Question
JAVA: finish savings account, commented with instructions. /* SavingsAccount class Anderson, Franceschi */ // 1. ***** indicate that SavingsAccount inherits from BankAccount public class SavingsAccount
JAVA: finish savings account, commented with instructions.
/* SavingsAccount class Anderson, Franceschi */
// 1. ***** indicate that SavingsAccount inherits from BankAccount public class SavingsAccount { public final double DEFAULT_RATE = .03; // 2. ****** define the private interestRate instance variable // interestRate, a double, represents an annual rate
// 3. ***** write the default constructor /** default constructor * explicitly calls the BankAccount default constructor * set interestRate to default value DEFAULT_RATE * print a message to System.out indicating that * constructor is called */
// 4. ***** write the overloaded constructor /** overloaded constructor * explicitly call BankAccount overloaded constructor * call setInterestRate method, passing startInterestRate * print a message to System.out indicating that * constructor is called * @param startBalance starting balance * @param startInterestRate starting interest rate */
// 5. ****** write the accessor method: /** accessor method for interestRate * @return interestRate */
// 6. ****** write the mutator method: /** mutator method for interestRate * @param newInterestRate new value for interestRate * newInterestRate must be >= 0.0 * if not, print an error message */
// 7. ****** write this business method: /** applyInterest method, no parameters, void return value * call deposit method, passing a month's worth of interest * remember that interestRate instance variable is annual rate * balance must also be considered */
// 8. ***** write this method /** toString method * @return String containing formatted balance and interestRate * invokes superclass toString to format balance * formats interestRate as percent using a DecimalFormat object */
// 9. ***** write this method /** equals method * @return boolean containing formatted balance and interestRate * invokes superclass equals to compare balance * and compares interestRate */
}
#################################################################
/* Teller class for exercising the SavingsAccount class * Anderson, Franceschi */
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.text.*;
public class Teller extends JFrame { private JButton changeRate, applyInterest, deposit, withdraw, accountInfo, exit; private JTextArea ledgerActivity, ledgerBalance, ledgerRate; private String lActivity, lBalance, lRate; private double rate, balance, amount; private NumberFormat currency, percent; private static SavingsAccount s1; private JPanel buttonPanel, ledgerPanel;
public Teller( ) { super( "Savings Account Ledger" ); Container cp = getContentPane( );
buttonPanel = new JPanel( ); buttonPanel.setLayout( new FlowLayout( ) );
changeRate = new JButton( "Change interest rate" ); buttonPanel.add( changeRate ); ButtonHandler bh = new ButtonHandler( ); changeRate.addActionListener( bh );
applyInterest = new JButton( "Apply interest" ); buttonPanel.add( applyInterest ); applyInterest.addActionListener( bh );
deposit = new JButton( "Deposit" ); buttonPanel.add( deposit ); deposit.addActionListener( bh );
withdraw = new JButton( "Withdraw" ); buttonPanel.add( withdraw ); withdraw.addActionListener( bh );
accountInfo = new JButton( "Display Account Information" ); buttonPanel.add( accountInfo ); accountInfo.addActionListener( bh );
exit = new JButton( "Exit" ); buttonPanel.add( exit ); exit.addActionListener( new ActionListener( ) { public void actionPerformed( ActionEvent e ) { System.exit( 0 ); } } );
ledgerPanel = new JPanel( ); ledgerPanel.setLayout( new GridLayout( 1, 3 ) );
ledgerActivity = new JTextArea( ); lActivity = "ACTIVITY "; lActivity += "Account Opened "; ledgerActivity.setText( lActivity ); ledgerActivity.setEditable( false ); ledgerPanel.add( ledgerActivity );
currency = NumberFormat.getCurrencyInstance( ); ledgerBalance = new JTextArea( ); lBalance = "BALANCE "; lBalance += currency.format( s1.getBalance( ) ) + " "; ledgerBalance.setText( lBalance ); ledgerBalance.setEditable( false ); ledgerPanel.add( ledgerBalance );
percent = NumberFormat.getPercentInstance( ); ledgerRate = new JTextArea( ); lRate = "INTEREST RATE "; lRate += percent.format( s1.getInterestRate( ) ) + " "; ledgerRate.setText( lRate ); ledgerRate.setEditable( false ); ledgerPanel.add( ledgerRate );
cp.setLayout( new GridLayout( 2, 1 ) ); cp.add( buttonPanel ); cp.add( ledgerPanel );
setSize( 300, 350 ); setVisible( true ); }
private class ButtonHandler implements ActionListener { public void actionPerformed( ActionEvent e ) { if ( e.getSource( ) == accountInfo ) { JOptionPane.showMessageDialog( null, s1, "Account Information", JOptionPane.INFORMATION_MESSAGE ); } else { if ( e.getSource( ) == changeRate ) { rate = getInput( "new interest rate (Ex: .05 is 5%)" ); s1.setInterestRate( rate ); lActivity += "Rate changed "; } else if ( e.getSource( ) == applyInterest ) { s1.applyInterest( ); lActivity += "Interest applied "; } else if ( e.getSource( ) == deposit ) { amount = getInput( "amount to deposit" ); s1.deposit( amount ); lActivity += "Deposit "; } else if ( e.getSource( ) == withdraw ) { amount = getInput( "amount to withdraw" ); s1.withdraw( amount ); lActivity += "Withdrawal "; }
