Question
Java GUI Calculator The minimize, maximize and close standard icons need to display as circles (red, yellow, green) in the upper left hand corner of
Java GUI Calculator
The minimize, maximize and close standard icons need to display as circles (red, yellow, green) in the upper left hand corner of the calculator. The word calculator should be centered and the same color as the rest of the background calculator. The java icon should not display either.
Both sets of code are here now.
CalcGUI Code
import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import javax.swing.AbstractButton; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JTextField;
// the CalcGUI class defines a GUI for a calculator * public class CalcGUI extends JFrame { // create sentinal values that are triggered when an operation is being performed boolean addS = false; boolean subtractS = false; boolean divideS = false; boolean multiplyS = false; boolean decimalS = false; boolean newNumberEntered = false; // true if the last event was a digit being entered // Create a new MemoryCalc Object MemoryCalc calc = new MemoryCalc(); // Create an = button to the bottom of the GUI private JButton equals = new JButton("="); // Create a text field for the top of the GUI private JTextField topText = new JTextField("0.0"); // create an array of buttons for the center panel private JButton[] buttons = new JButton[12]; // create an array of text for the center button panel private String[] buttonNames = { "1", "2", "3", "4", "5", "6", "7", "8", "9", "0", ".", "C", }; // create buttons for the operators and add them to the east panel private JButton plus = new JButton("+"); private JButton minus = new JButton("-"); private JButton multiply = new JButton("*"); private JButton divide = new JButton("/"); private String topNumber = ""; // The string that is displayed on the calculator public CalcGUI() { // Set layout with 10 px horizontal gap and 10 px vertical gap setLayout(new BorderLayout(10, 10)); // define White (for topText) Color white = new Color(255, 255, 255); // set the top text field so that it cannot be changed by the user topText.setEditable(false); // Change topText color to white topText.setBackground(white); // change the top text field font to a bold 20pt mono font Font topTextFont = new Font("monospace", Font.BOLD, 20); topText.setFont(topTextFont); // add the top text field to the top of the GUI add(topText, BorderLayout.NORTH);
// add equals to the bottom of the calculator add(equals, BorderLayout.SOUTH); // register equals listener equals.addActionListener(new EqualsListener()); // Create a 3X3 panel for the center of the GUI // with 10px horizontal gap and 5px vertical gap JPanel pCenter = new JPanel(); pCenter.setLayout(new GridLayout(4, 3, 10, 10)); // create the numerical buttons and add them to the center panel // and add action listeners for (int i = 0; i < 10; i++) { buttons[i] = new JButton(buttonNames[i]); buttons[i].setEnabled(false); pCenter.add(buttons[i]); buttons[i].addActionListener(new DigitListener()); } // Create . and C buttons and add them to the center panel // and add action listeners buttons[10] = new JButton(buttonNames[10]); pCenter.add(buttons[10]); buttons[10].addActionListener(new DecimalListener()); buttons[11] = new JButton(buttonNames[11]); pCenter.add(buttons[11]); buttons[11].addActionListener(new CancelListener()); // Add the center panel to the frame add(pCenter, BorderLayout.CENTER); JPanel pEast = new JPanel(); pEast.setLayout(new GridLayout(4, 1, 10, 10)); pEast.add(plus); pEast.add(minus); pEast.add(multiply); pEast.add(divide); // register operator listeners plus.addActionListener(new OperatorListener()); minus.addActionListener(new OperatorListener()); multiply.addActionListener(new OperatorListener()); divide.addActionListener(new OperatorListener()); // Add the east panel to the frame add(pEast, BorderLayout.EAST); } // Add the event listener for digits 0 - 9 class DigitListener implements ActionListener { public void