Question
Java... a. Create a new JUnit test case: i. Name the new class SellTicketTest. ii. Ask for method stubs for setUpBeforeClass() and tearDownAfterClass(). iii. Leave
Java...
a. Create a new JUnit test case: i. Name the new class SellTicketTest. ii. Ask for method stubs for setUpBeforeClass() and tearDownAfterClass(). iii. Leave the box Class under test empty and click Finish.
b. When the skeleton class is generated, add bodies to the setUpBeforeClass() and tearDownAfterClass() methods to print messages to the console as you did for the previous setUp() and tearDown() methods.
If necessary, search on Google or refer to JUnit documentation to recall the difference between JUnit annotations @Before and @BeforeClass and between @After and @AfterClass.
Create a sellTicket1 test method as shown below. The logic of the code is similar to the SeatReserver.sellTicket() method but this test replaces user input with specific values:
@Test public void sellTicket1() { SeatingClass sClass = SeatingClass.ECONOMY; Passenger passenger = new Passenger( new PassengerName("Mary", "I", "Worth")); Seat seat = new Seat(); seat.setRow(3); seat.setLetter('D'); double price = sClass.getPrice(); Ticket ticket = new Ticket(passenger, seat, price); System.out.println("Ticket issued: " + ticket); Assert.assertEquals(ticket.getPrice(), 500.0, 0.005 ); }
c. Add a similar method sellTicket2 to test the case of a selling a ticket to an airline employee who expects to pay half price, $250, for an economy class ticket. For example, instantiate the passenger with:
PassengerName pName = new PassengerName("Mary", "I", "Worth"); StaffPassenger passenger = new StaffPassenger(pName, "EMP123");
d. Add a method sellTicket3 to see what happens when a StaffPassenger tries to buy a business class ticket and expects to pay full fare. Specify business class using an instance of Seating class created with the line:
SeatingClass sClass = SeatingClass.BUSINESS;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
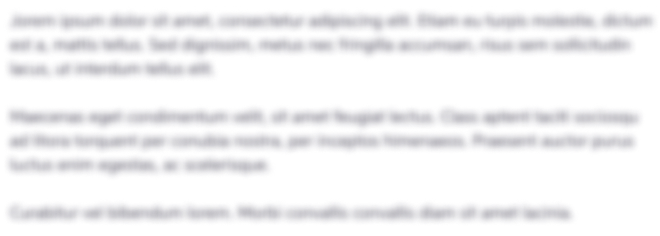
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started