Question
JAVA: 1. SUBMITTING NEW TRANSACTIONS Since the job of this program is to store and audit transactions, lets discuss how those transactions will be submitted
JAVA: 1. SUBMITTING NEW TRANSACTIONS
Since the job of this program is to store and audit transactions, lets discuss how those transactions will be submitted and stored within our program. Groups of transactions will be submitted together, and each of these groups will be represented by a perfectly sized array of primitive integers (see zybooks 1.4-1.7 for more on perfect size vs oversize arrays). Well see later that the encoding of these integers may vary from one group of transactions to the next, and so keeping track of each group of transactions as distinct from the others will be important. The collection of these groups of transactions will be represented by an oversize array of transaction groups (or of perfect size integer arrays).
The first method that we will implement adds a perfectly sized array of transactions into an oversize array of perfect size integer arrays. The signature and behavior of this method must exactly match the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | /** * Adds a transaction group to an array of transaction groups. If the allTransactions array is * already full then this method will do nothing other than return allTransactionCount. * * @param newTransactions is the new transaction group being added (perfect size). * @param allTransactions is the collection that newTransactions is being added to (oversize). * @param allTransactionsCount is the number of transaction groups within allTransactions * (before newTransactions is added. * @return the number of transaction groups within allTransactions after newTransactions is added. */ public static int submitTransactions(int[] newTransactions, int[][] allTransactions, int allTransactionsCount) {
return -1; } |
After implementing this method, you should create a file with an empty class definition named AuditableBankingTests. You can then submit these two files to zybooks and ensure that they are passing the first two tests. If not, correct any defects that they report before moving on.
2. TRANSACTION ENCODING AND PROCESSING COMMANDS
As mentioned in the previous step, different transaction groups may be encoded within an integer array in different ways. Here are descriptions of the three kinds of encoding that our program must support.
Binary Amount Transactions: When the first number (at index zero) within a transaction group array is zero, the rest of the numbers in that array represent transactions encoded as Binary Amount Transactions. Each binary amount transaction is either a zero or a one. Ones represent deposits of $1, and zeros represent withdraws of $1. Any other numbers found in such transaction arrays represent errors, but we will not test how your code responds to such errors, so you can ignore them.
Integer Amount Transactions: When the first number (at index zero) within a transaction group array is one, the rest of the numbers in that array represent transactions encoded as Integer Amount Transactions. Integer amount transactions can be either positive or negative integers. Positive integers represent deposits of the specified integer amount, and negative integers represent withdraws of the specified amount. Any zeros found within such transactions represent errors, but we will not test how your code responds to such errors, so you can ignore them.
Quick Withdraw Transactions: When the first number (at index zero) within a transaction group is two, the rest of the numbers in that array represent transactions encoded as Quick Withdraw Transactions. The non-negative integer numbers within quick withdraw transaction arrays represent the numbers of withdraws, rather than the amounts of withdraws (as was the case with the previous encoding). The amounts for quick withdraws are fixed at $20, $40, $80, and $100. And the number of withdraws of each amount is stored at indexes one, two, three, and four respectively. This means that the size of every quick withdraw array should be five. Arrays of other sizes, and negative withdraw amounts both represent errors, but we will not test how your code responds to such errors, so you can ignore them.
For the purpose of this program, the user will enter each group of transactions as a string of integers separated by spaces, which conforms to one of these three encoding standards. For example, they might enter this string containing a binary amount transaction:
0 1 0 0 1 1 1 0 1
Define a new method called processCommand that takes such a string as command/input, and correctly adds a new transaction group to the provided set of transactions groups. When the first character within a command does not correspond to a valid transaction encoding or the allTransactions array starts out full, this method should not make any changes to allTransactions. Note in the required method signature below, that this method returns an int (as is required to keep track of the potentially growing size of our oversize array):
1 2 3 4 | public static int processCommand(String command, int[][] allTransactions, int allTransactionsCount) { // TODO: Implement this method return -1; } |
The following methods from the Java8 API may be helpful in implementing this processCommand method:
Integer.parseInt(string) returns an integer encoding of the number represented by the string input.
string.trim() returns a new copy of the string this method is called on, but without any leading or trailing whitespace.
string.split( ) breaks the string this method is called on into parts around each space, and returns all of those parts as a single array of strings.
Since it is currently difficult to tell whether this method is working, we are going to write a test method within AuditableBankingTests to help us verify this. Add a method to this class with the following signature:
1 2 3 4 | public static boolean testProcessCommand() { // TODO: Implement this method return false; } |
This test method should test AuditablBankingTests.processCommand by passing in some hard-coded arguments (a command like 0 0 1 1 0 1 1 into an oversize array containing two prior transaction groups) and checking whether the return value and contents of allTransactions match the specified behavior (like allTransactions[2][2] == 1). Try checking for at least two very specific defects within in this test, and keep this test code as simple as possible to avoid the likelihood of confounding bugs within your tests. This method should print out feedback about any defects that it finds. In addition to this feedback for human eyes, this method should return false when any defects are found and true when none are found. Create a main method within your AuditableBankingTests class to run this test (and additional tests that you add in the future).