actionPerformed(ActionEvent e) { // add the number the user pushed to topText topNumber += ((AbstractButton) e.getSource()).getText(); topText.setText(topNumber); newNumberEntered = true; } } // Event listener for operators - +, -, *, / class OperatorListener implements ActionListener { public void actionPerformed(ActionEvent e) { // Set the currentValue to whatever is in the topText unless there // is a pending operation if (!addS && !subtractS && !divideS && !multiplyS) { calc.setCurrentValue(Double.parseDouble(topText.getText())); topNumber = ""; decimalS = false; newNumberEntered = false; } else { if (newNumberEntered == true) { // do ever calculation the user wanted equals.doClick(); newNumberEntered = false; } } for(int i = 0;i<10;i++){ buttons[i].setEnabled(true); } if (e.getSource() == plus) { addS = true; subtractS = false; divideS = false; multiplyS = false; } else if (e.getSource() == minus) { subtractS = true; addS = false; divideS = false; multiplyS = false; } else if (e.getSource() == divide) { divideS = true; addS = false; subtractS = false; multiplyS = false; } else if (e.getSource() == multiply) { multiplyS = true; addS = false; subtractS = false; divideS = false; } } } // Event listener for = class EqualsListener implements ActionListener { public void actionPerformed(ActionEvent e) { double answer; // holds the result of the calculation calc.setOp2Value(Double.parseDouble(topText.getText())); if (addS) calc.add(calc.getOp2Value()); else if (subtractS) calc.subtract(calc.getOp2Value()); else if (multiplyS) calc.multiply(calc.getOp2Value()); else if (divideS) calc.divide(calc.getOp2Value()); // update topText topText.setText(String.valueOf(calc.getCurrentValue())); // reset sentinal values decimalS = false; addS = false; subtractS = false; multiplyS = false; divideS = false; for(int i = 0;i<10;i++){ buttons[i].setEnabled(false); } // reset the topNumber topNumber = ""; } } // Event listener for . class DecimalListener implements ActionListener { public void actionPerformed(ActionEvent e) { // don't let the user enter more than one decimal if (!decimalS) { topNumber += "."; topText.setText(topNumber); decimalS = true; } } } // Event listener for C class CancelListener implements ActionListener { public void actionPerformed(ActionEvent e) { // set all operator sentinal values to false addS = false; subtractS = false; multiplyS = false; divideS = false; decimalS = false; // reset number calc.setCurrentValue(0); topNumber = ""; topText.setText("0.0"); } } /* main method to drive the calculator */ public static void main(String[] args) { // Create a new frame JFrame CalcGUI = new CalcGUI(); // set Title to Calculator CalcGUI.setTitle("Calculator"); // set size of Calculator and prevent it from being resized CalcGUI.setSize(400, 250); CalcGUI.setResizable(false);
// Center frame CalcGUI.setLocationRelativeTo(null); // when the window is closed the program ends CalcGUI.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // display the frame CalcGUI.setVisible(true); } }
MemoryCalc code
import java.util.InputMismatchException; import java.util.Scanner;
public class MemoryCalc {
private double currentValue; // the Current value (first operator) private double op2; // the value of the second operator
// Operations functions for +, -, *, / *
public void add(double op2) { currentValue += op2; }
public void subtract(double op2) { currentValue -= op2; }
public void multiply(double op2) { currentValue *= op2; }
public void divide(double op2) { if (op2 == 0) { currentValue = Double.NaN; } else { currentValue /= op2; } }
// Getters and Setters for currentValue and op2 *
public double getCurrentValue() { return currentValue; }
public double getOp2Value() { return op2; }
public void setCurrentValue(double currentValue) { this.currentValue = currentValue; }
public void setOp2Value(double op2) { this.op2 = op2; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
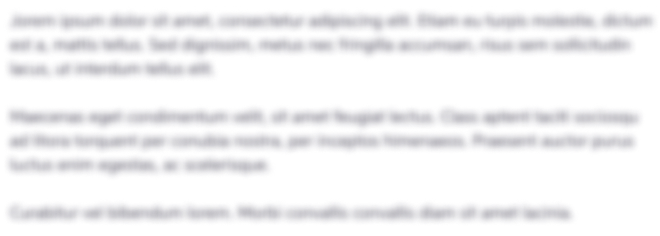
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started