Question
//Java: Cipher and decipher public class Message { public String message; public int lengthOfMessage; public Message (String m){ message = m; lengthOfMessage = m.length(); this.makeValid();
//Java: Cipher and decipher
public class Message {
public String message;
public int lengthOfMessage;
public Message (String m){
message = m;
lengthOfMessage = m.length();
this.makeValid();
}
public Message (String m, boolean b){
message = m;
lengthOfMessage = m.length();
}
/**
* makeValid modifies message to remove any character that is not a letter and turn Upper Case into Lower Case
*/
public void makeValid(){
//INSERT YOUR CODE HERE
}
/**
* prints the string message
*/
public void print(){
System.out.println(message);
}
/**
* tests if two Messages are equal
*/
public boolean equals(Message m){
if (message.equals(m.message) && lengthOfMessage == m.lengthOfMessage){
return true;
}
return false;
}
/**
* caesarCipher implements the Caesar cipher : it shifts all letter by the number 'key' given as a parameter.
* @param key
*/
public void caesarCipher(int key){
// INSERT YOUR CODE HERE
}
public void caesarDecipher(int key){
this.caesarCipher(- key);
}
/**
* caesarAnalysis breaks the Caesar cipher
* you will implement the following algorithm :
* - compute how often each letter appear in the message
* - compute a shift (key) such that the letter that happens the most was originally an 'e'
* - decipher the message using the key you have just computed
*/
public void caesarAnalysis(){
// INSERT YOUR CODE HERE
}
/**
* vigenereCipher implements the Vigenere Cipher : it shifts all letter from message by the corresponding shift in the 'key'
* @param key
*/
public void vigenereCipher (int[] key){
// INSERT YOUR CODE HERE
}
/**
* vigenereDecipher deciphers the message given the 'key' according to the Vigenere Cipher
* @param key
*/
public void vigenereDecipher (int[] key){
// INSERT YOUR CODE HERE
}
/**
* transpositionCipher performs the transition cipher on the message by reorganizing the letters and eventually adding characters
* @param key
*/
public void transpositionCipher (int key){
// INSERT YOUR CODE HERE
}
/**
* transpositionDecipher deciphers the message given the 'key' according to the transition cipher.
* @param key
*/
public void transpositionDecipher (int key){
// INSERT YOUR CODE HERE
}
}
-----------------------------------------------------------------------------------------------------------------------------------------------------------------
public class Tester {
public static void main(String[] args) {
//Testing makeValid
String originalMessage = "It's been snowing, now there's ice everywhere.";
Message M1 = new Message(originalMessage);
if (M1.equals(new Message("itsbeensnowingnowtheresiceeverywhere"))){
System.out.println("makeValid seems correct.");
}
else{
System.out.println("makeValid is incorrect.");
}
//Testing Caesar cipher
originalMessage = "Are you going to get the first one ?";
M1 = new Message(originalMessage);
int key1 = 5;
M1.caesarCipher(key1);
if (M1.equals(new Message ("fwjdtzltnslytljyymjknwxytsj"))) {
System.out.println("Caesar cipher seems correct.");
}
else {
System.out.println("Caesar cipher is incorrect.");
}
//Testing Caesar decipher
M1 = new Message ("fwjdtzltnslytljyymjknwxytsj");
M1.caesarDecipher(key1);
if (M1.equals(new Message (originalMessage))) {
System.out.println("You paid attention to the range of caesarCipher");
}
else {
System.out.println("the range of caesarCipher is wider than what you coded");
}
//Testing Caesar analysis
