Question
Java Exceptions to Create (Default message should include information relating to the exception): IllegallyParkedException NegativeValueException NotParkableException LotFullException VehicleMissingException ParkingSpaceOccupiedException InvalidColorException InvalidBrandException ---------------------------------------------- UML Documents Required:
Java
Exceptions to Create (Default message should include information relating to the exception):
IllegallyParkedException
NegativeValueException
NotParkableException
LotFullException
VehicleMissingException
ParkingSpaceOccupiedException
InvalidColorException InvalidBrandException
----------------------------------------------
UML Documents Required:
UML Hierarchy Diagram
UML Class Diagrams for each Class (Auto, Bicycle, Brand, Color, Parkable, ParkingLot, ParkingSpace, ValidParkingObject, Vehicle)
--------------------------------------------
Grading Breakdown:
Each Exception: 5 points each (40 points total)
UML Hierarchy Diagram (15 points)
UML Class Diagrams 5 points each (45 points total)
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * A Bicycle Object * @author kerlin */ public class Bicycle extends Vehicle implements Parkable { private String serialNumber; private Brand brand; private boolean locked; /** * Default Bicycle */ public Bicycle() { super(); serialNumber = "N/A"; brand = Brand.Unknown; locked = false; } /** * Full parameter Bicycle constructor * @param inSerialNumber String with Serial Number Data * @param inBrand String with Brand Data * @param inLocked boolean: True if bike is locked, False if bike is not locked */ public Bicycle(String inSerialNumber, String inBrand, boolean inLocked, int inSpeed, int inPass) { super(inSpeed,inPass); setSerialNumber(inSerialNumber); setBrand(inBrand); locked = inLocked; } @Override public boolean park() { setLocked(true); return true; } /** * Describes the Bicycle * @return a String with the Bicycle's data */ @Override public String toString() { return "Bicycle{" + "serialNumber=" + serialNumber + ", brand=" + brand + ", locked=" + locked + super.toString() + '}'; } /** * The Bicycle's serial number * @return a String containing the Bicycle's serial number */ public String getSerialNumber() { return serialNumber; } /** * Change the Bicycle's serial number * @param inSerialNumber a String to set as the Bicycle's serial number */ public void setSerialNumber(String inSerialNumber) { if ( inSerialNumber.length() > 2 && inSerialNumber.length() /** * Get the type of Bicycle * @return a String with the brand of the Bicycle */ public Brand getBrand() { return brand; } /** * Set the type of Bicycle * @param inBrand a String with the brand info for the Bicycle */ public void setBrand(String inBrand) { Brand[] validBrands = Brand.values(); boolean foundMatch = false; //Check the elements/values in the array for (int index = 0; index } /** * Check if the Bicycle is locked or not * @return a boolean with data on if the Bicycle is locked (true) or not (false) */ public boolean isLocked() { return locked; } /** * Set the locked status of the Bicycle * @param lock a boolean which locks (true) or unlocks (false) a Bicycle */ public void setLocked(boolean lock) { locked = lock; } public boolean equals(Object otherObject) { if (!(otherObject instanceof Bicycle)) { return false; //Since it's not even a Bicycle! } else { if (((Bicycle) otherObject).getSerialNumber().equals(getSerialNumber()) && ((Bicycle) otherObject).getBrand().equals(getBrand()) && ((Bicycle) otherObject).isLocked() == (isLocked())) { return true; } else { return false; // At least 1 attribute is different } } } @Override public boolean unpark() { setLocked(false); return true; } }
import java.util.Random; /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Bike Brands * @author kerlin */ public enum Brand { Unknown, Schwinn, Huffy, Aero; public static Brand randomBrand() { Brand[] myBrands = Brand.values(); Random gen = new Random(); int index = gen.nextInt((myBrands.length -1)) + 1; return myBrands[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index
