Question
ITS BEEN HOURS SINCE IVE BEEN WORKING ON THIS ON VS CODE, NO ONLINE LIVE HELP, PLEASE HELP WITH CODE AND EXPLANATION Summary of Task
ITS BEEN HOURS SINCE IVE BEEN WORKING ON THIS ON VS CODE, NO ONLINE LIVE HELP, PLEASE HELP WITH CODE AND EXPLANATION Summary of Task 1: Set up the HTML and CSS. Summary of Task 2: Write the JavaScript for the left side of the game.Summary of Task 3: Write the JavaScript for the right side of the game.Summary of Task 4: Finish the game logic. ITS BEEN HOURS SINCE IVE BEEN WORKING ON THIS ON VS CODE, NO ONLINE LIVE HELP, PLEASE HELP WITH CODE AND EXPLANATION
TASK 1: Set up the HTML and CSS
THIS IS SMILE PNG FILE
For this task, you will create the HTML page without any JavaScript. The result will look like the below screenshot when viewed in a browser. As you can see, only the instructions and the middle line are visible.
Part 1: Set up the HTML
- Create a basic valid HTML5 document as you learned to do in Week 1, and give it the name matching-game.html. Be sure to set the DOCTYPE, the meta charset, and an appropriate title (such as 'Matching Game').
- In the body element, add an h1 element with the text content of Matching Game.
- Below this, add text with the instructions e.g. Click on the extra smiling face on the left. This could be containing any appropriate element such as p.
Part 2: Add left and right divs
- Below the text instructions, create two divs as follows:
The leftSide div will contain all the smiley faces shown on the left side, and the rightSide div will contain all the smiley faces shown on the right side.
Part 3: Add CSS
- Use an internal stylesheet to add the following styles.
- For all img elements:
position: absolute;
This is so that we can fix the exact position of any image later. Although we havent actually added any images to the webpage at this stage, we are adding this style rule now so that we can easily control their exact position later.
- For all div elements:
position: absolute; width: 500px; height: 500px;
This will set both the left and right divs to be 500px square each, with absolute positioning.
- For only the div with the id of rightSide (recall how to use an ID selector):
left: 500px; border-left: 1px solid;
This moves the rightSide div 500 pixels to the right, so that it is to the right of the leftSide div. Then it uses the border-left property to create a vertical line between the two divs.
- For all img elements:
At this point, you should be able to view your webpage in your browser and see that it looks like the screenshot shown above.
TASK 2: Generate the left side images
For this task, you need to add JavaScript code which generates smiley face images on the left side only. The result will look like this when viewed in a browser:
You can download the smile.png file here:
smile.png
Add it to your images folder.
Part 1: Global variables
- Create a script element just before the closing tag.
- We will make careful use of two global variables.
- Using the let keyword, declare a variable named numberOfFaces at the top of your script element. Initialize it to 5.
- Using the const keyword, declare a variable named theLeftSide. Use either querySelector or getElementById to point it to the left side div.
Part 2: Create the generateFaces() function
- Declare a function named generateFaces with an empty parameter list.
- In this function, the faces are generated using a for a loop. Set up a for loop to iterate numberOfFaces times. In each iteration:
- Declare a variable named face and for its value, create an img node using document.createElement() with the appropriate argument. At the end of this operation, the variable face should point to a newly created img node that has not yet been attached to the DOM.
- Set the image's src property as follows, making sure that the smile.png file is truly in your images folder (download link is just below the screenshot above):
face.src = 'images/smile.png';
- The position of the face node is controlled by the top and left style properties. You will next generate random numbers to use as the values for these properties.
- Declare a variable named randomTop. Use Math.floor() and Math.random() to generate a random number between 1-400, and set this number as the value of randomTop.
- Declare another variable named randomLeft. Again, generate a random number in the 1-400 range and set it as the value of randomLeft.
- Set the value of face.style.top to randomTop, making sure to add 'px' to the end, e.g.:
face.style.top = randomTop + 'px';
-
- Similarly, set the value of face.style.left to randomLeft, making sure to add 'px'.
- Remember in Part 1, you created a global variable named theLeftSide. Use appendChild() to add the newly created face image element node as a child of theLeftSide.
- The first time that the generateFaces function is run, the for loop inside it should iterate 5 times, causing 5 smiley face images to appear at random locations on the left side.
Part 3: Load the Function
- To call the generateFaces function when the webpage is loaded, use or the other of the below options, not both.
