Question
I've complete most of the code but am receiving the following error when I compile the code: Possible lossy conversion from int to byte. This
I've complete most of the code but am receiving the following error when I compile the code: "Possible lossy conversion from int to byte." This is where the error in my code is occurring:
while (endOfFile = false) { try { byte input = inputFile.readByte(); input= input + 10; outputFile.writeByte(input); } catch(EOFException e) { endOfFile = true; } }
Module 8.3 | Challenge
The Cryptography Class
Overview
In this two-part challenge, you will create a Cryptography class with a public static encodeFile method and a public static decodeFile method. These methods encode and decode the contents of a file, respectively. Each method has an input file and an output file as parameters. Two testing programs will also be created.
For an overview on cryptography, watch this video presentation:
https://www.khanacademy.org/computing/computer-science/cryptography/crypt/v/intro-to- cryptography
Specifications
Part 1
MyMessage.txt
Create a text file named MyMessage.txt and enter these letters: abcdefg Note: The encrypted letters will be the letters: klmnopq
MyMessage.txt will be encrypted by method encodeFile producing a secret code that will be stored in an output file EncryptedMessage.txt.
Save MyMessage.txt in the same folder as the folder that will contain your Cryptography class and the test harness file.
Cryptography.java
Create the skeleton of a class called Cryptography.
Create a public static method encodeFile.
Add the throws clause to the method header for the IOException type.
FileInputStream and DataInputStream classes (java.io package) will be used to
read from the input file (MyMessage.txt).
A boolean variable endOfFile should be declared and initialized to false.
Declare and create new FileInputStream, DataInputStream, FileOutputSteream,
and DataOutputStream objects.
Open the files.
Process the files using the readByte and writeByte methods. Here is an algorithm:
while not end of file: try
read the next byte from the input file add 10 (which produces the new encoded character) write the byte to the output file
catch using EOFException set the endOfFile flag to true
Close the files.
CryptographyTest1.java
Create the skeleton of a testing program CryptographyTest1.
Display a message to explain what will happen when the program executes: Encrypting the contents of file MyMessage.txt. The encoded version will be stored in the file EncryptedMessage.txt.
Use try and catch blocks o CalltheencodeFilemethodwithMyMessage.txtand
EncryptedMessage.txt arguments. o DisplayamessageinformingtheusertoopenEncryptedMessage.txtinNotepad
to see the results.
o An error message should display if an IOException occurs.
Sample Output for Encoding a File
MyMessage.txt file contents
In this run, the MyMessage.txt was not found.
Encrypting the contents of the file MyMessage.txt. The encoded version will be stored in the file EncryptedMessage.txt.
Error - MyMessage.txt (The system cannot find the file specified)
This is the output from a correct program run.
Encrypting the contents of the file MyMessage.txt. The encoded version will be stored in the file EncryptedMessage.txt. Done. Use Notepad to inspect the encrypted file.
EncryptedMessage.txt file contents
Part 2
Cryptography.java
Now lets reverse the process.
Add a method decodeFile to the Cryptography class. This method will read an encrypted
file and decode it to produce the original message. For example, the EncryptedMessage.txt file with contents klmnopqr, will be saved to a new file RecoveredMessage.txt, which will contain the contents abcdefgh.
Hint: Copy your method encodeFile and modify to create the decodeFile method. CryptographyTest2.java
Create a second testing program CryptographyTest2.
Display a message to explain what will happen when the program executes: Decrypting the contents of the file EncryptedMessage.txt The decrypted file will be stored as RecoveredMessage.txt.
Include try and catch blocks. o CallthedecodeFilemethodwithEncryptedMessage.txtand
RecoveredMessage.txt arguments. o DisplayamessageinformingtheusertoopenRecoveredMessage.txtinNotepad
to see the results.
o An error message should display if an IOException occurs.
Sample Output for Decoding a File
EncryptedMessage.txt file contents
In this run, the EncryptedMessage.txt was not found.
Decrypting the contents of the file EncryptedMessage.txt. The decrypted file will be stored as RecoveredMessage.txt.
Error - EncryptedMessage.txt (The system cannot find the file specified)
This is the output from a correct program run.
Decrypting the contents of the file EncryptedMessage.txt. The decrypted file will be stored as RecoveredMessage.txt.
Done. Use Notepad to inspect the decrypted file.
RecoveredMessage.txt file contents
Additional Requirements
Follow the Basic Coding Standards.
Include a comment section at the top of your program with your student ID, name, and title of
the program.
Grading Rubric
2 points | Your programs compile and execute. |
3 points | Part 1: You have created the input file and variables specified. |
4 points | Part 1: You have correctly coded the processing algorithm. |
2 points | Part 1: Your output resembles the sample output. |
4 points | Part 2: Youve coded a method decodeFile. |
2 points | Part 2: Youve create a testing program with exception handling. |
2 points | Part 2: Your output resembles the sample output. |
1 point | Your code follows the Basic Coding Standards. |
Total: 20 points
Adapted from Deitels How to Program, 10e @2017
Step by Step Solution
There are 3 Steps involved in it
Step: 1
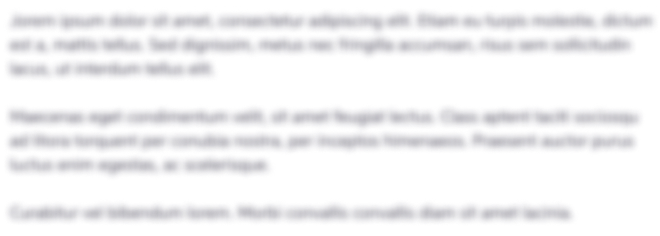
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started