Question
It turns out that Bowling uses a handicap system similar to golf. In both sports a handicap is used to allow players at different skill
It turns out that Bowling uses a handicap system similar to golf. In both sports a handicap is used to allow players at different skill levels to compete. The basic objective of Programming Project 3 is to create classes that enable you to calculate player's handicap. The games you will be working with are Golf and Bowling. Both games require adding scores and calculating handicaps. This functionality can be realized by implementing inheritance including an abstract class and polymorphism. In this project will be extending the Score and Golfer class we developed in Project 1 and adding classes required to calculate a Bowlers handicap. Below are the steps we will use to calculate handicaps in both games.
NOTE: Your code should ensure all instance field are valid as described in their requirements. If not write an error message to the standard output and continue. Set the field value to 9999. For example, the bowling score must be between 0 and 300(inclusive)(You may use a user defined exception but it isnt required for this project)
The Bowling handicap is calculated using the following steps:
Calculate the average of the bowlers last 5 scores.
Calculate the difference between the established base bowling average which in our case is 200 and the bowlers average.
Calculate the final handicap by taking 80% of the results of step 2.
Example
The bowlers last 5 scores average is 180.
200 - 180 = 20.
80% of 20 is 16.
The bowlers handicap is 16 so we would add 16 to his next score. Note if the bowler's average is higher than 200, there handicap would be subtract from their next score.
The Golf handicap index is calculated using the following steps:
Calculate the stroke differential of each the last 10 scores.
Stroke differential is calculated using the following formula:
Differential = (Score - course Rating) x (113 / Slope) : round to 2 decimal places.
Calculate the average of the 5 lowest differentials from step 1.
Calculate the final handicap by taking 96% of the results of step 2. round to 2 decimals places
Example
The golfers last 10 differentials are: 10.2, 11.5, 12.0, 9.8, 8.2, 9.9, 10.0 15.4, 12.3. 9.5.
The lowest 5 differentials are: 9.8, 8.2, 9.9, 10.0, 9.5
Average of 5 lowest differentials is: 47.4 / 5 = 9.48
96% of 9.48 is 9.1
The handicap index is 9.1. Note that like bowling a golfer handicap can be a negative number.
The Classes from Project 1 (Golfer and Score) will be reused with the following modifications:
Golfer Class - Must extend the Player abstract class (listed below)
Add a calculateHandicap method that returns a double representing the golfers current handicap. (Player's class abstract method)
Modify methods as required to accommodate the changes in the Score class.
Note: that Name and IDnum are stored in the Player class.
The toString methods return should be format similar to Project 1. However add the current handicap to the output. See Bowler class for an example.
An Array or ArrayList can be used. If an array is used array's size can be set to 20.
Score Class
Modify the course instance variable from a String to a Course object (see below) that contains the course name, course rating and course slope.
Remove the course rating and course slope instance fields.
Modify the rest of the methods and constructor to accommodate the change in the storage of course information.
Score is a gross score thus without any handicap added or subtracted.
The toString methods return should be format similar to Project 1.
The Course class
Instance Variables
courseName - a String representing the name of the course.
courseRating - a double representing the course rating, it must be between 60 and 80. (inclusive).
courseSlope - an int representing the course slope, it must be between 55 and 155 (inclusive).
Methods
Constructor - sets all instance fields from parameters
Default Constructor - sets all instance fields to a default value.
Access and mutator methods for all instance variables. Mutator method should be used to set all instance fields
toString - returns a nicely formatted String that contains its instance fields such as:
Bay Hill CC 69.5 123
The Bowler Class - Must extend the Player class listed below
Instance Variables
teamName - A String representing the bowler's team.
IDNum- A unique integer that identifies each bowler.
Array or ArrayList of BowlerScore objects - stores all the bowler's scores. The array's size can be set to 20.
Methods
Constructor - sets name and teamName from parameters and uses the static variable nextIDNum to retrieve the next available ID number. Creates Array.
Constructor - default constructor, sets all instance field to a default value. Creates Array.
