Question
JAVA Create a class called ParkedCar . The class has the following: Attributes: owner name e.g. Bob Smith car make e.g. Porsche cayenne model year
JAVA
Create a class called ParkedCar. The class has the following:
Attributes:
owner name e.g. Bob Smith
car make e.g. Porsche cayenne
model year e.g. 2015
car license plate number e.g. 1A2B3C
number of minutes parked e.g. 123
Provide a constructor, the constructor will take parameters in the following order (owner name ,make, model year, license plate number, minutes parked)
The following restrictions should be implemented in the constructor:
- Owner name, car make, and license plate number should not be null, if the provided value was null the value will be rejected and an error message such as car make cannot be null or car license plate number cannot be null will be produced.
- Model year cannot be earlier than 1900 or later than 2020 exclusive otherwise the value will be rejected and an error message model year cannot be earlier than 1900 or model year cannot be later than 2020 will be produced.
-
HINT: the current year can be obtained from the system by implementing the following :
-
- add the statement import java.util.Calendar; before the class header
- assign the following statement to your current year variable
-
CURRENT_YEAR = Calendar.getInstance().get(Calendar.YEAR);
Alternatively you can assign a number such as 2020 to your current year variable
-
number of minutes parked will not be negative value or 0 otherwise the value will be rejected and an error message minutes parked should be greater than 0 will be produced
-
Provide appropriately named accessor for the fields:
Owner name
Car make
Model year
Car license plate number
Number of minutes parked
-
Provide appropriately named mutators for the fields:
Owner name
Car make
Model year
Car license plate number
Number of minutes parked
The mutators will apply the same validation mentioned in the constructor section above
-
Provide a method displayDetails(), the method will display the details of the ParkedCar object in the following format:
Owner name: Adam White
Car make: Mazda
Car model year: 2010
Car license plate number: 1A2B3C
Number of minutes parked: 95
Step by Step Solution
There are 3 Steps involved in it
Step: 1
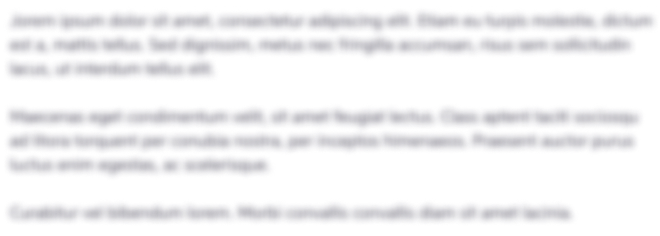
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started