Question
It is a bright, sunny day in AggieLand! Imagine you are walking around campus and trying to compute whether the sun is in your eyes,
It is a bright, sunny day in AggieLand! Imagine you are walking around campus and trying to compute whether the sun is in your eyes, or you can see your shadow in front of you, or neither.
Your work on this problem is to write a program that will do all of these steps:
1. Greet the user with this message (make sure to use println() to include a new line at the end): "It's a sunny day in AggieLand. Enter a direction (north, south, east or west) and a time (sunrise, noon, or sunset)."
2. Read the direction from System.in -- one of north, south, east or west
3. Read the time from System.in -- one of sunrise, noon or sunset.
4. If the direction is not one of these 4, print "I'm lost." and don't print anything else to the user.
5. If the time is not one of these 3, print "It's dark out." and don't print anything else to the user.
6. Print "The sun is in my eyes." if the direction is east at sunrise, if it's west at sunset.
7. Print "I can see my shadow in front of me." if the direction is west at sunrise, if it's east at sunset, or if it's north at noon.
8. If you have not printed anything in either of steps 6 or 7 above, then print "It's a beautiful day."
To make your program more resilient to user input, use String.equalsIgnoreCase() to compare two strings and let uppercase match lowercase. For instance this would let "NOON" and "noon" match, while String.equals() would only match "noon" with "noon", but not match "NOON" with "noon". You could also use String.toLowerCase() on the input to have the same effect.
Your program will be initially tested with a couple combinations of direction and time. But it will be graded based on all combinations AND good coding style (spacing, indentation, presence of comments).
SunnyDay.java
New
Full Screen
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
import java.util.Scanner;
// Specify the class of program.
public class SunnyDay {
// Opening main line needed for java.
public static void main(String[] args) {
// Define the message text to use in all output to the user.
final String kNorthMsg = "north";
final String kSouthMsg = "south";
final String kEastMsg = "east";
final String kWestMsg = "west";
final String kSunriseMsg = "sunrise";
final String kNoonMsg = "noon";
final String kSunsetMsg = "sunset";
final String kGreetingMsg1 = "It's a sunny day in AggieLand. ";
final String kGreetingMsg2 = "Enter a direction (north, south, east or west) ";
final String kGreetingMsg3 = "and a time (sunrise, noon, or sunset).";
final String kLostMsg = "I'm lost.";
2 Spaces
Automated Results
Run Tests
Below is the temple/ my attempt at the code. It shows both outputs for #4and 5 when it shouldnt.
import java.util.Scanner;
// Specify the class of program.
public class SunnyDay {
// Opening main line needed for java.
public static void main(String[] args) { // Define the message text to use in all output to the user. final String kNorthMsg = "north"; final String kSouthMsg = "south"; final String kEastMsg = "east"; final String kWestMsg = "west"; final String kSunriseMsg = "sunrise"; final String kNoonMsg = "noon"; final String kSunsetMsg = "sunset"; final String kGreetingMsg1 = "It's a sunny day in AggieLand. "; final String kGreetingMsg2 = "Enter a direction (north, south, east or west) "; final String kGreetingMsg3 = "and a time (sunrise, noon, or sunset)."; final String kLostMsg = "I'm lost."; final String kDarkMsg = "It's dark out."; final String kSunMsg = "The sun is in my eyes."; final String kShadowMsg = "I can see my shadow in front of me."; final String kBeautifulMsg = "It's a beautiful day."; //TODO: Greet user and get inputs. Add your comments/code below: System.out.println("It's a sunny day in AggieLand. Enter a direction (north, south, east or west) and a time (sunrise, noon, or sunset)."); java.util.Scanner scan = new java.util.Scanner(System.in); String direction = scan.next(); String time = scan.next(); //TODO: Figure out whether I am lost. Add your comments/code below: if ((direction.equals(kNorthMsg)) || (direction.equals(kSouthMsg)) || (direction.equals(kEastMsg)) || (direction.equals(kWestMsg))) { } else { System.out.println(kLostMsg); } //TODO: Figure out whether it is dark. Add your comments/code below: {if ((time.equals(kSunriseMsg)) || (time.equals(kNoonMsg)) || (time.equals(kSunsetMsg))) { } else { System.out.println(kDarkMsg); } //TODO: Figure out whether sun is in eyes and/or can see shadow. Add your comments/code below: //TODO: Handle all printing to the user. Add your comments/code below:
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
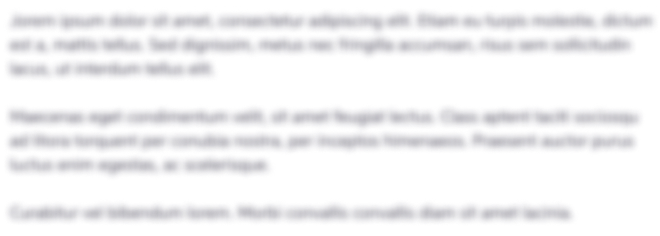
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started