Question
I've posted my class and driver. My output is not looking like the output provided. I've highlighted the parts(in the output section) that look different
I've posted my class and driver. My output is not looking like the output provided. I've highlighted the parts(in the output section) that look different from mine? I know it's affected by my IsEven and leapYear implementation. Since those are boolean values, is there another way to implement their output other than true and false? I was told I should always have a toString method in my class. Do I need one if my driver already has a print method? Thank you.
Write a class definition (not a program, there is no main method) named Geek(saved in a file Geek.java) that models a person who is a geek. For our purposes, a geek is someone who delights in answering all sorts of questions, such as is this string a palindrome?, what is the sum of all numbers between two numbers? among other things. A Geek has a name and also keeps track of how many questions s/he has answered. Your Geek class must have:
Only two instance variables - the Geeks name and number of questions asked so far.
public Geek(String name, int numQuestions)- Constructor - sets theGeeks name and the number of questions asked.
public String getName()- Takes no parameters and returns the Geeks name as a String (dont count this request in the total questions).
public int getNumberOfQuestions()- Takes no parameters and returns as an int how many questions has been asked (dont count this request in the total).
public boolean isEven(int num1, int num2)- It takes two integers and returns a boolean valueindicating if the sum of the numbers is even or not (this counts as the Geek being asked one more question, so update the appropriate instance variable).
public int sum(int num1, int num2)- Takes two integers and returns an int which is the sum of all numbers between the two inclusive (include the numbers in the sum) - for full credit this method should work even if the two numbers are the same (the sum is just one of the numbers) or if the first number is larger than the second. Also, you cannot assume which number will be greater.
public boolean leapYear(int year)- It takes an integer and returns a boolean value indicating if the year is a leap year. A leap year is one with 366 days. A year is a leap year if:
it is divisible by 4 (for example, 1980),
except that it is not a leap year if it is divisible by 100 (for example 1900);
however, it is a leap year if it is divisible by 400 (for example, 2000).
There were no exceptions before the introduction of the Gregorian calendar on October 15, 1582 (for example, 1500 was a leap year). You may NOT use Javas GregorianCalendar class. (This counts as the Geek being asked one more question, so update the appropriate instance variable). Save the Geek class in a file called Geek.java. Write a test driver called Assignment5.java with the main method to create a new Geek object and to test the methods in the class Geek. A sample output is shown below. Assignment5.java will provide the following menu to the user. Based on the users choice, the program needs to perform corresponding operation. This will be done by calling (invoking) one of the methods you defined in the Geek class. The program will terminate when the user enters q.
Command Options
-----------------------------------
a : get name
b: number of questions asked
c: sum is even
d: sum between two integers
e: leap year
?: display the menu again
q: quit this program
Here is the description for each option:
a: asks for the Geeks name
b: returns the number of questions asked so far
c: asks for two integers and prints if the sum of the numbers is even
d: asks for two integers and returns the sum of all numbers between them, inclusive
e: asks for an integer and returns if it is a leap year or not
?: displays the menu
q: quits
Helpful Hints
Work on it in steps - write one method, test it with a test driver and make sure it works before going
on to the next method.
Always make sure your code compiles before you add another method.
Your methods should be able to be called in any order.
Sample Output
(user input is in bold)
Command Options
a : get name
b: number of questions asked
c: sum is even
d: sum between two integers
e: leap year
?: display the menu again
q: quit this program
Please enter a command or type?
a
Name: Geek
Please enter a command or type?
b
Number of questions: 0
Please enter a command or type?
c
Enter a number: 3
Enter the second number: 3
The sum of the numbers is even
Please enter a command or type?
c
Enter a number: 3
Enter the second number: 4
The sum of the numbers is odd
Please enter a command or type?
b
Number of questions: 2
Please enter a command or type?
d
Enter a number: 1
Enter a second number: 10
The sum between 1 and 10 is 55
Please enter a command or type?
d
Enter the first number: 7
Enter the second number: 4
The sum between 4 and 7 is 22
Please enter a command or type?
b
Number of questions: 4
Please enter a command or type?
e
Enter a year: 1900
1900 is not a leap year
Please enter a command or type?
e
Enter a year: 1996
1996 is a leap year.
