Question
IT 280 Lab #5: Dictionary Validation Lab Instructions Program Inputs : A Chess Validation Dictionary (user created) A chessboard. The text discusses how to describe
IT 280 Lab #5: Dictionary Validation Lab
Instructions
Program Inputs:
A Chess Validation Dictionary (user created)
A chessboard.
The text discusses how to describe a chessboard.
Program Processing:
Validate the chess board for each validation item.
Program Output:
Print the results of each validation item.
e.g.
Fail: Number of black pieces 18 black pieces.
Pass: Number of white pieces 12 white pieces.
Pass: Kings One of each color.
Etc.
Please correct/fix the following python code to execute the dictionary validation chessboard and carry out the programs output as outlined above:
def isValidChessBoard(board):
piecesCount = {'b': 0, 'w': 0}
pawnCount = {'b': 0, 'w': 0}
hasKing = {'b': False, 'w': False}
letterAxis = ('a','b','c','d','e','f','g','h')
pieceColour = ('b','w')
pieceType = ('pawn','knight','bishop','rook','queen','king')
#each player has <= 16 pieces
for pos, i in board.items():
# check position value
#all pieces must be on valid space from '1a' to '8h'
if int(pos[0]) >= 9:
print('SpacesError')
return False
if pos[1] not in letterAxis:
print('yAxisError')
return False
# check piece data
if i != "":
#piece names begin with 'w' or 'b'
if i[0] not in pieceColour:
print('WhiteOrBlackError')
return False
thisPieceColour = i[0]
piecesCount[thisPieceColour] += 1
if piecesCount[thisPieceColour] >= 18:
print('TotalPieceError')
return False
#piece names must follow with 'pawn', 'knight', 'bishop', 'rook', 'queen', 'king'
thisPieceType = i[1:]
if thisPieceType not in pieceType:
print('PieceTypeError')
return False
elif thisPieceType == 'pawn':
pawnCount[thisPieceColour] += 1
#each player has <= 8 pawns
if pawnCount[thisPieceColour] >= 9:
print('PawnError')
return False
elif thisPieceType == 'king':
# one black king and one white king
if hasKing[thisPieceColour] == True:
print("AlreadyHasKingError")
hasKing[thisPieceColour] = True
if list(hasKing.values()) != [True, True]:
print("MissingKingError")
return False
return 'This board checks out'
board = {'1a': 'bking','2a': 'bqueen','3a': 'brook','4a': 'brook',
'5a': 'bknight','6a': 'bknight','7a':'bbishop','8a': 'bbishop',
'1b': 'bpawn','2b': 'bpawn','3b': 'bpawn','4b':'bpawn',
'5b': 'bpawn','6b': 'bpawn','7b': 'bpawn','8b': 'bpawn',
'1c': 'wking','2c': 'wqueen','3c': 'wrook','4c': 'wrook',
'5c': 'wbishop','6c': 'wbishop','7c': 'wknight','8c':'wknight',
'1e': 'wpawn','2e': 'wpawn','3e': 'wpawn','4e': 'wpawn',
'5e': 'wpawn','6e': 'wpawn','7e': 'wpawn','8e': 'wpawn',
'1f': '','2f': '','3f': '','4f': '','5f': '','6f': '','7f': '','8f': '',
'1g': '','2g': '','3g': '','4g': '','5g': '','6g': '','7g': '','8g': '',
'1h': '','2h': '','3h': '','4h': '','5h': '','6h': '','7h': '','8h': '',}
print(isValidChessBoard(board))
#validate chess board
dict_chess ={'1a':'bking', '8f':'wking', '1b':'bqueen', '6d': 'wqueen', \
'5c': 'wrook'}
chessHeightLocation = ['a','b','c','d','e','f','g','h']
chessWidthLocation = [1, 2, 3, 4, 5, 6, 7, 8]
colors = ['w', 'b']
pieces = ['pawn', 'knight', 'bishop', 'rook', 'queen', 'king']
def isValidChessBoard(dictChess):
playerCount = {'bpawn': 0, 'wpawn': 0, 'wking': 0, 'bking': 0, 'wpieceCount': 0, 'bpieceCount': 0}
for keysInChessBoard in dictChess:
#check for Space
#print(int(keysInChessBoard[0]) not in chessWidthLocation)
if (int(keysInChessBoard[0]) not in chessWidthLocation) or (keysInChessBoard[1] not in chessHeightLocation):
print('Not valid space: ' + keysInChessBoard)
return False
#check for black and white
if dictChess[keysInChessBoard][0] not in colors:
print('Not valid color of piece should have b for black or w for white: ' + dictChess[keysInChessBoard])
return False
#check for piece
if dictChess[keysInChessBoard][1:] not in pieces:
print('Not valid piece: ' + dictChess[keysInChessBoard])
return False
#check for pawns
if dictChess[keysInChessBoard] == 'bpawn' or dictChess[keysInChessBoard] == 'wpawn':
playerCount[dictChess[keysInChessBoard]] += 1
if playerCount[dictChess[keysInChessBoard]] > 8:
print('More than 8 pawns in one player')
return False
#check for king
if dictChess[keysInChessBoard] == 'bking' or dictChess[keysInChessBoard] == 'wking':
playerCount[dictChess[keysInChessBoard]] += 1
if playerCount[dictChess[keysInChessBoard]] > 1:
print('More than 1 king')
return False
#pieceCount
if dictChess[keysInChessBoard][0] in colors:
if dictChess[keysInChessBoard][0] == 'w':
playerCount[dictChess[keysInChessBoard][0]+'pieceCount'] += 1
elif dictChess[keysInChessBoard][0] == 'b':
playerCount[dictChess[keysInChessBoard][0]+'pieceCount'] += 1
if playerCount[dictChess[keysInChessBoard][0]+'pieceCount'] > 16:
print('More than 16 piece')
return False
#print(playerCount)
return True
print(isValidChessBoard(dict_chess))
Correct/fix the above python coding for Chess Validation Dictionary (chess board) and run the corrected coding in python.
Please post a screenshot of the code in python and write the corrected codes on Chegg using the appropriate python indentations.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
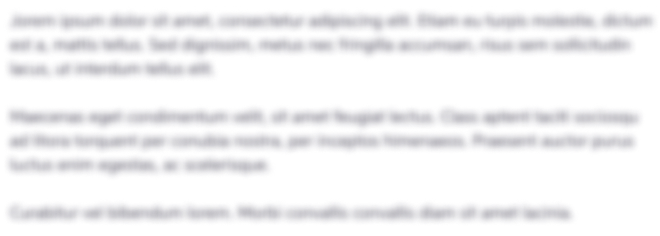
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started