3. AUDITING TRANSACTIONS
Next, well implement a method to calculate and return the total account balance based on a collection of provided transaction groups. Here is the required signature for this method (to be implemented within AuditableBanking.java), along with the signature of a corresponding test method (to be implemented within AuditableBankingTests.java).
1 2 3 4 | public static int calculateCurrentBalance(int[][] allTransactions, int allTransactionsCount) { // TODO: Implement this method return -1; } |
1 2 3 4 | public static boolean testCalculateCurrentBalance() { // TODO: Implement this method return false; } |
Another form of auditing that we would like to perform on collections of transaction groups is to count the number of overdrafts. An overdraft is when a withdraw is made that results in a negative balance. To detect overdrafts, youll need to process collections of transaction groups in order of increasing indexes starting at zero. Within each group of transactions, you should processes transactions in order of increasing indexes too. Here is the signature of the method that you must implement, along with some test code to add to your AuditableBankingTests class. These tests should help you verify your own understanding of the required methods behavior, and should also help you begin to test your implementation of it.
1 2 3 4 | public static int calculateNumberOfOverdrafts(int[][] allTransactions, int allTransactionsCount) { // TODO: Implement this method return -1; } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | public static boolean testCalculateNumberOfOverdrafts() { boolean foundProblem = false; int[][] transactions = new int[][] { {1,10,-20,+30,-20,-20}, // +2 overdrafts (ending balance: -20) {0,1,1,1,0,0,1,1,1,1}, // +2 overdrafts (ending balance: -15) {1,115}, // +0 overdrafts (ending balance: +100) {2,3,1,0,1}, // +1 overdrafts (ending balance: -100) };
// test with a single transaction of the Integer Amount encoding int transactionCount = 1; int overdrafts = AuditableBanking.calculateNumberOfOverdrafts(transactions,transactionCount); if(overdrafts != 2) { System.out.println("FAILURE: calculateNumberOfOverdrafts did not return 2 when transactionCount = 1, and transactions contained: "+Arrays.deepToString(transactions)); foundProblem = true; } else System.out.println("PASSED TEST 1/2 of TestCalculateNumberOfOverdrafts!!!");
// test with four transactions: including one of each encoding transactionCount = 4; overdrafts = AuditableBanking.calculateNumberOfOverdrafts(transactions,transactionCount); if(overdrafts != 5) { System.out.println("FAILURE: calculateNumberOfOverdrafts did not return 5 when transactionCount = 4, and transactions contained: "+Arrays.deepToString(transactions)); foundProblem = true; } else System.out.println("PASSED TESTS 2/2 of TestCalculateNumberOfOverdrafts!!!");
return !foundProblem; } |
After you get these two methods working revisit your definition of the processCommand method. Update this method to calculate and print out the balance when the first non-white-space character within the command string is a B (upper or lower case), and to calculate and print out the number of overdrafts when the first non-white-space character within the command string is an O (upper or lower case). The following methods from the Java8 API may be helpful in updating the processCommand method in this way:
string.charAt(index) returns the character at the specified zero-based index from within this string.
string.toUpperCase() returns a new copy of the string this method is called on, but with an upper case version of each alphabetic character.
4. THE DRIVER APPLICATION
The last step of this assignment is to implement a nice text-based interface to access and drive the program. Organize this functionality into whatever custom static methods you see fit, but ensure that running the main method within your AuditableBanking class results in an interaction logs comparable to the sample shown below. All of your variables should be local to some static method (no class or instance fields are allowed), unless they are constants marked with the keyword final. Pay close attention to the details within the example log below, to ensure that your programs welcome message, thank you message, command prompts, current balance, and overdraft numbers are all printed in the same manner. You do not need to worry about erroneous input from the user, because all of our grading tests will focus on properly encoded commands, as described within this specification. The user should be able to submit at least 100 transaction groups. Note that the users input below is shown in red, and that the rest of the text below was printed out by the program.
========== Welcome to the Auditable Banking App ========== COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: 0 1 1 0 0 0 1 1 COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: 1 -10 +100 COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: 2 2 1 0 0 COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: B Current Balance: 11 COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: o Number of Overdrafts: 2 COMMAND MENU: Submit a Transaction (enter sequence of integers separated by spaces) Show Current [B]alance Show Number of [O]verdrafts [Q]uit Program ENTER COMMAND: q ============ Thank you for using this App!!!! ============
Step by Step Solution
There are 3 Steps involved in it
Step: 1
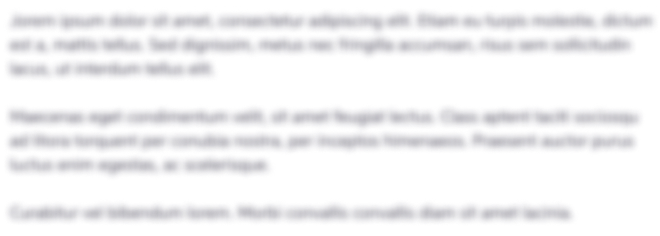
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started