Java - Data structures Consider an array of length n that contains unique integers between 1 and n+1. For example, an array A1 of length
Java - Data structures
Consider an array of length n that contains unique integers between 1 and n+1. For example, an array A1 of length 5 might contain the integers 3, 6, 5, 1, and 4. In an array of this form, one number between 1 and n+1 will be missing. In A1, the integer 2 is missing from the array. As another example, consider the array A2 that contain the integers 4, 7, 5, 2, 6, and 1. A2 has size 6, contains integers in the range of 1 to 7. In A2, the integer 3 is missing from this array.
Write a Java method
static int findMissing(int [] a)
that reads the array and returns the number not present in the array. The running time of the algorithm should be O(n).
For A1=[3,6,5,1,4], findMissing(A1) returns 2. For A2=[4,7,5,2,6,1], findMissing(A2) returns 3.
Hint: Use the formula: 1 + 2 + 3 + + n = n(n+1)/2
SortArray.Java
public class ArraySort { public static
private static
// move pivot to next-to-last position in array swap(a, mid, last - 1); int pivotIndex = last - 1; T pivot = a[pivotIndex];
// determine subarrays Smaller = a[first..endSmaller] // and Larger = a[endSmaller+1..last-1] // such that entries in Smaller are <= pivot and // entries in Larger are >= pivot; initially, these subarrays are empty int indexFromLeft = first + 1; int indexFromRight = last - 2; boolean done = false; while (!done) { // starting at beginning of array, leave entries that are < pivot; // locate first entry that is >= pivot; you will find one, // since last entry is >= pivot while (a[indexFromLeft].compareTo(pivot) < 0) indexFromLeft++; // starting at end of array, leave entries that are > pivot; // locate first entry that is <= pivot; you will find one, // since first entry is <= pivot while (a[indexFromRight].compareTo(pivot) > 0) indexFromRight--; if (indexFromLeft < indexFromRight) { swap(a, indexFromLeft, indexFromRight); indexFromLeft++; indexFromRight--; } else done = true; } // end while // place pivot between Smaller and Larger subarrays swap(a, pivotIndex, indexFromLeft); pivotIndex = indexFromLeft; return pivotIndex; } public static
Step by Step Solution
There are 3 Steps involved in it
Step: 1
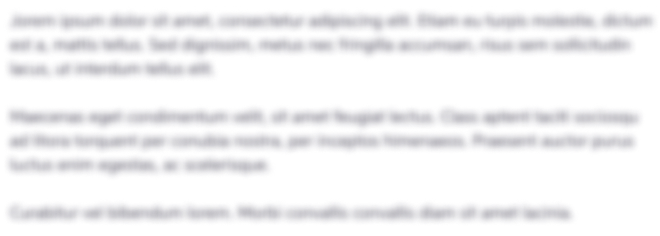
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started