Question
java. classes provided. ADD IN CODE PER THE INSTRUCTIONS. PLEASE READ INSTRUCTIONS. Instructions: Modify the barbers constructor so that it takes a string as a
java. classes provided. ADD IN CODE PER THE INSTRUCTIONS. PLEASE READ INSTRUCTIONS.
Instructions:
Modify the barbers constructor so that it takes a string as a parameter, which is going to be the barbers name. Add the barbers name to the existing print statements.
Since we now have an additional barber, we will also need to pass the second barber to the customer. Make sure you modify the customers constructor accordingly.
The second barber is created through a new instance of the barber class. But, the two instances will have to share many resources. Identify the resources that they will need to share, and make the shared variables static, which means they are now class variables. In addition, to make the class variables thread-safe, change the ArrayList to the appropriate object.
The two barbers share the same customer base, which means the addCustomerToWaiting method should be shared across the two barbers. A class method supports the above functionality and can be trivially achieved by adding the static keyword.
In the customers run method, instead of always waking up the same barber, you will now need to identify the correct barber to wake up. To help you do that, add a Boolean to the barber class, and update the variable in the appropriate places to reflect if the barber is currently sleeping. Use the variable to help you decide which barber to wake up. Remember, if both barbers are sleeping, wake up barber 1. Otherwise, wake up the barber who is sleeping. You should not be waking up both barbers.
Upon the completion of all customer threads, be sure to wake up both barbers, so that they can both go home.
SleepBarber.java
import java.util.ArrayList;
import java.util.Random;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class SleepingBarber extends Thread {
private int maxSeats;
private int totalCustomers;
private ArrayList
private Lock barberLock; //locks
private Condition sleepingCondition;
private boolean moreCustomers;
private static String barberName;
public SleepingBarber(String barberName) {
maxSeats = 3;
totalCustomers = 10;
moreCustomers = true;
customersWaiting = new ArrayList
barberLock = new ReentrantLock(); //locks
sleepingCondition = barberLock.newCondition();
this.start();
SleepingBarber.barberName= barberName;
}
public synchronized boolean addCustomerToWaiting(Customer customer) {
if (customersWaiting.size() == maxSeats) {
return false;
}
Util.printMessage("Customer " + customer.getCustomerName() + " is waiting");
customersWaiting.add(customer);
String customersString = "";
for (int i=0; i < customersWaiting.size(); i++) {
customersString += customersWaiting.get(i).getCustomerName();
if (i < customersWaiting.size() - 1) {
customersString += ",";
}
}
Util.printMessage("Customers currently waiting: " + customersString);
return true;
}
public void wakeUpBarber() {
try {
barberLock.lock(); //locks
sleepingCondition.signal();
} finally {
barberLock.unlock(); //locks
}
}
/*
* addCustomerToWaitingMethod
*/
public void run() {
while(moreCustomers) {
while(!customersWaiting.isEmpty()) {
Customer customer = null;
synchronized(this) {
customer = customersWaiting.remove(0);
}
customer.startingHaircut();
try {
Thread.sleep(1000);
} catch (InterruptedException ie) {
System.out.println(barberName + "ie cutting customer's hair" + ie.getMessage());
}
customer.finishingHaircut();
Util.printMessage("Checking for more customers...");
}
try {
barberLock.lock();
Util.printMessage("No customers, so time to sleep...");
sleepingCondition.await();
Util.printMessage("Someone woke me up!");
} catch (InterruptedException ie) {
System.out.println("ie while sleeping: " + ie.getMessage());
} finally {
barberLock.unlock();
}
}
Util.printMessage("All done for today! Time to go home!");
}
public static void main(String [] args) {
SleepingBarber sb = new SleepingBarber(barberName);
ExecutorService executors = Executors.newCachedThreadPool();
for (int i=0; i < sb.totalCustomers; i++) {
Customer customer = new Customer(i, sb);
executors.execute(customer);
try {
Random rand = new Random();
int timeBetweenCustomers = rand.nextInt(2000);
Thread.sleep(timeBetweenCustomers);
} catch (InterruptedException ie) {
System.out.println("ie in customers entering: " + ie.getMessage());
}
}
executors.shutdown();
// makes the program finish as soon as possible
while(!executors.isTerminated()) {
Thread.yield();
}
Util.printMessage("No more customers coming today...");
sb.moreCustomers = false;
sb.wakeUpBarber();
}
}
Customer.java
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Customer extends Thread {
private int customerName;
private SleepingBarber sb;
private Lock customerLock;
private Condition gettingHaircutCondition;
public Customer(int customerName, SleepingBarber sb) {
this.customerName = customerName;
this.sb = sb;
customerLock = new ReentrantLock();
gettingHaircutCondition = customerLock.newCondition();
}
public int getCustomerName() {
return customerName;
}
public void startingHaircut() {
Util.printMessage("Customer " + customerName + " is getting hair cut.");
}
public void finishingHaircut() {
Util.printMessage("Customer " + customerName + " is done getting hair cut.");
try {
customerLock.lock();
gettingHaircutCondition.signal();
} finally {
customerLock.unlock();
}
}
public void run() {
boolean seatsAvailable = sb.addCustomerToWaiting(this);
if (!seatsAvailable) {
Util.printMessage("Customer " + customerName + " leaving...no seats available.");
return;
}
sb.wakeUpBarber();
try {
customerLock.lock();
gettingHaircutCondition.await();
} catch (InterruptedException ie) {
System.out.println("ie getting haircut: " + ie.getMessage());
} finally {
customerLock.unlock();
}
Util.printMessage("Customer " + customerName + " is leaving.");
}
}
Util.java
import java.util.Calendar;
public class Util {
public static void printMessage(String message) {
Calendar cal = Calendar.getInstance();
String datetime = "" + cal.get(Calendar.YEAR);
datetime += "-" + (cal.get(Calendar.MONTH)+1);
datetime += "-" + cal.get(Calendar.DATE);
datetime += " " + cal.get(Calendar.HOUR_OF_DAY);
datetime += ":" + cal.get(Calendar.MINUTE);
datetime += ":" + cal.get(Calendar.SECOND);
datetime += "." + cal.get(Calendar.MILLISECOND);
System.out.println(datetime + " - " + message);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
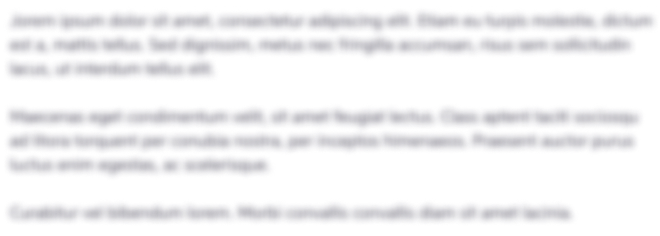
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started