btnM);
//Row 3
//Row 4
JButton btn0 = new JButton("0");
btn0.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btn0.getText();
textField.setText(input);
}
});
btn0.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btn0.setBounds(6, 224, 50, 50);
frame.getContentPane().add(btn0);
JButton btnPeriod = new JButton(".");
btnPeriod.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btnPeriod.getText();
textField.setText(input);
}
});
btnPeriod.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnPeriod.setBounds(68, 224, 50, 50);
frame.getContentPane().add(btnPeriod);
JButton btnPM = new JButton("+/-");
btnPM.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
double pm = Double.parseDouble(String.valueOf(textField.getText()));
pm = pm*(-1);
textField.setText(String.valueOf(pm));
}
});
btnPM.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnPM.setBounds(128, 224, 50, 50);
frame.getContentPane().add(btnPM);
JButton btnEquals = new JButton("=");
btnEquals.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String answer;
input2 = Double.parseDouble(textField.getText());
if (operation == "+") {
result = input1 + input2;
answer = String.format("%.2f",result);
textField.setText(answer);
}else if (operation == "-") {
result = input1 - input2;
answer = String.format("%.2f",result);
textField.setText(answer);
}else if (operation == "*"); {
result = input1 * input2;
answer = String.format("%.2f",result);
textField.setText(answer);
}
}
});
btnEquals.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnEquals.setBounds(188, 224, 50, 50);
frame.getContentPane().add(btnEquals);
//Row 4
//Row 5
JButton btnDel = new JButton("?");
btnDel.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String delete = null;
if(textField.getText().length() > 0) {
StringBuilder sB = new StringBuilder(textField.getText());
sB.deleteCharAt(textField.getText().length() - 1);
delete = sB.toString();
textField.setText(delete);;
}
}
});
btnDel.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnDel.setBounds(6, 284, 50, 50);
frame.getContentPane().add(btnDel);
JButton btnClear = new JButton("C");
btnClear.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
textField.setText(null);
}
});
btnClear.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnClear.setBounds(68, 284, 50, 50);
frame.getContentPane().add(btnClear);
JButton btnMod = new JButton("%");
btnMod.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
input1 = Double.parseDouble(textField.getText());
textField.setText("");;
operation = "%";
}
});
btnMod.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnMod.setBounds(128, 284, 50, 50);
frame.getContentPane().add(btnMod);
JButton btnDiv = new JButton("/");
btnDiv.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String input = textField.getText() + btnDiv.getText();
textField.setText(input);
}
});
btnDiv.setFont(new Font("Lucida Grande", Font.PLAIN, 18));
btnDiv.setBounds(188, 284, 50, 50);
frame.getContentPane().add(btnDiv);
//Row 5
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
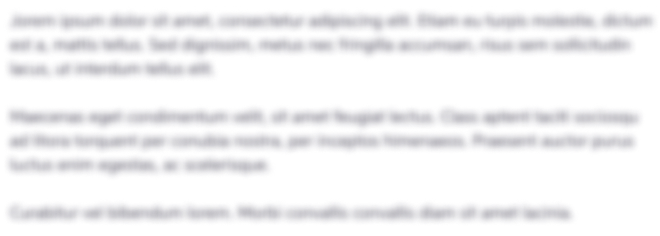
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started