Question
Java code ( Creating your Amoeba Colony program ) Once you are done writing and testing your class structure and algorithm, you are ready to
Java code ( Creating your Amoeba Colony program )
Once you are done writing and testing your class structure and algorithm, you are ready to start coding!
You will need a method that generates a random number again. Heres what you need to do to use it:
i.Include the following code at the top of your class file (so that you can use this class:
import java.util.Random;
To find out more about this, go to http://java.sun.com/javase/7/docs/api/index.html (like you did in Lab Assignment 2)
ii.Youll need to use some variables. Heres how you get a random number:
Random r = new Random();
int x = 1 + r.nextInt(10);
Note that the number in the parens (e.g., 10 above) is the upper limit of the random number. So, the random number that you get here will be an integer between 0 and 10. Need a larger range? Just change the 10 to the top of your range.
Heres another example, in this case if you are printing a random number to the console:
System.out.print( 1 + r.nextInt(5) + " " );
Youll need to use an if-else statement. We havent covered that yet, so heres the structure:
if( variableName1 < variableName2){
// put in what is done if the value of
// variableName is less than the value of
// variableName2
}else{
// put in what is done if the value of
// variableName is greater than or equal to the
// value of variableName2
}
(hint: think about what you need to do with Feed and Breed)
Now start translating your algorithm into java code.
Remember to code and then compile frequently. It will make it easier to find any bugs.
Also remember that you will need to create a tester class (where your main method will reside).
Once you get your program running correctly, there is one more thing to do. Any input requested from the user and/or output received from the user should be in a window (see E.1.14 and E.1.15 from lab 1). At this point, you probably have your output going to the console. For your final submission, it needs to go to a window (JOptionPane). Dont forget any additional libraries that you need to import to do this.
Thats it! Now you can nurture your own amoeba colony, and, chances are, theyll do just fine as your sisters pet! Of course, youll also need to turn it in to Moodle.
++++++++++++++++++++++++++++++++++++++++++++
Pseudocode for Tester Class
1. Initialise the object of AmoebaColony class.
2. TRY
3. Run setColony Properties method of AmoebaColony class.
4. Run setActions method of AmoebaColony class.
5. Run setBreeding method of AmoebaColony class.
6. Run setActionsmethod of AmoebaColony class.
7. Run setVitamins method of AmoebaColony class.
8. Run setSicknessmethod of AmoebaColony class.
9. Run setDeath method of AmoebaColony class.
10. Run finalOutput method of AmoebaColony class.
11. CATCH
12. PRINT ERROR MESSAGE "You entered incorrect or incomplete information!".
Pseudocode for AmoebaColony Class
A. setBreeding() method
1. IF (daysFed >= amountBreed){
2. breedSuccessNumber = amountBreed;
3. ELSE
4. breedSuccessNumber = daysFed;
5. ENDIF
6. FOR (int i = 0; i < breedSuccessNumber; i++)
7. nextAmoeba = previousAmoeba * 2;
8. previousAmoeba = nextAmoeba;
9. ENDFOR
10. nextAmoeba = nextAmoeba;
B. setVitamins() method
1. IF (yesnoVitamins == JOptionPane.YES_OPTION)
2. vitamins = true;
3. ELSE
4. vitamins = false;
5. ENDIF
C. setSickness() method
1. IF(vitamins == true)
2. IF(x <= 20)
3. sick = true;
4. sickness = "Yes";
5. ELSE
6. sick = false;
7. sickness = "No";
8. ENDIF
9. ELSE
10. IF(x <= 25)
11. sick = true;
12. sickness = "Yes";
13. ELSE
14. sick = false;
15. sickness = "No";
16. ENDIF
17. ENDIF
D. SetDeath() method
1. IF(sick == true)
2. finalAmoeba = Math.round(nextAmoeba * .90);
3. ELSE
4. finalAmoeba = nextAmoeba;
5. ENDIF
6. amountDied = nextAmoeba - finalAmoeba;
AmoebaTester.java
package amoebacolony; import javax.swing.JOptionPane;
public class AmoebaTester { public static void main(String[] args){ AmoebaColony newColony = new AmoebaColony(); try{
newColony.setColonyProperties(); newColony.setActions(); newColony.setBreeding(); newColony.setVitamins(); newColony.setSickness(); newColony.setDeath(); newColony.finalOutput(); }catch (NumberFormatException e){ JOptionPane.showMessageDialog(null, "You entered incorrect or incomplete information!", "Error", JOptionPane.ERROR_MESSAGE);
