Question
Java Android Programming In this exercise, you'll experiment with the menus and settings that are available from the Tip Calculator app. 1. Open the project
Java Android Programming
In this exercise, you'll experiment with the menus and settings that are available from the Tip Calculator app.
1. Open the project named ch08_ex1_TipCalculator,
2. Run the app. If the device or emulator has a physical Menu button, use it to display the menu. Otherwise, use the action overflow icon to display the menu. This menu should have two items: Settings and About.
3. Select the Settings item to display the Settings activity. Then, click the Back button to return to the main activity
4 Select the About item to display the About activity. This activity should appear as a dialog box. Then, click outside of the dialog box to return to the
main activity 5. Open the XML file in the menu directory and modify it so the Settings item is displayed in the action bar if there is enough room This should display an icon for the Settings item in the action bar, but the About item should remain in the options menu
6 Run the app. Then, use the main activity to set the tip percent to 17%.
7. Select the Settings action item to display the Settings activity. Then, remove the check from the Remember Tip Percent box. Next, click the Back button to return to the main activity. The tip percent should be reset to its default value of 159.
8. Use the main activity to set the tip percent to 17.
9. Change the orientation of the activity. Since the app isn't remembering the tip percent, this should reset the tip percent to 15%,
10. Select the Settings action item to display the Settings activity.
11. Use the Settings activity to select the "Remember Tip Percent" check box 12. Use the Settings activity to change the default rounding for the app so that the total is always rounded.
13. Use the main activity to increase or decrease the tip. To do that you may
need to click on the Increase (+) and Decrease (-) buttons several times. This should automatically round the total and adjust the tip percent accordingly. 14. Open the XML file for the menu and delete the icon attribute from the Settings item. This should display text for the Settings item in the action bar.
15. View the files in the resterawable-xhdpi directory of the project. This directory should include a second Settings icon 16. Open the XML file for the menu and modify it so the Settings item uses the new Settings icon. This should display the new Settings icon in the action bar
mainActivity.java
package edu.rowan.ex8_1; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import androidx.appcompat.widget.Toolbar; import android.view.View; import android.view.Menu; import android.view.MenuItem; import java.text.NumberFormat; import android.preference.PreferenceManager; import android.view.KeyEvent; import android.view.View.OnClickListener; import android.view.inputmethod.EditorInfo; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.TextView.OnEditorActionListener; import android.content.Intent; import android.content.SharedPreferences; import android.content.SharedPreferences.Editor; public class MainActivity extends AppCompatActivity implements OnEditorActionListener, OnClickListener { // define variables for the widgets private EditText billAmountEditText; private TextView percentTextView; private Button percentUpButton; private Button percentDownButton; private TextView tipTextView; private TextView totalTextView; // define instance variables that should be saved private String billAmountString = ""; private float tipPercent = .15f; // define rounding constants private final int ROUND_NONE = 0; private final int ROUND_TIP = 1; private final int ROUND_TOTAL = 2; // set up preferences private SharedPreferences prefs; private boolean rememberTipPercent = true; private int rounding = ROUND_NONE; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); // get references to the widgets billAmountEditText = (EditText) findViewById(R.id.billAmountEditText); percentTextView = (TextView) findViewById(R.id.percentTextView); percentUpButton = (Button) findViewById(R.id.percentUpButton); percentDownButton = (Button) findViewById(R.id.percentDownButton); tipTextView = (TextView) findViewById(R.id.tipTextView); totalTextView = (TextView) findViewById(R.id.totalTextView); // set the listeners billAmountEditText.setOnEditorActionListener(this); percentUpButton.setOnClickListener(this); percentDownButton.setOnClickListener(this); // set the default values for the preferences PreferenceManager.setDefaultValues(this, R.xml.preferences, false); // get default SharedPreferences