Question
*****Java***** Exercise 2 Comparing Content of Objects 1. Add a public method equals(ClassA otherClass) to class classA that returns the value true if (i) this
*****Java*****
Exercise 2 Comparing Content of Objects
1. Add a public method equals(ClassA otherClass) to class classA that returns the value true if (i) this object and otherClass have the same value in variable numItems and (ii) both arrItems arrays are null or both store the same values and in the same order in the
first numItems entries; the method returns the value false otherwise. Do not try to access values from variable arrItems if arrItems is null.
2. Add a public method equals(DataTypes otherType) to class DataTypes that returns the value true if (i) all primitive instance variables in this object and otherType have the same values and (ii) variables varA in this object and otherType are equal according to method equals of class ClassA.
3. Run the java class TestEquals. Fix your code if it fails any of the 4 tests.
Here are classes:
ClassA:
public class ClassA { private final int SIZE_ARRAY = 5; public int numItems; public int[] arrItems;
public ClassA(int n, int[] arr) { numItems = n; arrItems = arr; }
public ClassA() { numItems = 0; arrItems = new int[SIZE_ARRAY]; }
}
============================
DataTypes
public class DataTypes { public int intVar; public double doubleVar; public char charVar; public boolean boolVar; public ClassA varA;
public DataTypes() { }
public DataTypes(int newIntVar, double newDoubleVar, char newCharVar, boolean newBoolVar, ClassA newVarA) { intVar = newIntVar; doubleVar = newDoubleVar; charVar = newCharVar; boolVar = newBoolVar; varA = newVarA; }
}
=============================
TestEquals
public class TestEquals {
public static void main(String[] args) { int[] arr1 = { 1, 2, 3 }; int[] arr2 = { 3, 2, 1 }; int[] arr3 = { 1, 2, 3 }; DataTypes data1 = new DataTypes(2, 2.3, 'a', false, new ClassA()); DataTypes data2 = new DataTypes(2, 2.3, 'a', false, new ClassA()); DataTypes data3 = new DataTypes(2, 2.3, 'a', false, new ClassA(3, arr1)); DataTypes data4 = new DataTypes(2, 2.3, 'a', false, new ClassA(3, arr2)); DataTypes data5 = new DataTypes(2, 2.3, 'f', true, new ClassA(3, arr2)); DataTypes data6 = new DataTypes(2, 2.3, 'a', false, new ClassA(3, arr3));
if (data1.equals(data2)) System.out.println("Test 1 passed"); else System.out.println("Test 1 failed");
if (data3.equals(data6)) System.out.println("Test 2 passed"); else System.out.println("Test 2 failed");
if (!data3.equals(data4)) System.out.println("Test 3 passed"); else System.out.println("Test 3 failed");
if (!data4.equals(data5)) System.out.println("Test 4 passed"); else System.out.println("Test 4 failed"); }
}
=================
Please finish ClassA and DataTypes
Step by Step Solution
There are 3 Steps involved in it
Step: 1
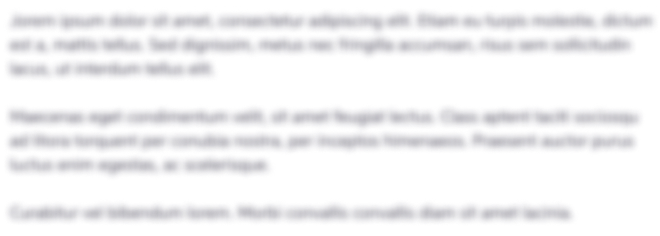
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started