Question
It's my java lab. Please help me to do.. import java.util.Random; public class Die { private int roll; private int numSides; /** * Default constructor
It's my java lab. Please help me to do..
import java.util.Random; public class Die { private int roll; private int numSides; /** * Default constructor for a Die * Initializes roll to 0 * and numSides to the standard 6 */ public Die() { } /** * Constructor for a Die * Initializes roll to 0 * and numSides to specified * number of sides * @param numSides the * number of sides to the die */ public Die(int numSides) { } /** * Assigns roll to a random value * from 1 to numSides */ public void makeRoll() { } /** * Returns the roll number * @return the number generated * when die is rolled */ public int getRoll() {
return -1;
} }
public class Dice { private Die die1; private Die die2; /** * Default constructor * sets both dice to * be 6 sided */ public Dice() { } /** * Constructor to set * both dice to have the * same number of sides * @param sides the number * of sides of both dice */ public Dice(int sides) { } /** * Rolls both dice */ public void roll() { } /** * Returns the roll value * of Die1 * @return the roll value * of Die1 */ public int getDie1Roll() {
return -1;
} /** * Returns the roll value * of Die2 * @return the roll value * of Die2 */ public int getDie2Roll() {
return -1;
} /** * Returns the sum of the * roll values of Die1 * and Die2 * @return the sum of the rolls */ public int getSumRolls() {
return -1;
} }
Player Class Specifications
Write a class to represent one player of the game, whether it be the user of our program or the computer A.I.
Each player rolls his or her own pair of dice and has a current score.
Implement the constructors and other methods as follows.
Note: You are not permitted to add any additional methods or otherwise alter the class except to implement the given methods.
public class Player { private Dice dice; private int score; /** * Player constructor that * sets the number of sides * of the dice the player will * roll and gives the player * a default score of 0 * @param sides */ public Player(int diceSides) { } /** * Rolls the two player dice */ public void rollDice() { } /** * Prints the value of the the rolls * of the two dice to the console, with * each roll separated by a blank space */ public void printRoll() { } /** * Prints out whether a special roll was made * and updates the score accordingly * For snake eyes (two 1s) prints Snake Eyes! * and updates the score by 5 * When the two die rolls match otherwise, * prints out Matching! and updates the * score by 3 * For total of 3, prints out Total of 3! * and updates the score by 1 * For total of 5, prints out Total of 5! * and updates the score by 2 * For total of 7, prints out Oh no! Total of 7! * and resets the score back to 0 * For total of 9, prints out Total of 9! * and updates the score by 3 * For total of 11, prints out Total of 11! * and updates the score by 4 * For any other odd number, prints out Odd Roll! * and updates the score by 2 */ public void updateScore() { } /** * Returns the player's current score * @return the current score */ public int getScore() { return -1; } }
BattleDieGalactica Class Specifications
Write a class to represent one game of Battle Die Galactica
Implement the below methods, while leaving the constructor to be the default provided by the compiler
Note: You *are* permitted to add any additional methods in addition to writing the provided methods
This class must work identically to what is shown in the sample output.
Note that the user should be able to end the game early at any time by typing n in response to the question Roll again? (y/n):
As well as the displaying the game play as the game progresses, it must determine who won (player or computer) or whether the game was a tie, and print a game ending summary.
It must display this information as shown in the sample output below.
import java.util.Scanner; public class BattleDieGalactica { private Player player; private Player ai; /** * Prompts the user to input a number of die sides * Prints out the user choice and * Constructs a new player and ai based on the number * of sides of the dice * Note that the user input will be rounded up to 6, 8, 10 * or 12 and also rounded down to 12 if a higher number is * input. * @param input the Scanner */ private void gameSetUp(Scanner input) { System.out.print("Enter the number of sides (6, 8, 10 or 12): "); } /** * Prints out the current score for each opponent * to the console in the form: * Player: * Computer: * Note: no <> should be displayed */ public void printScore() { } /** * Determines whether any player has reached * 21 points, thus ending the game * @return whether the game is over */ private boolean gameOver() { return false; } public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("***Welcome to Battle Die Galactica!*** "); BattleDieGalactica game = new BattleDieGalactica(); input.close(); } }
Optional Test Class
There are a lot of components involved in this assignment.
To make sure that each piece is working properly, I recommend that you create an optional GameTest.java class where you call the different methods you write as you go along to see that they are working properly.
For example:
public class GameTest { public static void main(String[] args) { System.out.println("***Testing Die Class***"); Die d = new Die(6); d.makeRoll(); System.out.println("Die roll: " + d.getRoll()); System.out.println(" ***Testing Dice Class***"); Dice dice = new Dice(8); dice.roll(); System.out.println("Die 1 roll: " + dice.getDie1Roll()); System.out.println("Die 2 roll: " + dice.getDie2Roll()); System.out.println(" ***Testing Player Class***"); Player player = new Player(6); player.rollDice(); player.printRoll(); System.out.println("Score: " + player.getScore()); //add more method calls where needed } }
Sample Output:
***Welcome to Battle Die Galactica!*** Enter the number of sides (6, 8, 10 or 12): 7 8-sided dice! Roll the dice? (y/n): y Your Roll: 2 5 Oh no! Total of 7! Current scores: Player: 0 Computer: 0 Counter Roll: 1 2 Total of 3! Current scores: Player: 0 Computer: 1 Roll again? (y/n): y Your Roll: 3 3 Matching! Current scores: Player: 3 Computer: 1 Counter Roll: 1 2 Total of 3! Current scores: Player: 3 Computer: 2 Roll again? (y/n): y Your Roll: 5 7 Current scores: Player: 3 Computer: 2 Counter Roll: 7 2 Total of 9! Current scores: Player: 3 Computer: 5 Roll again? (y/n): y Your Roll: 5 6 Total of 11! Current scores: Player: 7 Computer: 5 Counter Roll: 6 2 Current scores: Player: 7 Computer: 5 Roll again? (y/n): y Your Roll: 5 4 Total of 9! Current scores: Player: 10 Computer: 5 Counter Roll: 6 6 Matching! Current scores: Player: 10 Computer: 8 Roll again? (y/n): n Game summary: Player: 10 Computer: 8 Congratulations! You win!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
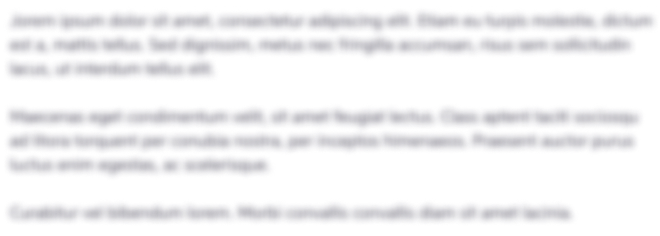
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started