import java.util.Random; /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Colors * @author kerlin */ public enum Color { Unknown, Red, Orange, Pink, Black; public static Color randomColor() { Color[] myColors = Color.values(); Random gen = new Random(); int index = gen.nextInt((myColors.length -1)) + 1; return myColors[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; import java.util.Random; import java.util.Scanner; /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * This code tests Auto (and eventually you should test Bicycle and ParkingSpace) * @author kerlin */ public class Driver { /** * @param args the command line arguments */ public static void main(String[] args) throws FileNotFoundException { } }
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Things that can Park * @author kerlin */ public interface Parkable { /** * Method that parks a Parkable vehicle * @return true if vehicle is correctly parked */ boolean park(); /** * Method that unparks a Parkable vehicle * @return true if vehicle has been correctly unparked */ boolean unpark(); }
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * A Parking Lot Object * @author kerlin */ public class ParkingLot { private ParkingSpace[] spaces; private double percentFull; /** * Constructor for a Parking Lot * @param numAuto number of Auto ParkingSpaces * @param numBike number of Bicycle ParkingSpaces */ public ParkingLot(int numAuto, int numBike) { spaces = new ParkingSpace[numAuto + numBike]; for (int i = 0; i @Override public String toString() { int count = 0; for (int i = 0; i /** * Try to park a given Vehicle * @param inBike Auto to park * @return True if we could park, false otherwise */ public boolean park(Bicycle inBike) { for(int i = 0; i /** * Try to find and return a parked vehicle * @param target Plate or Serial Number to look for * @return The parked vehicle OR a null if no such vehicle could be found */ public Object find(String target) { for(int i = 0; i
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * A Parking Space object * @author kerlin */ public class ParkingSpace { private boolean occupied; private int number; private ValidParkingObject type; private Object vehicle; private static int numberOfSpaces = 0; /** * Gets the vehicle parked in the ParkingSpace (DOES NOT REMOVE VEHICLE) * @return the vehicle parked in the ParkingSpace */ public Object getVehicle() { return vehicle; } /** * Default Parking Space */ public ParkingSpace() { occupied = false; number = numberOfSpaces; numberOfSpaces++; type = ValidParkingObject.Unknown; vehicle = null; } /** * Full parameter ParkingSpace constructor * @param inType String noting the type of object which can park in this Parking Space */ public ParkingSpace(String inType) { occupied = false; number = numberOfSpaces; numberOfSpaces++; setType(inType); vehicle = null; } /** * Describes the Parking Space * @return a String with the Parking Space's data */ @Override public String toString() { return "ParkingSpace{" + "occupied=" + occupied + ", number=" + number + ", type=" + type + ", vehicle=" + vehicle + '}'; } /** * Try to park an Auto in this Parking Space * @param inAuto the Auto try to park here * @return a boolean noting if the Auto was able to park (true) or not (false) */ public boolean parkAuto(Auto inAuto) { if (isOccupied()) { return false; } else if (getType().equals(ValidParkingObject.Auto)) { occupied = true; vehicle = inAuto; return true; } else return false; } /** * Try to park a Bicycle in this Parking Space * @param inBike the Bicycle to try parking here * @return a boolean noting if the Bicycle was able to park (true) or not (false) */ public boolean parkBicycle (Bicycle inBike) { if (isOccupied()) { return false; } else if (getType().equals(ValidParkingObject.Bicycle)) { vehicle = inBike; occupied = true; return true; } else return false; } /** * Unparks a vehicle from the ParkingSpace * @return the Vehicle in the ParkingSpace */ public Object unpark() { occupied = false; return vehicle; } /** * Check if the Parking Space is occupied or not * @return a boolean which is true if the Parking Space is in use and false if the Parking Space is not in use */ public boolean isOccupied() { return occupied; } /** * Get the ParkingSpace number * @return an int with the ParkingSpace's number */ public int getNumber() { return number; } /** * Get the type of ParkingSpace * @return a String denoting what type of Object is allowed to park in this ParkingSpace */ public ValidParkingObject getType() { return type; } /** * Set the type of Parking Space * @param inType a String to note what type of Object is allowed to park in this Parking Space */ public void setType(String inType) { ValidParkingObject[] validTypes = ValidParkingObject.values(); boolean foundMatch = false; //Check the elements/values in the array for (int index = 0; index } public boolean equals(Object otherObject) { if (!