Question
*Java coding DESCRIPTION You have to design an e-commerce shopping cart. These require classes Item, Electronics, Food, Dress, Cart and Main. Another class Test (Test.java)
*Java coding
DESCRIPTION You have to design an e-commerce shopping cart. These require classes Item, Electronics, Food, Dress, Cart and Main. Another class Test (Test.java) will be provided to you in Blackboard. This class will contain main() method and help you test your own code. You do not need to upload Test.java.
ITEM CLASS Your Item class should contain:
Attributes (protected)
String name - name of the Item
double price - price of the item
Methods (public)
void setName(String n) - sets the name of Item
void setPrice(double p) - sets the price of Item
String getName() - retrieves name
double getPrice() - retrieves price
String formattedOutput() returns a string containing detail of the Item as "Name,price" (without the quotes, no space only comma separating the values, for example: Apple,1.00
Constructors
Item(String n, double p) - constructor, sets name=n & price=p.
Item() - default constructor, sets name="" & price=0
ELECTRONICS CLASS
Your Electronics class inherits from Item. It should have following:
Attributes (private)
String model
int year
Methods (public)
void setModel(String m) - sets the model of Electronics.
void setYear(int year) - sets the manufacturing year of Electronics. If year<0, it is considered an invalid input and ignored (no update)
String getModel() - retrieves model.
int getYear() - retrieves manufacturing year.
String formattedOutput() overrides method from Item class and returns a string containing detail of the Electronic as "Name,price,model,makeYear" (without the quotes, no space only comma separating the values, for example: Probook,HP,2018,590.00
Constructors
Electronics(String n, double p, String md, int my) - If year<0, set it to 0.
Electronics() - default constructor, sets String attributes to "" and int/double to 0/0.00.
FOOD CLASS
Your Food class inherits from Item. It should have following:
Attributes (private)
double weight
String weightUnit
Methods (public)
void setWeightUnit(String wu) - sets the weightUnit of Food.
void setWeight(double wt) - sets the weight of Food. If w<0, no update is required.
String getWeightUnit() - retrieves weightUnit.
double getWeight() - retrieves weight.
String formattedOutput() overrides method from Item class and returns a string containing detail of the Food as "Name,prince,weight,weightUnit" (without the quotes, no space only comma separating the values, for example: Greens,1,lb,2.49
Constructors
Food(String n, double p, double w, String wu) - If w<0, set it to 0.
Food() - default constructor, sets String attributes to "" and int/double to 0/0.00.
DRESS CLASS
Your Dress class inherits from Item. It should have following:
Attributes (private)
String size
String fabric
Methods (public)
Constructor with parameters.
Additional set/get functions for new attributes.
String formattedOutput() overrides method from Item class and returns a string containing detail of the Food as "Name,price,size,fabric" (without the quotes, no space only comma separating the values, for example: Shirt,Small,cotton,9.49
Constructors
Dress(String n, double p, String s, String f)
Dress() - default constructor, sets String attributes to "" and int/double to 0/0.00.
CART CLASS
Your Cart class should contain:
Attributes (private)
Item items[] - Items in the cart. Size of this array is initialized in constructor.
int itemQuantities[] quantity of each item in the cart. Example: A cart has 2 apples and 1 computer. item[0] has Apple with quantity[0] =2, Item[1] has Computer with quantity[1] = 1 i.e. quantity[i] is the quantity of item[i]
int itemCount number of items (types, not quantities) at any given state. For above example if you have 2 apples and 1 Electronics in the cart, your itemCount should be 2.
double totalPrice - price of all the items in the cart
int totalQuantity - quantity of all the items in the cart. For above example, total quantity is 3.
Constructors
Cart() - sets default value cartSize to 10 and creates arrays items & itemQuantities arrays having size 10, initializes other int/double type attributes to 0/0.00.
Cart(int cartSize) - sets cartSize and creates arrays items & itemQuantities arrays having size cartSize, initializes other int/double type attributes to 0/0.00.. If cartSize is less than or equal to zero, set it to default size 10.
Methods - all methods are public (unless mentioned)
int getItemCount() - retrieves itemCount
double getTotalPrice() - retrieves totalPrice
int getTotalQuantity() - retrieves totalQuatnity
int getCartSize() - retrieves cartSize
void setCartSize(int newCartSize) - sets cartSize. It always works if newCartSize greater than current cartSize. If the newCartSize is less than current cartSize, resize only if itemCount is less than newCartSize, otherwise leave unchanged. If newCartSize is less than or equal to zero, no update is done.
void add (Item item, int addQuantity) - to add items to the cart. Make sure you can only add items if there is enough room in the cart (the quantity of each item does not matter). If an item is added (you can use searchItem method here) that is already in the cart, increase the quantity, but do not duplicate the item. If addQuantity is less than or equal to zero, no update is required.
void remove (Item item, int removeQuantity) - to remove removeQuantity number of items from the cart (if the removeQuantity is greater than quantity of items in the cart, it removes all of them). For example, you have 7 apples in the cart and removeItem(Apples,15) is called, then you remove all 7 of them (including the item from the cart), but if removeItem(Apples,5) is called, you remove 5 of them and 2 apples remain in the cart. Note that, you can only remove item if it is present in the cart (you can use searchItem method). If removeQuantity is less than or equal to zero, no update is required.
private int search (Item item) returns index of the item in the array if found, or -1 if item is not found in the cart. Compare name of the item only. This is a helper function you can use in addItem or removeItem method.
String formattedOutput() - Calls the formattedOutput() function of all items in the cart, followed by the quantity of each item type in cart, and its total price (the price should reflect the quantity). Finally, formattedOutput() should include the total number of items in the cart and the total price of all items in the cart.
MAIN CLASS
This file already has a template with empty main() method. Your Main class in Zybook should look like:
public class Main { public static void main(String[] args) { } }
BONUS (20 points)
Modify int searchItem(Item item) method in Cart class, to compare items considering all of their attributes. You have to use instance of operator for this. You are not allowed to use any new method(s) for this task. For example, if the name matches for Electronics class object, you need to check price, model and year. However, if its a Dress class object, you need to check if price, size and fabric matches. For example: without implementing bonus, following two items would be taken as same: Probook,HP,2008,190.00 and Probook,HP,2019,690.00, but would be different with the bonus version of searchItem.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
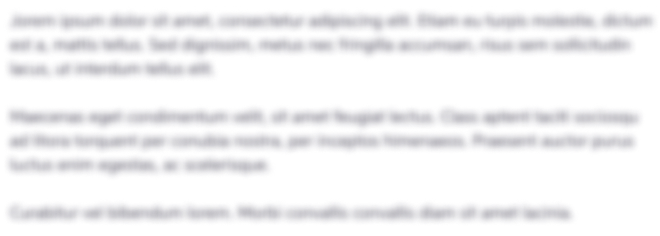
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started