- Add an inline onload event handler to the body element:
- Alternatively, you can add the following code at the top of your script element, globally and not inside any functions, to set up an addEventListener on the window object (the load event has inconsistent results used on the body, so the window object must be used instead)
window.addEventListener('load', generateFaces);
At this point, you should be able to view your webpage in your browser and see that it looks like the screenshot shown above for Task 2. TASK 3: Handle the right side
You will now extend the JavaScript you developed in Task 2 to generate images on the right side. At the end of this task, your assignment should look similar to this in the browser, with smiley faces on both sides, one less on the right side:
Part 1: Global variable
- Declare a new global variable named theRightSide, below where you declared the variable theLeftSide. Point this variable to the div with the ID of rightSide.
Part 2: Clone images to the right side
- Add the following code in the generateFaces function, below the for loop you created in Task 2:
-
Declare a variable called leftSideImages or similar. For its value, clone the entire theLeftSide div node, including all its children, i.e.:
-
const leftSideImages = theLeftSide.cloneNode(true);
-
- After this, use removeChild() to remove the last child of leftSideImages. This will ensure that there is one less smiley face on the right side.
- Use appendChild() to append leftSideImages as a child of theRightSide.
- At this point, the DOM will look like this:
- It is totally fine to have a div under a div. In fact, this is quite common.
At this point, you should be able to view your webpage in your browser and see that it looks like the screenshot shown above for Task 3. However, nothing will happen yet if you click on the faces.
TASK 4: Finish the game logic
In this task, you will complete the JavaScript code developed in Tasks 2 and 3 to include adding and removing event handlers and other game logic.
Part 1: Add an event handler function to the extra face using addEventListener
The player is supposed to click on the extra smiley face on the left side that is not on the right side. Remember, the faces on the right side are a clone of the left side, except for the last child which was been removed on the theRightSide node.
- That means the extra smiley face on the left side will be the last child of the theLeftSide node. You will need to add an event handler to this face so that when it is clicked, the program will go to the next level.
- Inside the generateFaces function, below the existing code, set up an event listener for the last child of theLeftSide with addEventListener.
- Have addEventListener() listen for the event 'click' as the first argument. For the second argument, use the function name nextLevel (or something similar).
- Outside of the function generateFaces, declare a new function named nextLevel (or whatever name you used for the second argument in the event listener you just created.)
- The starting code for this function is provided below:
function nextLevel() { event.stopPropagation(); numberOfFaces += 5; generateFaces(); }
- The line
event.stopPropagation();
is necessary in order to ensure that the event does not also get applied to other elements in the web page, such as other faces. That would trigger the function multiple times, which is not what we want.
- The line
numberOfFaces += 5;
increases the number stored in numberOfFaces by 5, so that the next time the faces are generated there are 5 more than before on both sides
- The line
generateFaces();
means that a new set of faces is generated. Because of the increase in value of numberOfFaces, there will be 5 more faces than before on both sides.
Part 2: Add an event handler function to the body using addEventListener()
You need to add another function for handling the situation when the player clicks on anything except the correct face.
- In the generateFaces function, below where you added the last event listener, use addEventListener() one more time. You will use it on document.body with the first argument 'click' and the second argument of gameOver.
- Outside of the generateFaces function, declare a third function named gameOver with an empty parameter list.
- Inside this function, write an alert() with the message of 'Game Over!'.
Part 3: Remove event handler functions using removeEventListener()
- Inside the gameOver function, use removeEventListener twice to remove the event handlers you created with addEventListener. That means you need to use it on document.body to remove the gameOver event handler function for the 'click' event, and on theLeftSide.lastChild to remove the nextLevel event handler function for the 'click' event.
Part 4: Delete the child nodes
Each time the player clicks on the correct face, all faces must be removed before a new set of faces is generated and added to the page. So that means at the appropriate place, all children of both theLeftSide and theRightSide div nodes need to be removed. In the past week, you learned how to remove all child nodes of a parent node using a while loop. Use that knowledge to add two while loops to the nextLevel function to remove all child nodes of theLeftSide and theRightSide. Be sure to place these loops before the call to generateFaces() in the nextLevel function. MY REPLY TO "ANONYMOUS"-- WHAT QUESTION?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
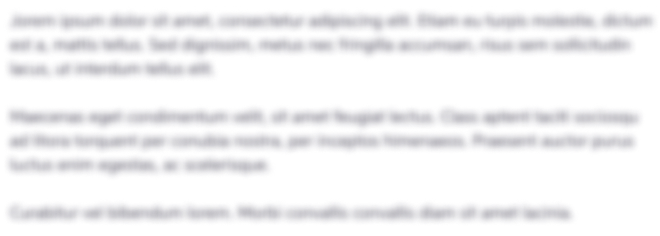
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started