Question
Java Code:: In the board game Risk, the player's goal is to conquer the world by occupying every country. The play involves neighboring countries fighting
Java Code::
In the board game Risk, the player's goal is to conquer the world by occupying every country. The play involves neighboring countries fighting battles. Most battles in Risk involve four armies, two from the attacking country, and two from the defending country.
The process for fighting a battle involves the attacker rolling three dice, and the defender rolling two dice. We will assume these are typical 6-sided dice with values 1 through 6, inclusive. The highest die of the attacker is compared with the highest die of the defender, and the second-highest die of the attacker is compared with the second-highest die of the defender. If both of the attacker's dice are higher than the defender's, the attacker wins the battle and the defender loses two armies. If both of the defender's dice are higher than or equal to the attacker's, the defender wins the battle and the attacker loses two armies. Otherwise, each player loses one army. Some examples follow:
Attacker | Defender | Comparison | Result |
1 5 4 | 4 2 | attacker 5 > defender 4, attacker 4 > defender 2 | attacker wins, defender loses two armies |
4 3 4 | 2 4 | attacker 4 <= defender 4, attacker 4 > defender 2 | tie, each player loses one army |
3 4 3 | 5 3 | attacker 4 <= defender 5, attacker 3 <= defender 3 | defender wins, attacker loses two armies |
Programming Test
You are to write the following program. Write the program exactly as specified. Do not "improve" it. Do not use language elements that we have not covered. Remember to use good coding practices, such as following the Java naming convention and formatting your code.
Write a class BattleUtils in the default package containing the following public static methods.
A public static method newDieVal that has no parameters and returns an int random value between 1 and 6 (inclusive).
Overloaded public static methods biggestDie that take either three or two int parameters. biggestDiereturns an int that is the largest of its parameter values.
Overloaded public static methods secondDie that take either three or two int parameters. secondDiereturns an int value that is the second-highest value of its parameters. Note that the second highest value of (5, 4, 5) is 5, since we consider that the highest value is one of the 5s, and the "other" 5 is the second highest. Similarly, the second highest value of (4, 4) is 4; the highest value is one of the 4s, and the second highest is the "other" 4.
A public static method battleScore. The parameter list for battleScore is four int values, and they are, in order, attackerHighestDie, attackerSecondHighestDie, defenderHighestDie, defenderSecondHighestDie. The method returns an int value of
1 if the attacker wins the battle and the defender loses two armies
-1 if the defender wins the battle and the attacker loses two armies
0 if there is a tie and the attacker and defender lose one army each
Write a class BattleProcessor in the default package that has only a main method. The main method
uses a Scanner and a sentinel-controlled loop to query the user for the number of battles to simulate. If the user enters -1, the program exits.
Uses a nested counter-controlled loop to simulate the number of battles specified by the user.
For each battle, the program
Uses newDieVal to determine the values of the attacker's three dice and the defender's two dice.
Uses biggestDie to determine the highest die value for each player.
Uses secondDie to determine the second-highest die value for each player.
Uses battleScore to determine the outcome of the battle.
As the simulations are run, the program totals how many battles the attacker wins, how many the defender wins, and how many are ties.
After the specified simulations have finished, the program prints the information specified in Program Output, below.
The program then queries the user for another number of battle simulations, or -1 to exit.
Program Output
After the outcome of the simulated battles has been determined, main should
print the percentages of the battles the attacker won, the defender won, and ties.
print the total number of armies lost by the attacker and defender. (Don't forget that the ties also factor into the armies lost.)
Sample Output
In the following sample, the user runs a simulation of five battles, then 1,000,000 battles, and then enters -1 to exit.
Enter the number of battles to simulate, or -1 to exit 5 defender loses 8 armies attacker loses 2 armies the attacker wins 80.0 percent of the battles the defender wins 20.0 percent of the battles they tie 0.0 percent of the battles Enter the number of battles to simulate, or -1 to exit 1000000 defender loses 1079216 armies attacker loses 920784 armies the attacker wins 37.1807 percent of the battles the defender wins 29.2591 percent of the battles they tie 33.5602 percent of the battles Enter the number of battles to simulate, or -1 to exit -1 |
Submission
When your program is complete, place your two Java source files into a single zip file containing no directories and submit it as your response in Blackboard.
Hints
Write and test one method, then move on to the next and write and test it. For example, start with the main method. Create a Scanner and sentinel-controlled loop and ask the user how many battles to fight or to enter -1 to exit. Test this loop. Then write your counter-controlled loop and in it, just print "fighting battle "
Grading Elements
Five points each:
all classes and methods named correctly
all methods are in the proper class
all methods have correct headers, return types, signatures
all methods return the correct value
main method uses a sentinel-controlled loop to ask the user for the number of battles to simulate or to exit. It uses a counter-controlled loop to simulate the battles.
main method uses the specified utility methods to perform each simulated battle
main method prints the requested output
the program follows procedures and conventions established by your instructor and is submitted correctly.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
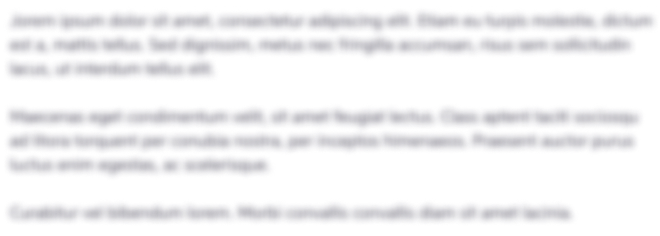
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started