Question
Java: fill in the comments in the ArrayMethodsL8b. Client and sample run included. /** Supporting methods for an integer array * @author YOUR NAME *
Java: fill in the comments in the ArrayMethodsL8b. Client and sample run included.
/** Supporting methods for an integer array * @author YOUR NAME * @version 10/27/2013 */
public class ArrayMethodsL8b { /** * instance variable array of ints */ private int [] intArray; /** * secondary constructor, * the instance variable intArray will be a copy of the array created by the user */ public ArrayMethodsL8b(int [] newArray) { setIntArray(newArray); } /** * accessor method, * returns a copy of the instance variable intArray */ public int [] getIntArray() { // instantiate array with the same length as the parameter int[] copy = new int [this.intArray.length]; for (int i = 0; i < this.intArray.length; i++) { copy[i] = this.intArray[i]; } return copy; } /** * returns the length of the instance variable intArray */ public int getLength() { return this.intArray.length; } /** * mutator method, * the instance variable intArray will be a copy of the array passed by the user */ public void setIntArray(int[] newArray) { // instantiate array with the same length as the parameter this.intArray = new int [newArray.length]; for (int i = 0; i < newArray.length; i++) { this.intArray[i] = newArray[i]; } } /** * equals method * checks if the array in this object is the same as the array in the other object * if the lengths are not the same returns false right away * otherwise compairs the elements until either the first not the equal pair is found * or there are no more elements to compare */ public boolean equals (ArrayMethodsL8b other) { boolean isEqual = true; if ( this.intArray.length != other.intArray.length ) { isEqual = false; // arrays are not the same size } else { for ( int i = 0; i < this.intArray.length && isEqual; i++ ) { if ( this.intArray[i] != other.intArray[i] ) { // found the first pair that is not the same // no need to compare any further isEqual = false; } } } return isEqual; } /** * toString method returns printable version * of the conent of intArray */ public String toString() { String returnValue = ""; for ( int i = 0; i < this.intArray.length; i++ ) { returnValue += this.intArray[i] + " "; } return returnValue += " "; } // *** BUSINESS METHODS *** // /** * "business" method that calculates product * of all the integers in the array * @return an integer - value of the product */ public int arrayProduct() { int product = this.intArray[0]; for ( int i = 1; i < this.intArray.length; i++ ) { product *= this.intArray[i]; } return product; } /** * "business" method that swaps elements if necessary * and calculates the numbers of swaps * @return an integer - the number of swaps performed */ public int calculateSwaps() { //STEP 1 // in a for loop compare elements pairwise: first with last; second with the second last; etc // if the element at the lower index is greater that the element in the higher index swap them // remember to count the number of swaps System.out.println("in calculateSwaps method - implement me"); return 0; // THIS IS A STUB } /** * "business" method that reverses the order of elements in the intArray * i.e. if the elements were 11 22 33 44 55 the method will change the order to 55 44 33 22 11 * */ public void reverse() { // STEP 2 // in a for loop change the order of elements by swapping first with last; // second with the second last; etc // System.out.println("in reverse method - implement me"); } /** * "business" method that searches intArray to see if it contains the number * * @return index of the element that is the same as the number * or -1 if not found */ public int searchForNumber(int number) { // STEP 9 // in a for loop check for an element that is the same as the number // stop the search as soon as such element is found. // IMPORTANT: no return from the loop please, have appropriate // compound boolean condition that will stop the loop when needed //
System.out.println("in searchForNumber method - implement me"); return 0; // THIS IS A STUB } }
====================================================================================================================
/** Lab8b * @author YOUR NAME * @version 10/27/2013 */ import java.util.*; public class ArrayMethodsClientL8b { public static void main( String [] args ) { // declare and initialize array of integers in ascending order int [] intNumbersSortedA = { 1, 2, 3, 4, 5, 6 }; // STEP 5 // declare and initialize an array with 10 randomly generated // numbers between 1 and 9 System.out.println("STEP 5 - implement me"); // create an ArrayMethodsL8b object - passing the intNumbersSortedA array as the argument System.out.println("--->calling secondary constructor"); ArrayMethodsL8b arr = new ArrayMethodsL8b(intNumbersSortedA); // print the content of the array System.out.println( "The elements of the array sorted in ascending order are: " + arr.toString()); // print the value of the product calculated by the method arrayProduct System.out.println( "The product of all elements in the array is: " + arr.arrayProduct() ); // calculate number of swaps System.out.println("The number of swaps was " + arr.calculateSwaps()); System.out.println("The array after swaps is: " + arr.toString()); System.out.println(); // STEP 3 // call reverse method // STEP 4 // print the content of the array // print the value of the product calculated by the method arrayProduct // calculate number of swaps // STEP 6 // call mutator method to change the array in arr object to the one that contains random numbers // STEP 7 // print the content of the array // print the value of the product calculated by the method arrayProduct // calculate number of swaps // STEP 8 // prompt the user for a number to search for // STEP 10 // call searchForNumber method and print either the position of the // searched for value or not found message } }
===================================================================
SAMPLE RUN #1 ============= --->calling secondary constructor The elements of the array sorted in ascending order are: 1 2 3 4 5 6 The product of all elements in the array is: 720 The number of swaps was 0 The array after swaps is: 1 2 3 4 5 6 --->calling reverse method The elements of the array sorted in descending order are: 6 5 4 3 2 1 The product of all elements in the array is: 720 The number of swaps was 3 The array after swaps is: 1 2 3 4 5 6 --->calling mutator method The elements of the array are: 5 5 6 8 8 6 8 5 6 1 The product of all elements in the array is: 13824000 The number of swaps was 3 The array after swaps is: 1 5 5 8 6 8 8 6 6 5 Enter the number to search for 8 The number 8 was found at index 3 SAMPLE RUN #2 ============= --->calling secondary constructor The elements of the array sorted in ascending order are: 1 2 3 4 5 6 The product of all elements in the array is: 720 The number of swaps was 0 The array after swaps is: 1 2 3 4 5 6 --->calling reverse method The elements of the array sorted in descending order are: 6 5 4 3 2 1 The product of all elements in the array is: 720 The number of swaps was 3 The array after swaps is: 1 2 3 4 5 6 --->calling mutator method The elements of the array are: 9 2 2 7 5 6 6 6 2 7 The product of all elements in the array is: 3810240 The number of swaps was 2 The array after swaps is: 7 2 2 6 5 6 7 6 2 9 Enter the number to search for 3 The number 3 was not found
Step by Step Solution
There are 3 Steps involved in it
Step: 1
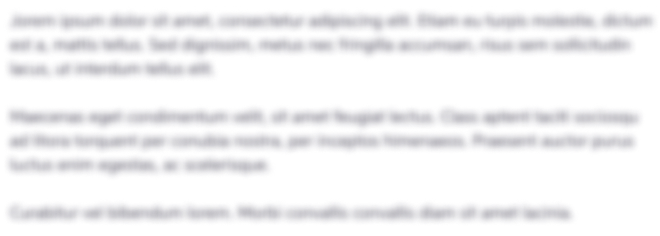
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started