A calculateHandicap method, that returns a double representing the golfers current handicap. (Player's class abstract method)
Access and mutator methods for all variables. NOTE Mutator method for IDNum should use the static variable nextIDNum. Mutator method should be used to set all instance fields
addScore - receives a BowlerScore object and adds it to the Array of BowlerScores.
toString - returns a nicely formated String that contains its instance fields:
John Smith ID number: 1234 Team Name: Waffle House Current Handicap: 16 Score Date Lane 139 6/3/14 Codova Lanes 145 7/23/14 Felton Lanes
The BowlerScore Class
Instance Variables
laneName - a String representing the name of the lane
score - an int representing a game's score, it must be between 0 and 300 (inclusive), The score is the gross score, without adding or substracting the handicap.
date - a String representing the date in format mm/dd/yy
Methods
Constructor - sets all instance fields from parameters
Default Constructor - sets all instance fields to a default value.
Access and mutator methods for all instance variables. Mutator method should be used to set all instance fields
toString - returns a nicely formated String that contains the score and its instance fields
139 6/3/12 Codova Lanes
The Player Class (abstract class)
Instance Variables
name - A String representing the player's name
IDNum- A unique integer that identifies each player
Static Variable - int nextIDNum - starts at 1000 and is used to generate the next Player's IDNum
Methods
Constructor - sets name and uses the static variable nextIDNum to retrieve the next available ID number.
Constructor - default constructor, sets all instance field to a default value.
Accessor and Mutator methods for instance variables.
toString - returns a neatly formated String representing the player's information and all their scores.
calculateHandicap - abstract method that returns a double representing the players handicap
The PlayerTester
This class should consist of a main method that tests all the methods in each class, either directly by calling the method from the Tester or indirectly by having another method call a method.
Players, Golf Course and Score information will be read from a file. A sample file is provided that shows, use this file format to aid in grading. Using file processing makes entering and testing program with data sets much more efficient.
The file created should include valid and invalid data that fully tests the application. Simple text file process is covered in slides named TextFileProcessing and TextFileExamples on elearning.
Included file called data.txt contains Player's data. Your project will be graded using a similar input file. Your project must run with an input file formatted as data.txt is formatted. Note the file format is as follows:
Number of scores
Character designation of type of player (G - golfer, B - bowler)
Name data etc
Score data
Create an Array of Players objects.
Populate the Array with both Golfer and Bowler objects.
After the ArrayList is filled, call the toString method on each object in the Array. Ensure each iteration calls the correct object.
Create UML Class Diagram for the final version of your project. The diagram should include:
All instance variables, including type and access specifier (+, -);
All methods, including parameter list, return type and access specifier (+, -);
Include Generalization and Aggregation where appropriate.
The PlayerTester does not need to be included.
Refer to the UML Distilled pdf on the content page as a reference for creating class diagrams.
A Link to a drawing program, Dia, is also posted on the content page.
Submission Requirements: Your project must be submitted using the instructions below. Any submissions that do not follow the stated requirements will not be graded.
1. You should have 10 files for this assignment:
Golfer.java - The Golfer class
Score.java - The Golfer's Score class
Course.java - The score's Course class
Bowler.java - The Bowler class
BowlerScore.java - The Bowler's Score class
Player.java - An abstract super class of players
PlayerTester.java A driver program for your project
data.txt - your test file
Simply UML Class diagram of your 5 classes, do not include the Tester. (Dia file or image file , jpg, gif, pdf etc)
The javadoc files for all the classes except the tester.(Do not turn in)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
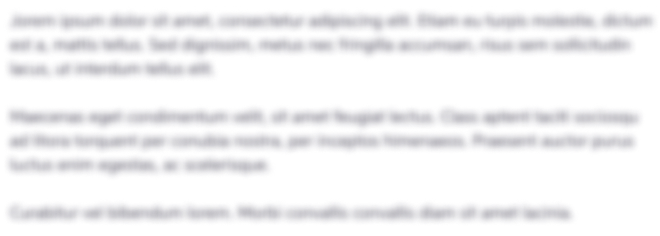
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started