Question
JAVA /** * A simple model for a consumer credit card. * The task is to: * 1. Understand and execute the code, then explain
JAVA
/**
* A simple model for a consumer credit card.
* The task is to:
* 1. Understand and execute the code, then explain why the original Balance displayed for each card is different.
* 2. Modify the class to include a method that updates the credit limit. (2)
* 3. Use the method from Q2 to update the credit limit of the last CreditCard in the wallet to 3000 and display the new card summary
* 4. Modify the makePayment method so that it ignores requests to process a negative payment amount. (2)
* 5. Provide the code to test that your code for Q4 works as expected.
* 6. Modify the printSummary method to a non-static method and modify the main method code to work accordingly with this modification. (2)
* 7. Explain the difference between a static and a non-static method in a class.
* 8. Add a toString() method that returns a String representation of a CreditCard (instead of printing it to the console like printSummary). (2)
* - Use StringBuilder for this method
* 9. Print the details of the wallet again, using the standard println statement to print the String representation of each card.
*/
public class CreditCard {
// Instance variables:
private String customer; // name of the customer (e.g., "John Bowman")
private String bank; // name of the bank (e.g., "California Savings")
private String account; // account identifier (e.g., "5391 0375 9387 5309")
private int limit; // credit limit (measured in dollars)
protected double balance; // current balance (measured in dollars)
// Constructors:
/**
* Constructs a new credit card instance. This is a full-argument constructor
* @param cust the name of the customer (e.g., "John Bowman")
* @param bk the name of the bank (e.g., "California Savings")
* @param acnt the account identifier (e.g., "5391 0375 9387 5309")
* @param lim the credit limit (measured in dollars)
* @param initialBal the initial balance (measured in dollars)
*/
public CreditCard(String cust, String bk, String acnt, int lim, double initialBal) {
customer = cust;
bank = bk;
account = acnt;
limit = lim;
balance = initialBal;
}
/**
* Constructs a new credit card instance with default balance of zero.
* @param cust the name of the customer (e.g., "John Bowman")
* @param bk the name of the bank (e.g., "California Savings")
* @param acnt the account identifier (e.g., "5391 0375 9387 5309")
* @param lim the credit limit (measured in dollars)
*/
public CreditCard(String cust, String bk, String acnt, int lim) {
this(cust, bk, acnt, lim, 0.0); // use a balance of zero as default
}
// Accessor methods:
/** Returns the name of the customer. */
public String getCustomer() { return customer; }
/** Returns the name of the bank */
public String getBank() { return bank; }
/** Return the account identifier. */
public String getAccount() { return account; }
/** Return the credit limit. */
public int getLimit() { return limit; }
/** Return the current balance. */
public double getBalance() { return balance; }
// Update methods:
/**
* Charges the given price to the card, assuming sufficient credit limit.
* @param price the amount to be charged
* @return true if charge was accepted; false if charge was denied
*/
public boolean charge(double price) { // make a charge
if (price + balance > limit) // if charge would surpass limit
return false; // refuse the charge
// at this point, the charge is successful
balance += price; // update the balance
return true; // announce the good news
}
/**
* Processes customer payment that reduces balance.
* @param amount the amount of payment made
*/
public void makePayment(double amount) { // make a payment
balance -= amount;
}
// Utility method to print a card's information
public static void printSummary(CreditCard card) {
System.out.println("Customer = " + card.customer);
System.out.println("Bank = " + card.bank);
System.out.println("Account = " + card.account);
System.out.println("Balance = " + card.balance); // implicit cast
System.out.println("Limit = " + card.limit); // implicit cast
}
public static void main(String[] args) {
CreditCard[] wallet = new CreditCard[3];
wallet[0] = new CreditCard("John Bowman", "California Savings",
"5391 0375 9387 5309", 5000);
wallet[1] = new CreditCard("John Bowman", "California Federal",
"3485 0399 3395 1954", 3500);
wallet[2] = new CreditCard("John Bowman", "California Finance",
"5391 0375 9387 5309", 2500, 300);
for (int val = 1; val <= 16; val++) {
wallet[0].charge(3*val);
wallet[1].charge(2*val);
wallet[2].charge(val);
}
for (CreditCard card : wallet) {
CreditCard.printSummary(card); // calling static method
while (card.getBalance() > 200.0) {
card.makePayment(200);
System.out.println("New balance = " + card.getBalance());
}
System.out.println();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
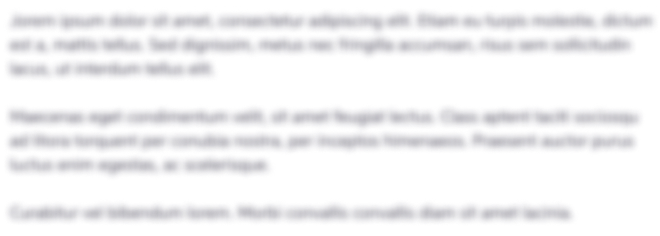
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started