Question
JAVA Data Structures Help, guaranteed thumbs up! public class Course implements CourseADT { // TODO: add appropriate instance variables and complete constructor(s). // Complete each
JAVA Data Structures Help, guaranteed thumbs up!
public class Course implements CourseADT {
// TODO: add appropriate instance variables and complete constructor(s). // Complete each method and add other methods as necessary
Course(String courseName, int section) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } @Override public boolean enroll(Student student) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public boolean withdraw(Student student) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public String enrollmentList() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } public String toString() { throw new UnsupportedOperationException("Not supported yet."); } } /////////////////////////////////////////////////////////////////////////////////////////////////////////////
public interface CourseADT { /** * Enroll a student in this course * @param student * @return true if successful */ boolean enroll(Student student); /** * Withdraw the specified student form this course. * @param student * @return true if successful */ boolean withdraw(Student student); /** * Return a list of courses plus the students enrolled in each. * @return */ String enrollmentList(); } /////////////////////////////////////////////////////////////////////////////////////////////////////
import DSLib.*;
/** * Lab1 - Bag Client for School Registrar * * */ public class Lab1 {
/** * @param args the command line arguments */ public static void main(String[] args) { // Create a bag of Courses BagADT
// Create some courses and add to the bag Course history101_1 = new Course("History101", 1); Course history101_2 = new Course("History101", 2); Course math101_1 = new Course("Math101", 1); Course programming101_1 = new Course("Programming101", 1);
// Enroll some students history101_1.enroll(new Student("Tom", 123)); history101_1.enroll(new Student("Dick", 234)); history101_2.enroll(new Student("Harry", 345)); Student alice456 = new Student("Alice", 456); // Soon to be withdrawn history101_2.enroll(alice456); history101_2.enroll(new Student("Ted", 567)); history101_2.enroll(new Student("Alice", 678)); math101_1.enroll(new Student("Tom", 123)); // Try to enroll Tom a second time boolean result = math101_1.enroll(new Student("Tom", 123)); System.out.println("A second student enrollment was " + result);
programming101_1.enroll(new Student("Alice", 678));
// Create a list of courses courses.add(history101_1); courses.add(history101_2); courses.add(math101_1); courses.add(programming101_1);
// Print list of courses System.out.println("Course List"); Course[] courseList = (Course[])(courses.toArray()); for (Course course : courseList) { System.out.println(course); }
// Print list of courses + enrolled students System.out.println(" Course Enrollment"); for (Course course : courseList) { System.out.println(course); // Name+course section System.out.println(course.enrollmentList()); }
// Drop a student and reprint enrollment history101_2.withdraw(alice456);
System.out.println(" Course Enrollment"); for (Course course : courseList) { System.out.println(course); // Name+course section System.out.println(course.enrollmentList()); } }
}
/////////////////////////////////////////////////////////////////////////////////////////////
public class Student {
Student(String studentName, int id) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } @Override public String toString() { throw new UnsupportedOperationException("Not supported yet."); } // Need to compare this student to another public boolean equals(Student aStudent) { throw new UnsupportedOperationException("Not supported yet."); }
///////////////////////////////////////////////////////////////////////////////////////////////////////
package DSLib; import java.util.ArrayList; public class Bagimplements BagADT { private ArrayList bag = new ArrayList(); public int size() { return this.bag.size(); } public boolean isFull() { return false; } public boolean isEmpty() { return this.bag.isEmpty(); } public boolean add(T newEntry) { return this.bag.add(newEntry); } public T remove() { return removeRandom(); } public boolean remove(Object anEntry) { return this.bag.remove(anEntry); } public T removeRandom() { int size = this.bag.size(); if (size == 0) return null; int index = (int)(Math.random() * size); return this.bag.remove(index); } public void clear() { this.bag = new ArrayList(); } public int getFrequencyOf(T anEntry) { int counter = 0; for (T item : this.bag) { if (anEntry.equals(item)) counter++; } return counter; } public boolean contains(T anEntry) { for (T item : this.bag) { if (anEntry.equals(item)) return true; } return false; } public Object[] toArray() { T[] t = this.bag.toArray((T[])new Object[this.bag.size()]); return (Object[])t; } }