(otherObject instanceof ParkingSpace)) { return false; //Since it's not even a ParkingSpace! } else { if (((ParkingSpace) otherObject).getNumber() == (getNumber()) && ((ParkingSpace) otherObject).getType().equals(getType()) && ((ParkingSpace) otherObject).isOccupied() == (isOccupied())) { return true; } else { return false; // At least 1 attribute is different } } } }
import java.util.Random; /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * * @author kerlin */ public enum ValidParkingObject { Unknown, Auto, Bicycle; public static ValidParkingObject randomValidParkingObject() { ValidParkingObject[] myValidParkingObjects = ValidParkingObject.values(); Random gen = new Random(); int index = gen.nextInt((myValidParkingObjects.length -1)) + 1; return myValidParkingObjects[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * A Vehicle object * @author kerlin */ public class Vehicle { private int speed; private int pass; public Vehicle() { speed = 0; pass = 0; } public Vehicle(int speed, int pass) { setSpeed(speed); setPass(pass); } @Override public String toString() { return "Vehicle{" + "speed=" + speed + ", pass=" + pass + '}'; } public int getSpeed() { return speed; } public void setSpeed(int inSpeed) { if (inSpeed > 0) speed = inSpeed; else speed = 0; } public int getPass() { return pass; } public void setPass(int inPass) { if (inPass > 0) pass = inPass; else pass = 0; } }
Bicycle%27%2%ABC0%true Bicycle%14%2%ABC1%false Bicycle%5%2%ABC2%false Bicycle%11%2%ABC3%true Auto%8%7%ABC4%true Auto%95%7%ABC5%false Bicycle%11%2%ABC6%true Auto%56%7%ABC7%true Auto%0%7%ABC8%false Auto%0%7%ABC9%false Bicycle%0%2%ABC10%false Bicycle%20%2%ABC11%false Bicycle%21%2%ABC12%false Auto%21%7%ABC13%false Auto%58%7%ABC14%true Auto%61%7%ABC15%false Bicycle%29%2%ABC16%false Bicycle%10%2%ABC17%false Auto%78%7%ABC18%false Auto%22%7%ABC19%true Auto%31%7%ABC20%true Auto%22%7%ABC21%false Bicycle%15%2%ABC22%true Bicycle%18%2%ABC23%false Bicycle%12%2%ABC24%true Bicycle%0%2%ABC25%true Bicycle%18%2%ABC26%false Bicycle%4%2%ABC27%false Bicycle%7%2%ABC28%false Auto%2%7%ABC29%true Auto%89%7%ABC30%false Bicycle%23%2%ABC31%false Bicycle%0%2%ABC32%false Auto%87%7%ABC33%false Bicycle%29%2%ABC34%true Auto%86%7%ABC35%true Auto%14%7%ABC36%true Auto%14%7%ABC37%true Auto%32%7%ABC38%true Auto%51%7%ABC39%false Bicycle%4%2%ABC40%false Auto%2%7%ABC41%false Bicycle%11%2%ABC42%false Bicycle%11%2%ABC43%true Auto%72%7%ABC44%true Auto%81%7%ABC45%false Auto%66%7%ABC46%true Bicycle%23%2%ABC47%false Bicycle%21%2%ABC48%true Bicycle%17%2%ABC49%true Auto%1%7%ABC50%false Bicycle%24%2%ABC51%true Auto%57%7%ABC52%true Auto%94%7%ABC53%false Bicycle%0%2%ABC54%true Auto%93%7%ABC55%false Bicycle%11%2%ABC56%true Bicycle%12%2%ABC57%true Bicycle%21%2%ABC58%true Auto%64%7%ABC59%false Auto%57%7%ABC60%true Bicycle%10%2%ABC61%false Auto%15%7%ABC62%true Bicycle%8%2%ABC63%true Auto%55%7%ABC64%true Bicycle%7%2%ABC65%false Auto%48%7%ABC66%true Auto%50%7%ABC67%true Auto%34%7%ABC68%false Auto%95%7%ABC69%false Auto%88%7%ABC70%false Bicycle%5%2%ABC71%false Auto%9%7%ABC72%true Auto%90%7%ABC73%true Auto%92%7%ABC74%false Bicycle%0%2%ABC75%false Bicycle%27%2%ABC76%false Auto%9%7%ABC77%true Auto%93%7%ABC78%false Bicycle%5%2%ABC79%true Auto%18%7%ABC80%false Bicycle%20%2%ABC81%true Auto%23%7%ABC82%false Bicycle%14%2%ABC83%false Bicycle%11%2%ABC84%false Auto%14%7%ABC85%true Auto%31%7%ABC86%true Bicycle%7%2%ABC87%false Auto%1%7%ABC88%true Auto%50%7%ABC89%true Auto%96%7%ABC90%false Bicycle%19%2%ABC91%true Auto%97%7%ABC92%true Auto%61%7%ABC93%true Bicycle%18%2%ABC94%true Bicycle%10%2%ABC95%true Bicycle%22%2%ABC96%false Bicycle%17%2%ABC97%false Auto%64%7%ABC98%false Auto%68%7%ABC99%true
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
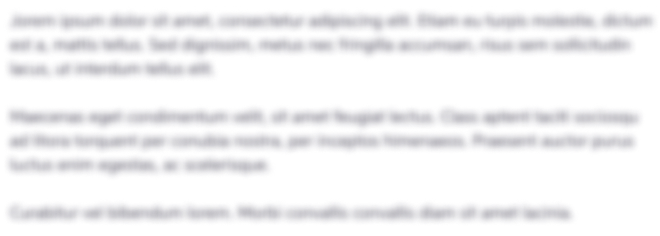
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started