} } }
AmoebaColony.java
package amoebacolony; import javax.swing.JOptionPane; import java.util.Random; public class AmoebaColony { public String colonyName; public String caretakerName; public String sickness; public int startAmoeba; public int nextAmoeba; public int daysFed; public int amountBreed; public double finalAmoeba; public double amountDied; public int yesnoVitamins; public int breedSuccessNumber; public boolean vitamins; public boolean sick; public boolean colonyname; public void setColonyProperties(){ String welcomeMsg = ("Hello and welcome to our program. This program allows you to create and take care of your own amoeba colony!"); String title = ("Welcome!"); JOptionPane.showMessageDialog(null, welcomeMsg, title, JOptionPane.INFORMATION_MESSAGE); colonyName = JOptionPane.showInputDialog("What would you like to name your colony?"); caretakerName = JOptionPane.showInputDialog("What is your name, caretaker?"); startAmoeba = Integer.parseInt(JOptionPane.showInputDialog("How many amoebas would you like to start with?")); }// end setColonyProperties() public void setActions(){ JOptionPane.showMessageDialog(null, "Congratulations, " + caretakerName+ ", you've created your amoeba colony, " + colonyName + ", of " + startAmoeba + " amoebas!", colonyName, JOptionPane.INFORMATION_MESSAGE); JOptionPane.showMessageDialog(null, "Now you can feed your colony, breed your colony, and give them vitamins to prevent sickness!" , colonyName, JOptionPane.INFORMATION_MESSAGE); daysFed = Integer.parseInt(JOptionPane.showInputDialog(null, "How many days would you like to feed your colony?" + " Note: They need one day of food for each time they breed.", "Feeding", JOptionPane.INFORMATION_MESSAGE)); amountBreed = Integer.parseInt(JOptionPane.showInputDialog(null, "How many times would you like to breed your colony?" + " Note: Each successful breed doubles your colony's size." + " Every time they breed requires 1 day worth of food." + " You have chosen to feed your colony " + daysFed + " time(s).", "Breeding", JOptionPane.INFORMATION_MESSAGE)); yesnoVitamins = JOptionPane.showConfirmDialog(null, "Would you like to give your colony vitamins?" + " Note: Giving vitamins reduces the chance of your colony getting sick.", "Vitamins", JOptionPane.INFORMATION_MESSAGE, JOptionPane.YES_NO_OPTION); //Above is asking user what they would like to do about feeding, breeding, and vitamins }// end setActions() public void setBreeding(){ if (daysFed >= amountBreed){ breedSuccessNumber = amountBreed; } else{ breedSuccessNumber = daysFed; } int previousAmoeba = startAmoeba; for (int i = 0; i < breedSuccessNumber; i++){ nextAmoeba = previousAmoeba * 2; previousAmoeba = nextAmoeba; } nextAmoeba = nextAmoeba; }// end setBreeding() public void setVitamins(){ if (yesnoVitamins == JOptionPane.YES_OPTION){ vitamins = true; }else if(yesnoVitamins ==JOptionPane.NO_OPTION){ vitamins = false; } //decision to give vitamins }// end setVitamins() public void setSickness(){ Random r = new Random(); int x = 1 + r.nextInt(100); if(vitamins == true){ if(x <= 20){ sick = true; sickness = "Yes"; }else{ sick = false; sickness = "No"; } } else{ if(x <= 25){ sick = true; sickness = "Yes"; }else{ sick = false; sickness = "No"; } } }// end setSickness() public void setDeath(){ if(sick == true){ finalAmoeba = Math.round(nextAmoeba * .90); }else{ finalAmoeba = nextAmoeba; } amountDied = nextAmoeba - finalAmoeba; }// end setDeath() public void finalOutput(){ JOptionPane.showMessageDialog(null, "Summary of your new Amoeba Colony!:" + " Colony Name: " + colonyName + " Caretaker Name: " + caretakerName + " Starting Size: " + startAmoeba + " amoeba(s)." + " How many times fed: " + daysFed + " Number of times to breed request: " + amountBreed + " Number of successful breeds: " + breedSuccessNumber + " Sickness?: " + sickness + " How many died?: " + amountDied + " Final number of amoebas in colony: " + finalAmoeba); } }
++++++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
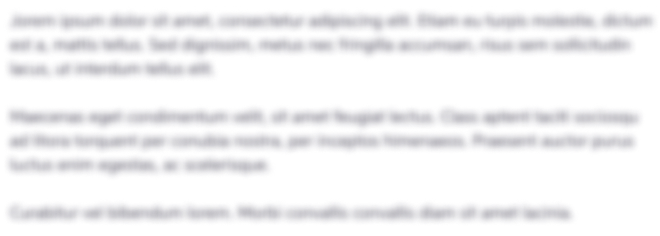
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started