Answered step by step
Verified Expert Solution
Question
1 Approved Answer
It is a question from a book called Essentials of Software Engineering 4th edition Chapter 1 Exercises 1.7 4) Download the programs for this chapter,
It is a question from a book called Essentials of Software Engineering 4th edition
Chapter 1 Exercises 1.7
4) Download the programs for this chapter, and add at least one more test case for each method of the StringSorter class. The methods are in TestStringSorter.java and one more test case has to be added to each method. Read, write and sort.
Sort1.java
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.io.Writer; import java.io.PrintWriter; import java.io.FileWriter; import java.util.List; import java.util.ArrayList; import java.util.Iterator; public class Sort1 { // given that we don't really know how to deal with a read/write error (IOException) // inside the functions, we'll let it pass through static List readFromStream(BufferedReader d) throws IOException { ArrayList lines=new ArrayList(); while(true) { String input=d.readLine(); if(input==null) break; lines.add(input); } return lines; } static void writeToStream(List l, Writer w1) throws IOException { PrintWriter w=new PrintWriter(w1); Iterator i=l.iterator(); while(i.hasNext()) { w.println((String)(i.next())); } } static void inPlaceSort(List l) { java.util.Collections.sort(l); } // the default behavior for exceptions is OK for this program public static void main(String args[]) throws IOException { if(args.length!=2) { System.out.println("Usage: java Sort1 inputfile outputfile"); } else { java.io.BufferedReader in=new BufferedReader(new FileReader(args[0])); Writer out=new FileWriter(args[1]); List l=readFromStream(in); inPlaceSort(l); writeToStream(l,out); in.close(); out.close(); } } }
StringSorter.java
import java.io.*; // for Reader (and subclasses), Writer (and subclasses) and IOException import java.util.*; // for List, ArrayList, Iterator public class StringSorter { ArrayList lines; // given that we don't really know how to deal with a read/write error (IOException) // inside the functions, we'll let it pass through public void readFromStream(Reader r) throws IOException { BufferedReader br=new BufferedReader(r); lines=new ArrayList(); while(true) { String input=br.readLine(); if(input==null) break; lines.add(input); } } public void writeToStream(Writer w) throws IOException { PrintWriter pw=new PrintWriter(w); Iterator i=lines.iterator(); while(i.hasNext()) { pw.println((String)(i.next())); } } // returns the index of the largest element in the list static int findIdxBiggest(List l, int from, int to) { String biggest=(String) l.get(0); int idxBiggest=from; for(int i=from+1; i<=to; ++i) { if(biggest.compareTo(l.get(i))<0) {// it is bigger than biggest biggest=(String)l.get(i); idxBiggest=i; } } return idxBiggest; } // assumes i1, i2 are in range static void swap(List l, int i1, int i2) { Object tmp=l.get(i1); l.set(i1, l.get(i2)); l.set(i2, tmp); } public void sort() { for(int i=lines.size()-1; i>0; --i) { int big=findIdxBiggest(lines,0,i); swap(lines,i,big); } } /* void sort() { java.util.Collections.sort(lines); } */ public void sort(String inputFileName, String outputFileName) throws IOException{ Reader in=new FileReader(inputFileName); Writer out=new FileWriter(outputFileName); StringSorter ss=new StringSorter(); ss.readFromStream(in); ss.sort(); ss.writeToStream(out); in.close(); out.close(); } }
import java.io.IOException; import javax.swing.JOptionPane; StringSorterBadGUI.java public class StringSorterBadGUI { public static void main(String args[]) throws IOException { try { StringSorter ss=new StringSorter(); String inputFileName=JOptionPane.showInputDialog("Please enter input file name"); String outputFileName=JOptionPane.showInputDialog("Please enter output file name"); ss.sort(inputFileName, outputFileName); } finally { System.exit(1); } } }
StringSorterCommandLine.java
import java.io.IOException; public class StringSorterCommandLine { public static void main(String args[]) throws IOException { if(args.length!=2) { System.out.println("Usage: java Sort1 inputfile outputfile"); } else { StringSorter ss=new StringSorter(); ss.sort(args[0],args[1]); } } }