lBalance += currency.format( s1.getBalance( ) ) + " "; lRate += percent.format( s1.getInterestRate( ) ) + " "; ledgerActivity.setText( lActivity ); ledgerBalance.setText( lBalance ); ledgerRate.setText( lRate ); repaint( ); } } }
public double getInput( String inputItem ) { double amount = 0.0; String input = ""; boolean badInput = true; do { input = JOptionPane.showInputDialog( "Enter the " + inputItem ); if ( input != null ) { try { amount = Double.parseDouble( input ); badInput = false; } catch ( NumberFormatException nfe ) { badInput = true; JOptionPane.showMessageDialog( null, inputItem + " must be numeric", "Input error", JOptionPane.ERROR_MESSAGE ); } } }while ( badInput == true ); return amount; }
public static void main( String [] args ) { double balance = 0.0, rate = 0.0; String input = "";
boolean badBalance = true; boolean defaultObj = false;
do { input = JOptionPane.showInputDialog( "Enter the starting balance;" + " or press return to use the default constructor" );
if ( input == null ) { System.exit( 0 ); } else if ( input.length( ) == 0 ) { s1 = new SavingsAccount( ); // use default constructor badBalance = false; defaultObj = true; } else { try { balance = Double.parseDouble( input ); if ( balance < 0.0 ) JOptionPane.showMessageDialog( null, "Balance cannot be negative" ); else badBalance = false; } catch ( NumberFormatException e ) { JOptionPane.showMessageDialog( null, "Balance must be a number" ); } } }while ( badBalance );
if ( ! defaultObj ) { boolean badRate = true; do { try { input = JOptionPane.showInputDialog( "Enter the interest rate (for example .05 is 5%)" ); if ( input != null ) { rate = Double.parseDouble( input ); if ( rate < 0.0 ) JOptionPane.showMessageDialog( null, "Interest rate cannot be negative" ); else badRate = false; } else System.exit( 0 ); } catch ( NumberFormatException e ) { JOptionPane.showMessageDialog( null, "Interest rate must be a number" ); } }while ( badRate ); s1 = new SavingsAccount( balance, rate ); System.out.println("created SavingsAccount with user's input: " + s1); }
System.out.println(" Testing equals method"); SavingsAccount testS1 = new SavingsAccount(10,.05 ); System.out.println("testS1: " + testS1); System.out.println(); SavingsAccount testS2 = new SavingsAccount( 10, .05); System.out.println("testS2: " + testS2); System.out.println(); SavingsAccount testS3 = new SavingsAccount(10,.25 ); System.out.println("testS3: " + testS3); System.out.println(); SavingsAccount testS4 = new SavingsAccount( 100, .05); System.out.println("testS4: " + testS4); System.out.println(); if (testS1.equals(testS2)) System.out.println("testS1 and testS2 are equal - CORRECT"); else System.out.println("testS1 and testS2 are not equal - INCORRECT");
if (testS1.equals(testS3)) System.out.println("testS1 and testS3 are equal - INCORRECT"); else System.out.println("testS1 and testS3 are not equal - CORRECT");
if (testS1.equals(testS4)) System.out.println("testS1 and testS4 are equal - INCORRECT"); else System.out.println("testS1 and testS4 are not equal - CORRECT");
Teller app = new Teller( ); app.setDefaultCloseOperation( JFrame.EXIT_ON_CLOSE ); } }
############################################################
/** BankAccount class, version 1 * Anderson, Franceschi * Represents a generic bank account */ import java.text.DecimalFormat;
public class BankAccount { public final DecimalFormat MONEY = new DecimalFormat( "$#,##0.00" ); private double balance;
/** Default constructor * sets balance to 0.0 */ public BankAccount( ) { this.balance = 0.0; }
/** Overloaded constructor * @param startBalance beginning balance */ public BankAccount( double startBalance ) { deposit( startBalance ); }
/** Accessor for balance * @return current account balance */ public double getBalance( ) { return this.balance; }
/** Deposit amount to account * @param amount amount to deposit; * amount must be >= 0.0 */ public void deposit( double amount ) { if ( amount >= 0.0 ) this.balance += amount; else System.out.println( "Deposit amount must be positive." ); }
/** withdraw amount from account * @param amount amount to withdraw; * amount must be >= 0.0 * amount must be <= balance */ public void withdraw( double amount ) { if ( amount >= 0.0 && amount <= this.balance ) this.balance -= amount; else System.out.println( "Withdrawal amount must be positive " + "and cannot be greater than balance" ); }
/** toString * @return the balance formatted as money */ public String toString( ) { return ( "balance is " + this.MONEY.format( this.balance ) ); } /** * compare this object with the content of the other object * @return true if they are the same */ public boolean equals(BankAccount other) { final double THRESHOLD = 0.0001; return Math.abs(this.balance - other.balance) < THRESHOLD; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
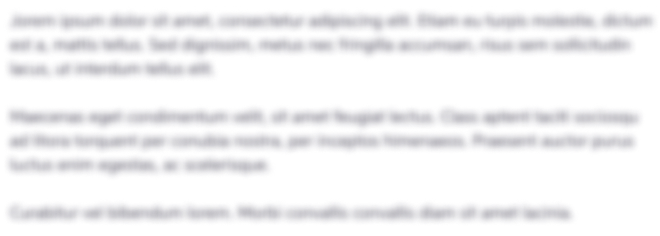
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started