3
Please enter a command or type?
e
Enter a year: 2000
2000 is a leap year
Please enter a command or type?
b
Number of questions: 7
Please enter a command or type?
q
Press any key to continue . .
GEEK CLASS
public class Geek { // instance variables go below here private String geekName; private int numOfQuestions; //Constructor public Geek(String name, int numQuestions) { geekName = name; numOfQuestions = numQuestions; }
//accessor public String getName() { return geekName; } public int getNumberOfQuestions() { return numOfQuestions; } //returns if sum of numbers is even public boolean isEven(int num1, int num2) { numOfQuestions++; if((num1 + num2)%2 == 0) { return true; } else { return false; } } //sum of all numbers between two inclusive integers public int sum(int num1, int num2) { numOfQuestions++; int sum = 0; int max, min; if(num1==num2) { return num1; } if(num1 > num2) { max = num1; min = num2; } else { max = num2; min = num1; } for (int i=min; i<=max; i++) { sum += i; } return sum; } //indicating leap year public boolean leapYear(int year) { numOfQuestions++; if((year%4 == 0 && year%100 != 0) || (year%400 ==0)) { return true; } else { return false; } }
}
DRIVER
import java.util.*;
public class Assignment5 {
public static void main (String[] args) {
Scanner console = new Scanner (System.in);
String choice; char command; int num1, num2, year;
// print the menu printMenu(); // create new Geek object Geek myGeek = new Geek("Geek", 0);
do { // ask a user to choose a command System.out.println(" Please enter a command or type ?"); choice = console.next().toLowerCase(); command = choice.charAt(0);
switch (command) { case 'a': System.out.println("Geek Name: " + myGeek.getName());//prints the Geek's name break; case 'b': // System.out.println("Number of questions: " + myGeek.getNumberOfQuestions()); break; case 'c': // System.out.println("Enter the first number: "); num1 = console.nextInt();//asks for two integers and finds and prints if their sum is even or odd System.out.println("Enter the second number: "); num2 = console.nextInt();//asks for two integers and finds and prints if their sum is even or odd System.out.println("The sum of the numbers " + myGeek.isEven(num1, num2)); break; case 'd': // System.out.println("Enter the first number: "); num1 = console.nextInt(); System.out.println("Enter the second number: "); num2 = console.nextInt(); System.out.println("The sum between " + num1 + " and " + num2 + " is " + myGeek.sum(num1, num2));//asks for two integers and finds and prints the sum of all integers between them (inclusive) break; case 'e': // System.out.println("Enter a year: ");//asks for a year and finds out and prints if is leap year or not year = console.nextInt(); System.out.println(year + "is" + myGeek.leapYear(year)); break; case '?': printMenu(); break; case 'q': break;
default: System.out.println("Invalid input");
}
} while (command != 'q');
console.close(); } //end of the main method
public static void printMenu() { System.out.print(" Command Options " + "----------------------------------- " + "a: get name " + "b: number of questions asked " + "c: sum is even " + "d: sum between two integers " + "e: leap year " + "?: display the menu again " + "q: quit this program ");
} // end of the printMenu method
} // end Assignment5 class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
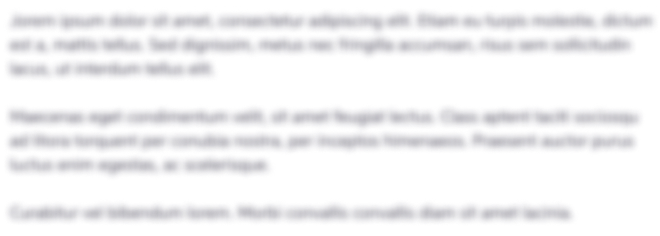
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started