Question
Java: Cryptogram project: make a cryption/decryption program that reads from a file: instructions, then the client as well as the .txt file which is to
Java: Cryptogram project: make a cryption/decryption program that reads from a file: instructions, then the client as well as the .txt file which is to be crypted/decrypted at the bottum.
. For this project, encrypting consists of translating each character into another character. For instance, if we consider the English alphabet, including characters a through z, each character is going to be encrypted based on its mapping given by the cryptogram code.
To support this concept, you need to implement Cryptogram.java class; the client class called CryptogramClient.java where the main method is located is fully implemented. See the UML Diagram and the sample run below.
Cryptogram class has four instant variables:
ALPHABET an array of 26 chars (lowercase letters a to z) which is final
letterCounters - an array of ints, that is the same size as the ALPHABET array and will contain the frequencies of each letter in the processed file. The constructor creates the array, so initially the counters will be all 0
totalNumberOfLetters int holding the sum of all letters in the file
cryptCode an array of chars, that is the same size as the ALPHABET array and contains the same values as the ALPHABET array but arranged randomly. This array needs to be generated by the setCryptCode method which would be called by the constructor. For example, we could have:
ALPHABET | cryptCode |
a | u |
b | p |
c | h |
d | a |
e | s |
f | x |
g | z |
h | b |
i | j |
To encrypt a phrase, a new String is produced where each letter in the phrase is replaced by the corresponding letter in the cryptCode. For example, the word Caged would be encrypted as huzsa(please note that the algorithm converts all the letters to lower case first). To decrypt an encrypted phrase, a new String would be produced where the letters in the encrypted phrase are replaced by the corresponding letters in the original alphabet. For example, the encrypted word xssa would be decrypted as feed.
Sample Run:
Alphabet: a b c d e f g h i j k l m n o p q r s t u v w x y z
Cryptcode: d l a p r k y w v g h s e j i z f o u n t b m q c x
The original line is "I would rather be a 'could be'"
The encrypted phrase is "v mitsp odnwro lr d 'aitsp lr'"
The decrypted phrase is "i would rather be a 'could be'"
The original line is "if I cannot be an 'are', "
The encrypted phrase is "vk v adjjin lr dj 'dor', "
The decrypted phrase is "if i cannot be an 'are', "
If we have 26 different characters in the original alphabet, then we will have 26 different characters in the cryptCode, furthermore the encrypted alphabet (the cryptCode) is randomly generated. Note that none of the letters would map to itself, and each mapping is unique.
Cryptogram constructor:
creates this.letterCounters object
sets this.totalNumberOfLetters to 0
sets this.totalNumberOfCharacters to 0
creates this.cryptCode object
calls setCryptCode method
setCryptCode method should follow this algorithm:
copy all the elements from the ALPHABET array to the cryptCode array at randomly generated positions that are different from the positions of the letters in ALPHABET
encrypt method should:
utilize private findLetterInAlphabet (this private method should look very similar to the sequential search algorithm for sorted data )
utilize StringBuilder
update the letterCounters, the running sum of letters in the file stored in totalNumberOfLetters and the running sum of characters in the file stored in totalNumberOfCharacters
decrypt method should:
utilize private findLetterInCryptCode method (this private method should look very similar to the sequential search algorithm for unsorted data see findWinners method in lecture notes)
utilize StringBuilder
Note that in the main method a Cryptogram object is created, a loop is set-up that reads from a file one line at time. As the line is read the program encrypts the read sentence, outputs the encrypted sentence, then decrypts it, and outputs the decrypted sentence, which should be identical to the original sentence (except for case).
Once the file has been processed your program should output the file statistics that show frequency of each letter in the file, shown as count and as percentage. encrypt method should use letterCounters array to keep track of number of occurrences of each letter.
The CryptogramClient.java is done, so when finished, submit only Cryptogram.java class for grading.
Here is the file to be processed by the cryptogram: wish.txt:
I would rather be a 'could be' if I cannot be an 'are', because a could be is a maybe who is reaching for a star. I would rather be a 'has been' than a 'might-have-been' by far; for a might-have-been has never been, but a 'has' was once an are. - Milton Berle
###############################################
and here is the CryptogramClient.java which is already complete:
// Cryptogram Client // YOUR NAME // 11/1/2017
import java.util.*; import java.io.*;
public class CryptogramClient { public static void main(String[] args) throws IOException { Cryptogram cryptogram = new Cryptogram(); System.out.println(cryptogram); // print alphabet and substitution code
Scanner fromFile = new Scanner(new File("wish.txt")); while (fromFile.hasNext()) { String phrase = fromFile.nextLine(); System.out.println(" The original line is \t \"" + phrase + "\"");
String code = cryptogram.encrypt(phrase);
System.out.println("The encrypted phrase is \t \"" + code + "\"");
String plainText = cryptogram.decrypt(code);
System.out.println("The decrypted phrase is \t \"" + plainText + "\""); } System.out.println(); cryptogram.displayStatistics(); } }
UML Diagram cCryptogram chart] char[] intl] ALPHABET e cryptCode e letterCounters e totalNumberOfLetters int e totalNumberOfCharacters int NOTFOUND - mob Cryptogram() in setCryptCode() m findLetterinAlphabet(char) void int String encrypt(String) mfindLetterinCryptCode(char) mio decrypt(String) String void String displayStatistics() mtoString) createx CCryptogramClient mmain(String[]) voidStep by Step Solution
There are 3 Steps involved in it
Step: 1
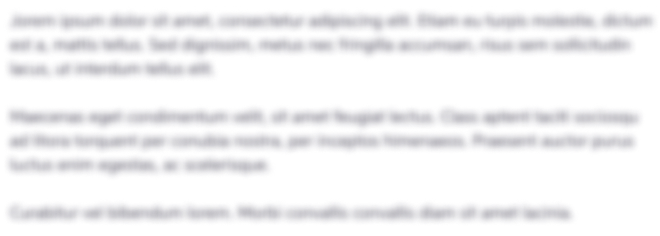
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started