Question
JAVA CODE, NEW ANSWER, NOT THE SAME AS OTHERS Problems 6-10 SeatRow and Airplane Implement a program that assigns seats on an airplane. Assume the
JAVA CODE, NEW ANSWER, NOT THE SAME AS OTHERS Problems 6-10 SeatRow and Airplane
Implement a program that assigns seats on an airplane. Assume the airplane has 20 seats in first class (5 rows of 4 seats each, separated by an aisle) and 90 seats in economy class (15 rows of 6 seats each, separated by an aisle).
Your program will take three commands: add passengers, show seating, and quit. When passengers are added, the program will ask for the class (first or economy), the number of passengers traveling together (1 or 2 in first class; 1 to 3 in economy), and the seating preference (aisle or window in first class; aisle, center, or window in economy). Then try to find a match and assign the seats.
Some more details:
Passengers traveling together must always be assigned seats next to each other, in the same row, or else no match is made.
At least one member of their party must be given the seat preference, or else no match is made.
If the user enters something out of range for num passengers or not matching one of the correct preferences, no match is made.
Fill in the seats from front to back, and left to right, after considering the preference and num passengers of each seating assignment request.
Every first class seat is considered an aisle seat, since there is an aisle in between each seat. The window seats in first class can be considered both aisle and window seats.
You don't need to handle any invalid user input. Assume it will all be valid.
The driver / user interface class, AirplaneSeatAssigner, has already been implemented as is given here: AirplaneSeatAssigner.java Download AirplaneSeatAssigner.java. Your task is to implement the SeatRow and Airplane classes, using these templates: SeatRow.java Download SeatRow.java, Airplane.java. Download Airplane.java. Notice the templates have more details about each class.
Example:
A)dd S)how Q)uit: aF)irst E)conomy: fPassengers? (1-2): 2
A)isle W)indow: w
A)dd S)how Q)uit: aF)irst E)conomy: fPassengers? (1-2): 1A)isle W)indow: wA)dd S)how Q)uit: aF)irst E)conomy: ePassengers? (1-3): 3
A)isle C)enter W)indow: w
A)dd S)how Q)uit: aF)irst E)conomy: e
Passengers? (1-3): 2 A)isle C)enter W)indow: w A)dd S)how Q)uit: s 1 [* * . *] 2 [. . . .] 3 [. . . .] 4 [. . . .] 5 [. . . .] 6 [*** .**] 7 [... ...] 8 [... ...] 9 [... ...] 10 [... ...] 11 [... ...] 12 [... ...] 13 [... ...] 14 [... ...] 15 [... ...] 16 [... ...] 17 [... ...] 18 [... ...] 19 [... ...] 20 [... ...] A)dd S)how Q)uit: q AirplaneSeatAssigner.java
import java.util.Scanner; /** * An airplane system menu. */ public class AirplaneSeatAssigner { private Scanner in; private Airplane plane; /** * Constructs an AirplaneMenu object. */ public AirplaneSeatAssigner() { in = new Scanner(System.in); plane = new Airplane(); } /** * Runs the system. */ public void run() { boolean done = false; while (!done) { System.out.print("A)dd S)how Q)uit: "); String command = in.nextLine().toUpperCase(); if (command.equals("A")) { System.out.print("F)irst E)conomy: "); String tclass = in.nextLine().toUpperCase(); if (tclass.equals("F")) { System.out.print("Passengers? (1-2): "); int num = in.nextInt(); in.nextLine(); // extra input String seat_pref = ""; if (num <= 2) { System.out.print("A)isle W)indow: "); seat_pref = in.nextLine().toUpperCase(); } boolean success = false; if (seat_pref.equals("A")) { success = plane.addPassengers(Airplane.FIRST, num, SeatRow.AISLE); } else if (seat_pref.equals("W")) { success = plane.addPassengers(Airplane.FIRST, num, SeatRow.WINDOW); } if (!success) { System.out.println("Assignment did not succeed."); } } else if (tclass.equals("E")) { System.out.print("Passengers? (1-3): "); int num = in.nextInt(); in.nextLine(); String seat_pref = "";
if (num <= 3) { System.out.print("A)isle C)enter W)indow: "); seat_pref = in.nextLine().toUpperCase(); } boolean success = false; if (seat_pref.equals("A")) { success = plane.addPassengers(Airplane.ECONOMY, num, SeatRow.AISLE); } else if (seat_pref.equals("C")) { success = plane.addPassengers(Airplane.ECONOMY, num, SeatRow.CENTER); } else if (seat_pref.equals("W")) { success = plane.addPassengers(Airplane.ECONOMY, num, SeatRow.WINDOW); } if (!success) { System.out.println("Assignment did not succeed."); } } } else if (command.equals("S")) { System.out.print(plane.toString()); } else if (command.equals("Q")) { done = true; } } } public static void main(String[] args) { new AirplaneSeatAssigner().run(); } }
SeatRow.java
/** * Airline seating. For Part I, complete the SeatRow class that represents a row * of seats. Use the following format to represent a row of seats as a string: * [. . . .] (first-class empty), [* * * *] (first class all full), [... ...] * (economy empty). */ public class SeatRow { public static final int WINDOW = 0; public static final int CENTER = 1; public static final int AISLE = 2; // ADD YOUR INSTANCE VARIABLES EHRE /** * Constructs a SeatingRow object. * * @param numSeats the number of seats in this row; */ public SeatRow(int numSeats) { // FILL IN } /** * Adds passenger(s)s to seat(s), based on preference. * * @param numPassengers the number of passengers * @param preference where the passengers want to be seated * @returns true if the passenger can be added, false otherwise */ public boolean addPassengers(int numPassengers, int preference) { return false; // FIX ME } /** * Adds (a) passenger(s) to a seat, if possible * * @param from a starting seat, inclusive * @param to an ending seat, inclusive * @return true if all the seat(s) in the range can be added, false otherwise */ public boolean add(int from, int to) { return false; // FIX ME } /** * Gets a string displaying the seating of this row * * @return the seating chart */ public String toString() { return ""; // FIX ME }
}
Airplane.java /** * Airline Seating. Part II. Complete the Airplane class here */ public class Airplane { public static final int FIRST = 0; public static final int ECONOMY = 1; public static final int FIRST_CLASS_ROWS = 5; public static final int ECONOMY_CLASS_ROWS = 15; public static final int SEATS_IN_FIRST_CLASS_ROW = 4; public static final int SEATS_IN_ECONOMY_CLASS_ROW = 6; /** * Constructs an Airplane object. */ public Airplane() { // FILL IN } /** * Adds the passengers to the plane. * * @param tclass the type of class * @param npassenger the number of passengers * @param where where the passenger wants to sit * @return true if the assignment succeeded. */ public boolean addPassengers(int tclass, int npassenger, int where) { return false; // FIX ME } /** * Gets a string displaying the seating of the airplane. * * @return the seating chart */ public String toString() { return ""; // FIX ME } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
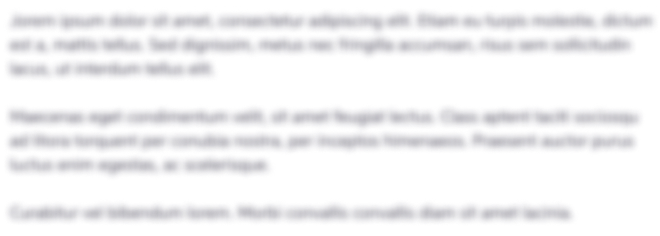
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started