Question
IT 212 -- Project 3 Goal: Implement and test Card and Deck classes as shown in the Card/Deck UML Diagram. Details: The ranks of the
IT 212 -- Project 3
Goal: Implement and test Card and Deck classes as shown in the Card/Deck UML Diagram. Details:
The ranks of the cards from lowest to highest:
2 3 4 5 6 7 8 9 10 J Q K A
The order of the suits from lowest to highest:
C D H S (Club Diamond Heart Spade)
Include two test scripts test1.rb and test2.rb.
test1.rb tests both the Card and Deck classes in the traditional way using print statements. Test all methods in the Card and Deck classes. test2.rb tests both the Card and Deck classes using the unit test framework with assert_equal methods. Write unit tests for all methods in the Card class, but you only have to test these methods in the Deck class: new, deal, add_to_bottom, add_to_top, count, empty? When writing unit tests for add_to_bottom and add_to_top, you only need to check that the count of the Deck object increases by 1; you don't need to check the actual contents of the Deck object after the method call. When writing unit tests for deal, just check that the count of the Deck object decreases by 1 and the Card object that is returned.
Important: Don't forget these items before submitting. (Up to a 10 point deduction if you forget them.)
Include a commented header at the top of each Ruby source code file with your name, project number, and submission date: Place each class in a file with the same name. For example, Card goes in the Card.rb file. The traditional test file is named test1.rb; the unit test file is named test2.rb. The four scripts in your zip file should be named Card.rb, Deck.rb, test1.rb, test2.rb. Before zipping up your submission, put the four scripts in a folder named Proj3Smith (replace Smith by your last name). Then zip up the folder to create Proj3Smith.zip. Only highlighting the scripts, then creating a zip file is not correct.
Grading: Functionality: 45% , Testing Completeness and Correctness: 30%, Indentation: 10%, Comments: 10%. Header at the top of each source code file with your name, project name, and submission date; scripts named correctly; zipfile named correctly: 10%.
Hints:
Double check the UML diagram to see if the parameters that you are passing in to each method is correct. Test your Card class completely before starting to write the Deck class. The bottom of the deck is index 0; the top of the deck is the maximum index (51 in the 52 card deck). Here are three Array methods that you may want to use in your implementation of the Deck class:
Add a new card onto the top of the deck: @cards.push(c)Here is how to create a new card with the specified rank and suit and append it to the array: c = Card.new(rank, suit) @cards << c # or c = Card.new(rank, suit) @cards.push(c) # or shorter @cards.push(Card.new(rank, suit)Remove a card from the top of the @cards array: @cards.popInsert a card c at the bottom of the deck (before the item with index 0): @cards.insert(0, c)When a card is inserted before index 0 or in the middle of the array, the index of each card that is greater than the insertion point is increased by 1 to make room for the insertion. Shuffle the Card objects in the deck: @cards.shuffle!
Use a double loop in the Deck#initialize method like this: @cards = [ ] for rank in 2..14 for suit in ['C', 'D', 'H', 'S'] # create a new card with the specified rank # and suit and append it to the array. @cards << Card.new(rank, suit) end endYou can use the array append operator << or you can use Array#push. Use a single loop to show all the cards in the Deck#to_s method: output = "" for card in @cards # Concatenate all of the cards # to the output variable endUse the += operator to concatenate all the cards to the output string. The ith card in the @cards array is @cards[i]. You will need to get the string representation of this card before appending it. Don't forget to put spaces between the cards. The last line in the body of the to_s method must be return outputMake sure that you don't print anything in the to_s methods. In the initialize method, define @symbols to be the array of possible symbols for rank shown on the card objects. These symbols are used in the to_smethod. @symbols = [nil, nil, '2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K', 'A']The Card class does not have setters for rank and suit because rank and suit should be read-only; you can't change the rank and suit of a card after the card is created. Cards in a new, unshuffled deck are placed in this order, bottom to top: 2C 3C 4C 5C 6C 7C 8C 9C 10C JC QC KC AC 2D 3D 4D 5D 6D 7D 8D 9D 10D JD QD KD AD 2H 3H 4H 5H 6H 7H 8H 9H 10H JH QH KH AH 2S 3S 4S 5S 6S 7S 8S 9S 10S JS QS KS ASWhenever you print something, always put a new line at the end unless there is a good reason not to. Including new lines at the end of your print statements makes your output more readable. To double space, do this: print d.count, " "The number of parameters you pass in to the constructor call (in this case Card.new) must match the number of parameters of the initialize method for that class. In the case of the Card class, c = Card.new(rank, suit)has two inputs because the header for initialize also has two parameters: def initialize(the_rank, the_suit)In you traditional test file test1.rb, do not print the return values of methods like Deck#add_to_top, Deck#add_to_bottom, Deck#shuffle!. For example, for Deck#add_to_top: # Don't do this: d = Deck.new c = Card.new(12, 'S') print d.add_to_top(c), " " # Do this: d = Deck.new c = Card.new(12, 'S') d.add_to_top(c) print d, " "To test the initialize and to_s methods of the Deck class, do this: d = Deck.new print d, " "(The method Deck#initialize does not have any parameters, so Deck.new should not have any parameters either.) Inheritance is not used for Project 3, for the first line of the Deck class: # Incorrect: class Deck < Card # Correct: class Deck
Step by Step Solution
There are 3 Steps involved in it
Step: 1
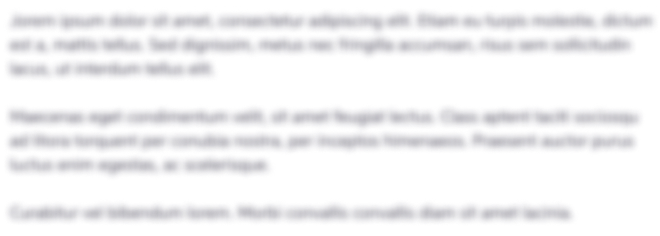
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started