Question
it is java code. if you work with eclipse. here is the need files: https://drive.google.com/open?id=1nN4n6179Zit2ENTefXJvGlZVKx2YQay9 https://drive.google.com/open?id=1BuwRem2aT7DOkbh7fco8HR8ThjfluZVc This is a puzzle that can always be solved.
it is java code.
if you work with eclipse. here is the need files: https://drive.google.com/open?id=1nN4n6179Zit2ENTefXJvGlZVKx2YQay9
https://drive.google.com/open?id=1BuwRem2aT7DOkbh7fco8HR8ThjfluZVc
This is a "puzzle" that can always be solved. Can you solve it five times in a row? If you can you probably developed an algorithm as to how to place the numbers.
The puzzle will present a series of three inequalities and a series of four random numbers. You solve the puzzle by entering the numbers in a row such that all of the inequalities are satisfied. For example, if the puzzle is:
Place the numbers in the boxes to solve the inequalities below (Enter to quit): [ ] < [ ] < [ ] > [ ] < [ ] 6 9 18 12 5 Enter the numbers on one line each separated by spaces:
The solution would be:
5 6 18 9 12
Since
5 < 6 < 18 > 9 < 12
// The commented code below asks the user for for a solution. // It is "interactive" with the user. // Scanner in = new Scanner(System.in); // genChallenge(); // displayChallenge(); // String line = in.nextLine(); // while (!line.equals("")) { // line = line.trim(); // remove spaces at beginning and end. // processInput(line); // if (userNumbers != null) { // if (testInput()) { // System.out.println("Congratulations! "); // genChallenge(); // displayChallenge(); // } // } // line = in.nextLine(); // } // in.close(); }
}
now, the user do not need to solve the puzzle by himself but require to write the following static void genSolution() { method to let the java app solve the puzzle. it mean that he need to fill out the code for the genSolution() method.
// Do NOT touch the code below until you get to the genSolution() method. import java.util.Scanner; import java.util.Arrays; import java.util.Random;
public class Main {
static int COUNT = 3; // # inequalities but there are COUNT+1 numbers. static final int MAX_NUMBER = 20; static char[] inequalities; // array of < and > characters. static int[] numbers; // array of randomly generated small integers. static int[] userNumbers; // these are the numbers your algorithm entered in genSolution() method. // Randomly generate the inequalities. static void genInequalities() { Random rand = new Random(); for (int i = 0; i < COUNT; i++) { inequalities[i] = rand.nextBoolean() ? '<' : '>'; } } // detect if num is duplicated in the numbers array. static boolean duplicate(int num, int index) { for (int i = 0; i < index; i++) { if (numbers[i] == num) { return true; } } return false; } // Randomly generate the numbers for the game. static void genNumbers() { numbers = null; numbers = new int[COUNT + 1]; int genCount = 0; Random rand = new Random(); while (genCount < COUNT + 1) { int randInt = rand.nextInt(MAX_NUMBER); if (!duplicate(randInt, genCount)) { numbers[genCount++] = randInt; } } } // Generate the challenge. static void genChallenge() { inequalities = new char[COUNT]; numbers = new int[COUNT + 1]; userNumbers = new int[COUNT + 1]; genInequalities(); genNumbers(); } // Display the challenge on the command line. static void displayChallenge() { System.out.printf("Place the numbers in the boxes to solve the inequalities below (Enter to quit): ", COUNT); for (int i = 0; i < COUNT; i++) { System.out.printf("[] %c ", inequalities[i]); } System.out.printf("[] "); for (int i = 0; i < COUNT + 1; i++) { System.out.printf("%2d ", numbers[i]); } System.out.printf(" "); System.out.println("Enter the numbers on one line each separated by a space:"); } // For interacting with the user. static void processInput(String line) { userNumbers = null; // regex here so multiple spaces can be entered. String [] tokens = line.split("[\\s]+"); int [] nums = new int [COUNT+1]; if (tokens.length != COUNT+1) { System.out.println("Wrong number of inputs. Try again."); return; } for (int i = 0; i < COUNT+1; i++) { try { int number = Integer.parseInt(tokens[i]); nums[i] = number; } catch (Exception e) { System.out.println("One of the numbers is not an integer. Try again."); return; } } int [] compare1 = new int[COUNT+1]; int [] compare2 = new int[COUNT+1]; System.arraycopy(numbers, 0, compare1, 0, numbers.length); System.arraycopy(nums , 0, compare2, 0, nums.length); Arrays.sort(compare1); Arrays.sort(compare2); for (int i = 0; i < numbers.length; i ++) { if (compare1[i] != compare2[i]) { System.out.println("There is a mismatch in your input. Try again."); return; } } // good user input userNumbers = new int [COUNT+1]; for (int i = 0; i < COUNT+1; i++) { userNumbers[i] = nums[i]; } } // See if there are any duplicate numbers in the user's solution. static boolean duplicateAnswer() { boolean duplicate = false; int[] testNumbers = Arrays.copyOf(userNumbers, userNumbers.length); Arrays.sort(testNumbers); for (int i = 0; i < testNumbers.length - 1; i++) { duplicate = testNumbers[i] == testNumbers[i + 1]; if (duplicate) { break; } } return duplicate; } // Test the user's numbers to see if they are a solution. static boolean testInput() { int i; boolean compare = false; for (i = 0; i < COUNT; i++) { System.out.printf("%2d %c ", userNumbers[i], inequalities[i]); compare = inequalities[i] == '<' ? userNumbers[i] < userNumbers[i + 1] : userNumbers[i] > userNumbers[i + 1]; if (!compare) { break; } } if (!compare) { System.out.printf("%2d ", userNumbers[i + 1]); System.out.println("Last inequality is not true. Try again."); } else { // print last number. System.out.printf("%2d ", userNumbers[i]); } if (duplicateAnswer()) { System.out.println("You used a number twice and that's not allowed. Try again."); compare = false; } return compare; } // Do NOT touch the code above. // Automatically generate a solution to the challenge. // Hints: // 1. You must sort the numbers array first. // 2. You must use the inequalities and userNumbers arrays. // 3. You need only one for loop with an if/else statement within it. static void genSolution() { // Your code goes here. } // The uncommented code tests your genSolution() code. public static void main(String[] args) { for (int i = 0; i < 10; i++) { genChallenge(); displayChallenge(); genSolution(); if (testInput()) { System.out.println("Congratulations! "); } else { System.out.println("Oh no! "); break; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
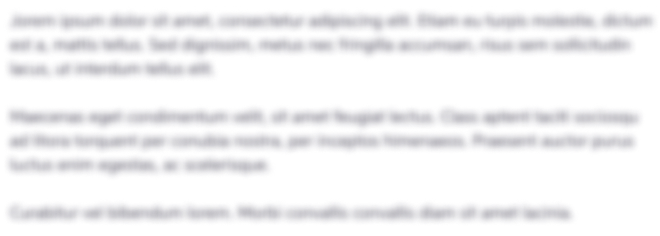
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started