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package DSLib; public interface BagADTLab 1 - Bag Client A school offers a number of courses which students can enroll in. Additionally, students may take multiple courses. For example, Tom, Dick and Harry are students. Tom and Dick enroll in History 101, Dick and Harry are enrolled in Programming 101. Thus, the school's Registrar has two courses to manage. One way of tackling this problem is using the BagADT. The Registrar maintains a 'bag' of courses and each course maintains a 'bag' of students. Our Registrar needs a program to perform the following: 1. Create a pool of course offerings. 2. Add students to specific course. 3. Drop students from a specific course. 4. Prepare a report of course offerings. 5. Prepare a report of student enrollment (by course). Use the standard problem solving methodology that has been described. Examine the description and determine which classes would be most appropriate. As a start, a prototype Netbeans project has been provided that also contains a fully working implementation of the BagADT as a 'library'. As you examine the starter main class, take note of the import statement. This class already creates a pool of course offerings and also serves as a test program - without modification. Examine the code. It can provide insight as to handle the other facets of this lab exercise. The immediate demands are minimal, therefore, you need not design your classes to contain all the data that a typical system would require. For example, a Course class would only need to identify the course name and section; a Student class need only be identified by name and ID. NOTE: as there may be similar names, a student is uniquely identified by ID. Start with the Student class. A constructor header is provided, but you need to provide definitions of instance variables and any other methods required to support the program. For example, a toString method. Because you may be comparing one student to another, the equals method need to be implemented. The Course class is somewhat more complex. Multiple students may be enrolled in the same course, however, no student can be enrolled a second time. In support of Step 2, it contains a list of all enrolled students. It must also be able to remove a student from that course (Step 3). Additionally, it must be able to return a string of all enrolled students in that course (in support of Step 5). If necessary, import the Bag library. When tested, your output should appear as follows: Course List History101 Section 1 History101 Section 2 Math101- Section 1 Programming101- Section 1 Course Enrollment History101 Section 1 Tom (123) Dick (234) History101 Section 2 Harry (345) Alice (456) Ted (567) Alice (678) Math101- Section 1 Tom (123) Programming101- Section 1 Alice (678) Course Enrollment History101 Section 1 Tom (123) Dick (234) History101 Section 2 Harry (345) Ted (567) Alice (678) Math101- Section 1 Tom (123) Programming101- Section 1 Alice (678) Lab 1 - Bag Client A school offers a number of courses which students can enroll in. Additionally, students may take multiple courses. For example, Tom, Dick and Harry are students. Tom and Dick enroll in History 101, Dick and Harry are enrolled in Programming 101. Thus, the school's Registrar has two courses to manage. One way of tackling this problem is using the BagADT. The Registrar maintains a 'bag' of courses and each course maintains a 'bag' of students. Our Registrar needs a program to perform the following: 1. Create a pool of course offerings. 2. Add students to specific course. 3. Drop students from a specific course. 4. Prepare a report of course offerings. 5. Prepare a report of student enrollment (by course). Use the standard problem solving methodology that has been described. Examine the description and determine which classes would be most appropriate. As a start, a prototype Netbeans project has been provided that also contains a fully working implementation of the BagADT as a 'library'. As you examine the starter main class, take note of the import statement. This class already creates a pool of course offerings and also serves as a test program - without modification. Examine the code. It can provide insight as to handle the other facets of this lab exercise. The immediate demands are minimal, therefore, you need not design your classes to contain all the data that a typical system would require. For example, a Course class would only need to identify the course name and section; a Student class need only be identified by name and ID. NOTE: as there may be similar names, a student is uniquely identified by ID. Start with the Student class. A constructor header is provided, but you need to provide definitions of instance variables and any other methods required to support the program. For example, a toString method. Because you may be comparing one student to another, the equals method need to be implemented. The Course class is somewhat more complex. Multiple students may be enrolled in the same course, however, no student can be enrolled a second time. In support of Step 2, it contains a list of all enrolled students. It must also be able to remove a student from that course (Step 3). Additionally, it must be able to return a string of all enrolled students in that course (in support of Step 5). If necessary, import the Bag library. When tested, your output should appear as follows: Course List History101 Section 1 History101 Section 2 Math101- Section 1 Programming101- Section 1 Course Enrollment History101 Section 1 Tom (123) Dick (234) History101 Section 2 Harry (345) Alice (456) Ted (567) Alice (678) Math101- Section 1 Tom (123) Programming101- Section 1 Alice (678) Course Enrollment History101 Section 1 Tom (123) Dick (234) History101 Section 2 Harry (345) Ted (567) Alice (678) Math101- Section 1 Tom (123) Programming101- Section 1 Alice (678){ int size(); boolean isFull(); boolean isEmpty(); boolean add(T paramT); T remove(); boolean remove(Object paramObject); T removeRandom(); void clear(); int getFrequencyOf(T paramT); boolean contains(T paramT); Object[] toArray(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
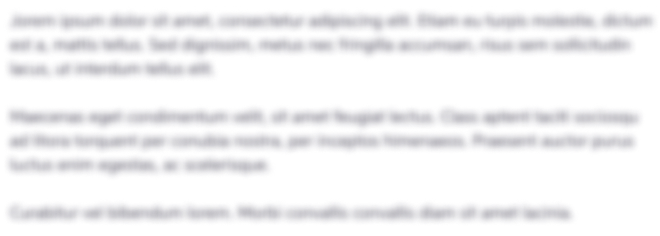
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started