object prefs = PreferenceManager.getDefaultSharedPreferences(this); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public void onPause() { // save the instance variables Editor editor = prefs.edit(); editor.putString("billAmountString", billAmountString); editor.putFloat("tipPercent", tipPercent); editor.commit(); super.onPause(); } @Override public void onResume() { super.onResume(); // get preferences rememberTipPercent = prefs.getBoolean("pref_remember_percent", true); rounding = Integer.parseInt(prefs.getString("pref_rounding", "0")); // get the instance variables billAmountString = prefs.getString("billAmountString", ""); if (rememberTipPercent) { tipPercent = prefs.getFloat("tipPercent", 0.15f); } else { tipPercent = 0.15f; } // set the bill amount on its widget billAmountEditText.setText(billAmountString); // calculate and display calculateAndDisplay(); } public void calculateAndDisplay() { // get the bill amount billAmountString = billAmountEditText.getText().toString(); float billAmount; if (billAmountString.equals("")) { billAmount = 0; } else { billAmount = Float.parseFloat(billAmountString); } // calculate tip and total float tipAmount = 0; float totalAmount = 0; float tipPercentToDisplay = 0; if (rounding == ROUND_NONE) { tipAmount = billAmount * tipPercent; totalAmount = billAmount + tipAmount; tipPercentToDisplay = tipPercent; } else if (rounding == ROUND_TIP) { tipAmount = StrictMath.round(billAmount * tipPercent); totalAmount = billAmount + tipAmount; tipPercentToDisplay = tipAmount / billAmount; } else if (rounding == ROUND_TOTAL) { float tipNotRounded = billAmount * tipPercent; totalAmount = StrictMath.round(billAmount + tipNotRounded); tipAmount = totalAmount - billAmount; tipPercentToDisplay = tipAmount / billAmount; } // display the other results with formatting NumberFormat currency = NumberFormat.getCurrencyInstance(); tipTextView.setText(currency.format(tipAmount)); totalTextView.setText(currency.format(totalAmount)); NumberFormat percent = NumberFormat.getPercentInstance(); percentTextView.setText(percent.format(tipPercentToDisplay)); } @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { int keyCode = -1; if (event != null) { keyCode = event.getKeyCode(); } if (actionId == EditorInfo.IME_ACTION_DONE || actionId == EditorInfo.IME_ACTION_UNSPECIFIED || keyCode == KeyEvent.KEYCODE_DPAD_CENTER || keyCode == KeyEvent.KEYCODE_ENTER) { calculateAndDisplay(); } return false; } @Override public void onClick(View v) { switch (v.getId()) { case R.id.percentDownButton: tipPercent = tipPercent - .01f; calculateAndDisplay(); break; case R.id.percentUpButton: tipPercent = tipPercent + .01f; calculateAndDisplay(); break; } } @Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.action_settings: // Toast.makeText(this, "Settings", Toast.LENGTH_SHORT).show(); startActivity(new Intent(getApplicationContext(), SettingsActivity.class)); return true; case R.id.menu_about: // Toast.makeText(this, "About", Toast.LENGTH_SHORT).show(); startActivity(new Intent(getApplicationContext(), AboutActivity.class)); return true; default: return super.onOptionsItemSelected(item); } } }
about.java
package edu.rowan.ex8_1; import android.os.Bundle; import android.app.Activity; public class AboutActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_about); } }
settings.java
package edu.rowan.ex8_1; import android.app.Activity; import android.os.Bundle; public class SettingsActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Display the fragment as the main content. getFragmentManager().beginTransaction() .replace(android.R.id.content, new SettingsFragment()) .commit(); } }
preferences.xml
"1.0" encoding="utf-8"?>"http://schemas.android.com/apk/res/android"> "pref_remember_percent" android:title="@string/remember_percent_title" android:summary="@string/remember_percent_summary" android:defaultValue="true" /> "pref_rounding" android:title="@string/rounding_title" android:summary="@string/rounding_summary" android:dialogTitle="@string/rounding_title" android:entries="@array/rounding_keys" android:entryValues="@array/rounding_values" android:defaultValue="@string/rounding_default" /> </PreferenceScreen>
settings.fragment.java
package edu.rowan.ex8_1; import android.os.Bundle; import android.preference.PreferenceFragment; public class SettingsFragment extends PreferenceFragment { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Load the preferences from an XML resource addPreferencesFromResource(R.xml.preferences); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
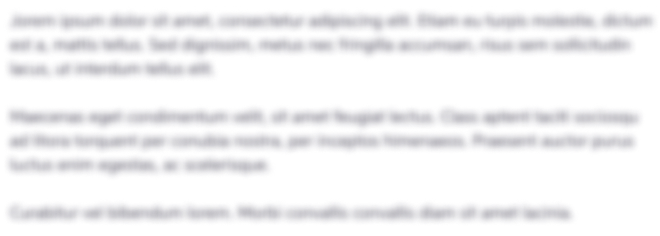
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started