Question
Java Coding 1 Goals and Overview. 1. To complete implementation of the menu-based system started in P4. 2. To implement phase 2 of a 5-phase
Java Coding
1 Goals and Overview. 1. To complete implementation of the menu-based system started in P4. 2. To implement phase 2 of a 5-phase well-structured project with two or three classes at this point. Overview Program 5 completes implementation of the menu and creates a polymorphic Employee class. This is the nal menu-driven application. The following changes need to be made: 1. Code must be written to execute more menu options: 3. List employees, 4. Change Employee Data, 5. Terminate an employee and 6. Pay employees 2. Add polymorphism to this project by making Employee a base class with two subclasses: Hourly and Salaried. Employee will become an abstract class with an abstract method: public abstract double getPay(); 3. Both subclasses, Hourly and Salaried, must implement the method getPay() using the proper calculation for this employee type. 4. Because the data is now polymorphic, we will write out the database as an OBJECT le, not as a TEXT le. Refer to the demo program called FileDemo. 2 Changes to The Employee Class. An object of type Employee will have the same data members and functions as P4 with the following changes: 1. Change the data members from private to protected so that the subclasses can access them. 2. Make this class abstract by adding an abstract method: getPay(). This method must be implemented in the two subclasses, Hourly and Salaried. In Employee, the prototype must be: public abstract double getPay() ;3 The Derived Employee Classes
Create two new classes: Salaried and Hourly by extending Employee. 1. To make a subclass of Employee, use extends on the rst line of your class: public class Hourly extends Employee { 2. The constructors for the derived classes should have 3 arguments to match the Employeeconstructor. When you call Salaried or new Hourly(), an entire object is allocated, including space for the data members inherited from Employee. The constructers for Salaried and Hourly will be called, and they must populate (store data into) the elds inherited from the superclass. 3. You must implement the getPay() method inherited from the superclass. In the Hourly class, write a method for getPay() that prompts for the number of hours worked during this pay period (half a month). Then calculate the pay by multiplying the pay rate by the number of hours worked. Return the answer. 4. In the Salaried class, write a method for getPay() that calculates the pay by dividing the pay rate by 24. Return the answer. 4 Changes to the Payroll Class 1. Add a second ArrayList to Payroll to store employees who have quit or been red. 2. Add a printWriter for a second output le named payroll.txt. Open it in the constructor. 3. Change the database output le to an Object le. Open it in the constructor. 4. Make sure your nally clause, after the menu loop, closes both les. It must also display on the screen all of the employees who have quit or been red on this run. 5. Implement listEmployees(). If the current user is the Boss (User #0), display a list of everyone in the database. If the current user is NOT the boss, display the current Users personal information. 6. Implement changeEmployeeData(). If the current user is the Boss (User #0), let him change the name (people get married and divorced) or the salary of an employee. 7. Add code to newEmployee() to make sure the login name is unique (no duplicates) before you accept it. This means that you must compare the entered login name to the login name of each of the Employee objects in the collection. Use a for-each loop to do this search. 8. Write a function to terminate an employee. Any employee can quit when he is logged in, and the Boss can re anyone. If the current user is NOT the boss, nd that Employee object in the ArrayList, remove it, and add it to the other ArrayList of terminated employees. If the current user is the Boss (User #0), prompt for the ID number of the employee to re, then locate that employee in the ArrayList remove it, and add it to the ArrayList of terminated employees. 9. Write a function to pay every employee currently in the ArrayList. Print the title Payroll Report and the current date. Print a line of column headers. Then use a for-each loop to walk down the list, using each employee to call its proper getPay() method. Display one line on the screen with the employees pay, ID, and name. Make the columns line up neatly and format the pay with two decimal places. Write the same information in the same format to an output le called payroll.txt
5 The Main Function for P5 The main() function can be in a separate class called Main or it can be inside the Payroll class. The rst line of this method should print a title line to the console (the program name and your name). Then instantiate a Payroll object and call the Payroll objects doMenu() function. Surround the code with a try block and catch IOExceptions. When caught, print a comment, a stack trace, and abort.
6 Testing and Submission. When you are sure your program works, delete the database le. Then follow the testing procedure below.
6.1 Testing 1. Start the program and enter your own name. Make up a logon name and a salary. Program Program 5: Payroll CS 6617 Spring 2015 3 2. Login and create one Employee. 3. List all the employees, then exit. 4. Copy the screen contents to a le named run1screen.txt or .docx if you use screen shots. 5. Copy the database le and give it the name run1.txt 6. Start the program again. Enter 3 more imaginary Employees, making 5 altogether. 7. Fire the employee you entered the rst day. 8. Be sure you have tested all menu options. Logout. 9. Log in as one of the new employees. Verify that the right data shows up. 10. Try to list all the employees: the code should not permit you to do so. Then quit and log out. 11. Log in as the Boss and list all the employees. 12. Pay all employees then exit. 13. Copy the screen contents to a le named run2screen.txt or .docx if you use screen shots. Make sure you see the list of terminated employees.
6.2 Submission Hand in a zipped folder containing: The .java les for all your classes. The output les from runs 1 and 2. Console output from both runs. Two output les: the database and the payroll.