StringSorterGUI.java
import java.io.IOException; import javax.swing.*; import java.awt.event.*; import java.awt.Dimension; public class StringSorterGUI { private static class ComboFileChooser extends JPanel { private JTextField tf; ComboFileChooser(String label) { JLabel l=new JLabel(label); add(l); tf=new JTextField(20); add(tf); JButton b=new JButton("..."); b.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { JFileChooser chooser=new JFileChooser("."); int returnVal = chooser.showOpenDialog(null); if(returnVal == JFileChooser.APPROVE_OPTION) { try { tf.setText(chooser.getSelectedFile().getCanonicalPath()); } catch (IOException ioe) {} } } }); add(b); this.setVisible(true); } String getFileName() { return tf.getText(); } } private static class StringSorterDialog extends JFrame { private ComboFileChooser inFile, outFile; StringSorterDialog() { super("String Sorter"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); inFile=new ComboFileChooser("Input file:"); outFile=new ComboFileChooser("Output file:"); JButton sortBtn=new JButton("Sort"); sortBtn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { StringSorter ss=new StringSorter(); try { ss.sort(inFile.getFileName(), outFile.getFileName()); } catch (IOException ioe) { } } }); // use a up top to bottom layout getContentPane().setLayout(new BoxLayout(getContentPane(),BoxLayout.PAGE_AXIS)); getContentPane().add(Box.createRigidArea(new Dimension(10,10))); getContentPane().add(inFile); getContentPane().add(Box.createRigidArea(new Dimension(10,10))); getContentPane().add(outFile); getContentPane().add(Box.createRigidArea(new Dimension(10,10))); getContentPane().add(sortBtn); getContentPane().add(Box.createRigidArea(new Dimension(10,10))); pack(); } } public static void main(String args[]) throws IOException { StringSorter ss=new StringSorter(); StringSorterDialog d=new StringSorterDialog(); d.setVisible(true); } }
Test.java
import javax.swing.JOptionPane; public class Test { public static void main(String args[]) { String tt=JOptionPane.showInputDialog("Gimme some input"); System.exit(1); } }
TestStringSorter.java
import junit.framework.TestCase; import java.util.ArrayList; import java.io.*; public class TestStringSorter extends TestCase { private ArrayList make123() { ArrayList l = new ArrayList(); l.add("one"); l.add("two"); l.add("three"); return l; } public void testFindIdxBiggest() { StringSorter ss=new StringSorter(); ArrayList l = make123(); int i=StringSorter.findIdxBiggest(l,0,l.size()-1); assertEquals(i,1); } public void testSwap() { ArrayList l1= make123(); ArrayList l2=new ArrayList(); l2.add("one"); l2.add("three"); l2.add("two"); StringSorter.swap(l1,1,2); assertEquals(l1,l2); } public void testReadFromStream() throws IOException{ Reader in=new FileReader("in.txt"); StringSorter ss=new StringSorter(); ArrayList l= make123(); ss.readFromStream(in); assertEquals(l,ss.lines); } public void testSort1() { StringSorter ss= new StringSorter(); ss.lines=make123(); ArrayList l2=new ArrayList(); l2.add("one"); l2.add("three"); l2.add("two"); ss.sort(); assertEquals(l2,ss.lines); } public void testWriteToStream() throws IOException{ // write out a known value StringSorter ss1=new StringSorter(); ss1.lines=make123(); Writer out=new FileWriter("test.out"); ss1.writeToStream(out); out.close(); // then read it and compare Reader in=new FileReader("in.txt"); StringSorter ss2=new StringSorter(); ss2.readFromStream(in); assertEquals(ss1.lines,ss2.lines); } public void testSort2() throws IOException{ // write out a known value StringSorter ss1=new StringSorter(); ss1.sort("in.txt","test2.out"); ArrayList l=new ArrayList(); l.add("one"); l.add("three"); l.add("two"); // then read it and compare Reader in=new FileReader("test2.out"); StringSorter ss2=new StringSorter(); ss2.readFromStream(in); assertEquals(l,ss2.lines); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
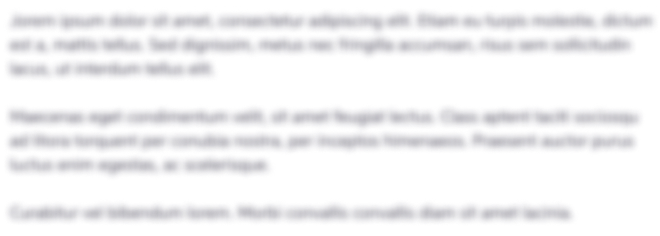
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started