originalMessage = "Attenborough is widely considered a national treasure in Britain, although he himself does not like the term.[6][7][8] In 2002 he was named among the 100 Greatest Britons following a UK-wide poll for the BBC.[9] He is the younger brother of the late director, producer and actor Richard Attenborough,[10] and older brother of the late motor executive John Attenborough.";
String codedMessage = "fyyjsgtwtzlmnxbnijqdhtsxnijwjifsfyntsfqywjfxzwjnsgwnyfnsfqymtzlmmjmnrxjqkitjxstyqnpjymjyjwrnsmjbfxsfrjifrtslymjlwjfyjxygwnytsxktqqtbnslfzpbnijutqqktwymjgghmjnxymjdtzsljwgwtymjwtkymjqfyjinwjhytwuwtizhjwfsifhytwwnhmfwifyyjsgtwtzlmfsitqijwgwtymjwtkymjqfyjrtytwjcjhzynajotmsfyyjsgtwtzlm" ;
Message M2 = new Message(codedMessage);
M2.caesarAnalysis();
if (M2.equals(new Message (originalMessage))) {
System.out.println("Caesar analysis seems correct.");
}
else {
System.out.println("Caesar analysis is incorrect.");
}
//Testing Vigenere Cipher
originalMessage = "Harder now : go for the Vigenere cipher";
Message M3 = new Message(originalMessage);
int[] key3 = {1,2,1,0};
M3.vigenereCipher(key3);
if (M3.equals(new Message("icsdftooxipfptuhfxjgfpfrfejpigs"))) {
System.out.println("Vigenere cipher seems correct.");
}
else {
System.out.println("Vigenere cipher is incorrect.");
}
//Testing Vigenere Decipher
M3 = new Message("icsdftooxipfptuhfxjgfpfrfejpigs");
M3.vigenereDecipher(key3);
if (M3.equals(new Message(originalMessage))){
System.out.println("Vigenere decipher seems correct.");
}
else{
System.out.println("Vigenere decipher is incorrect.");
}
//Testing transposition cipher
originalMessage = "Last one to code and you are good for this assignment !! :)";
Message M4 = new Message(originalMessage);
int key4 = 6;
M4.transpositionCipher(key4);
if (M4.equals(new Message ("leeuohitataadig*sonrfsn*tcdeoam*ooygrse*ndootsn*", false))){
System.out.println("Transposition cipher seems correct.");
}
else{
System.out.println("Transposition cipher is incorrect.");
}
//Testing transposition decipher
M4 = new Message("leeuohitataadig*sonrfsn*tcdeoam*ooygrse*ndootsn*", false);
M4.transpositionDecipher(key4);
if (M4.equals(new Message(originalMessage))){
System.out.println("Transposition decipher seems correct.");
}
else{
System.out.println("Transposition decipher is incorrect.");
}
}
}
2.1 First function Question 1. (10 pts) First, you wil need to implement the method makeValid: it will remove all characters that are not letters from 'a' to 'z' and change upper case letters to their corresponding lower case letters. This is important because leaving spaces, punctuation or upper case let- ters in a message will give information to the people who want to understand your message but should not have access to it (this is called cryptanalysis). 2.2 Caesar Cipher The Caesar cipher is one of the easiest and oldest ciphers. In this case the key is a number n. To encrypt a message, you shift all letters by n, so for instance if the key is 2, 2.1 First function Question 1. (10 pts) First, you wil need to implement the method makeValid: it will remove all characters that are not letters from 'a' to 'z' and change upper case letters to their corresponding lower case letters. This is important because leaving spaces, punctuation or upper case let- ters in a message will give information to the people who want to understand your message but should not have access to it (this is called cryptanalysis). 2.2 Caesar Cipher The Caesar cipher is one of the easiest and oldest ciphers. In this case the key is a number n. To encrypt a message, you shift all letters by n, so for instance if the key is 2Step by Step Solution
There are 3 Steps involved in it
Step: 1
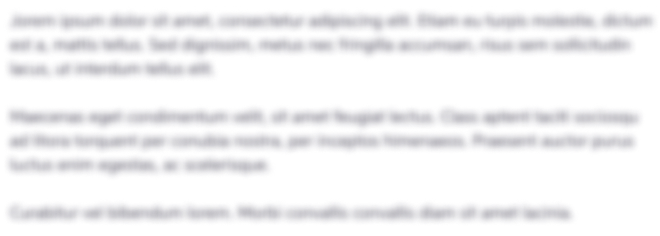
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started