MY CODE.....IT NEEDS TO BE MODIFIED WITH THE ABOVE PROVIDED INFORMATION
import java.util.*;
import java.io.*;
public class Employee{
private static String loginName;
private static int Salary;
private static String empName;
private static int empId;
public static File file;
public static FileWriter fileWriter;
Employee(String lname,int id,int sal,String ename){
loginName = lname;
empId=id;
Salary = sal;
empName = ename;
}
public int LogIn(){
return 0 ;
}
public int EnterEmployees(){
return 0 ;
}
public int ListOfEmployees(){
return 0 ;
}
public int TerminateAnEmployee(){
return 0 ;
}
public int PayEmployees(){
return 0 ;
}
public int ExitSystem(){
return 0 ;
}
public static void Payroll_menu() {
System.out.println ( "1) Log In 2) Enter Employees 3) List Of Employees 4) Terminate An Employee 5) Pay Employees 0) Exit System" );
System.out.print ( "Choose: ") ;
}
public void WriteStringToFile(String l,int i,int s,String e) {
try
{
file = new File("D://run1.txt");
FileWriter fw = new FileWriter(file.getAbsoluteFile(),true);
BufferedWriter bw = new BufferedWriter(fw);
Date date = new Date();
bw.write( l + " " + i + " " + s + " " + e + " " + date.toString());
bw.newLine();
bw.flush();
bw.close();
}
catch (IOException eo) {
eo.printStackTrace();
}
}
public void ReadStringFromFile(){
try {
FileReader fileReader = new FileReader(file);
StringBuffer stringBuffer = new StringBuffer();
int read;
char[] array = new char[1024];
while ((read = fileReader.read(array)) > 0) {
stringBuffer.append(array, 0, read);
}
fileReader.close();
System.out.println("File COntents:");
System.out.println(stringBuffer.toString());
System.out.println("Data shown successfully");
}
catch (IOException eox) {
eox.printStackTrace();
}
}
public void removeLineFromFile(String lineToRemove){
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String line = reader.readLine();
reader.close();
file = new File("D://run1.txt");
StringBuffer newContent = new StringBuffer();
BufferedReader br = new BufferedReader(new FileReader(file));
while ((line = br.readLine()) != null) {
if (!line.trim().equals(lineToRemove)) {
newContent.append(line);
newContent.append(" ");
}
}
br.close();
FileWriter removeLine = new FileWriter(file);
BufferedWriter change = new BufferedWriter(removeLine);
PrintWriter replace = new PrintWriter(change);
replace.write(newContent.toString());
replace.close();
}
catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) throws Exception{
Employee emp = new Employee(loginName,empId,Salary,empName);
file = new File("D://run1.txt");
Payroll_menu();
Scanner input=new Scanner(System.in);
switch ( input.nextInt() ) {
case 1:
if (!file.exists()) {
file.createNewFile();
Scanner in=new Scanner(System.in);
System.out.println(" Enter Employee login name ");
loginName = in.nextLine();
System.out.println("Enter Employee Salary ");
Salary = in.nextInt();
System.out.println("Enter Employee Id ");
empId=in.nextInt();
System.out.println(" Enter Employee name ");
empName = in.next();
file = new File("D://run1.txt");
emp.WriteStringToFile(loginName,empId,Salary,empName);
emp.LogIn();
}
else{
System.out.println(" File Alredy exists So Please enter new Employees");
}
break;
case 2:
if (file.exists()) {
Scanner in2=new Scanner(System.in);
System.out.println(" Enter login name for new Employee ");
loginName = in2.nextLine();
System.out.println("Enter Employee id");
empId = in2.nextInt();
System.out.println("Enter Employee Salary ");
Salary = in2.nextInt();
System.out.println(" Enter Employee name ");
empName = in2.next();
file = new File("D://run1.txt");
emp.WriteStringToFile(loginName,empId,Salary,empName);
emp.EnterEmployees();
}
else
{
System.out.println(" File not exists so please Login first ");
}
break;
case 3:
if (file.exists()) {
file.createNewFile();
emp.ReadStringFromFile();
emp.ListOfEmployees();
}
else
{
System.out.println(" File not exists so please Login first ");
}
break;
case 4:
Scanner in3 = new Scanner(System.in);
file = new File("D://run1.txt");
if (file.exists()) {
System.out.println(" Enter the line number to delete from file ");
String lineData = in3.next();
emp.removeLineFromFile(lineData);
}
else{
System.out.println(" File not exists so please Login first ");
}
break;
case 5:
if (file.exists()) {
file.createNewFile();
emp.PayEmployees();
}
else{
System.out.println(" File not exists so please Login first ");
}
break;
case 0:
System.out.println ( " Exit From System ") ;
emp.ExitSystem();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
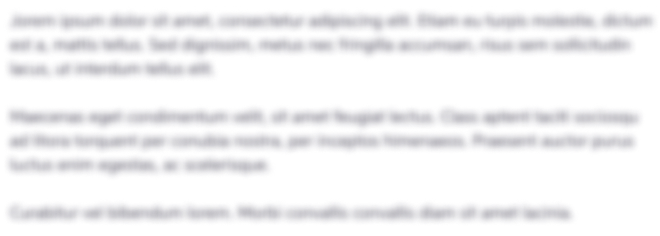
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started