Question
Java GUI for red, green, blue button ... some syntax error I cannot fix. please helps. there are 2 classes thanks. // this program demonstrates
Java GUI for red, green, blue button ... some syntax error I cannot fix. please helps. there are 2 classes thanks.
// this program demonstrates to create the frame and shows the color in circle
// whenever the red or green or blue color button is clicked.
// File: CircleIcon.java
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
// class definition that implements the Icon interface
public class CircleIcon implements Icon{
// declare the private variables
private int size;
private Color color;
// parameterized constructor
public CircleIcon(int aSize, Color color){
// assign the size
size = aSize;
// assign the color
this.color = color;
}
// the setColor() method accepts "color" as an input parameter to set the color for icon
public void setColor(Color color){
// set the icon color
this.color = color;
}
public int getIconWidth(){
// return the size of icon
return size;
}
// this method is implemented from Icon interface to set height for icon
public int getIconHeight(){
// return the size of icon
return size;
}
// this method is implemented from Icon interface to draw and fill the color for circle
public void paintIcon(Component c, Graphics g, int x, int y){
// define "g2" object from Graphics2D class
Graphics2D g2 = (Graphics2D)g;
// ellipse shape to draw the circle image
Ellipse2D.Double planet = new Ellipse2D.Double(x, y, size, size);
// set the color for circle image
g2.setColor(color);
// fill the color for circle image
g2.fill(planet);
}
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// create a class CircleIcon that implements the Icon interface to draw the circle shape image
// create the parameterized constructor to get the size and to draw the circle image
// in the setColor() method // set the color for icon
// in the method getIconWidth() // return the width of the icon.
// in the method getIconHeight() // return the height of the icon.
// in the method paintIcon() // create a graphic object to invoke the methods whenever
// the user wants to draw or color the images
// draw the ecllipse shape for circle, set the colr and fill that color to that circle.
// declare the private variables
// the class ActionMain is used to create the frame and to test the button using the action listeners
// in the main() function // create a frame object for JFrame class.
// Create a button object for JButton class with the button name as "rButton" for red color button
// in the method addActionLister()
// create the "Red" color button using createColorButtonListener() method
// create a button object for JButton class with the button name as "gButton" for green color button.
// in the method addActionLister()
// create the "Green" colr button using createColorButtonListener() method
// Create a button object for JButton class with the button name "bButton" for blue colr button
// in the method addActionLister()
// create the "Blue" color button using createColorButtonListener() method
// invoke the parameterized constructor for CircleIcon() class
// to set the default button color as "Red"
// create the layout and put into the frame using the JFrame object
// create the "red" button and put into the frame using the JFrame object
// create the "green" button and put into the frame using the JFrame object
// create the "blue" button and put into the frame using the JFrame object
// create the label and put into the frame using the JFrame object
// set the default option for close operation in frame using the JFrame object
// set the size of the frame and show it visible using the JFrame object
// in the createZoomButtonListener()
// the action listener is created for button to handle the event such as
// to change the icon colr using the ActionListener interface
// in the method actionPerformed()
// set the icon color and repaint the lable colr
// create objects for icon and label.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
// the classes actionMain is used to create the frame and to test the button using the action listeners
public class ActionMain{
// this main() function is used to create the frame, add the buttons, and draw the circle into
// the frame, whenever the user click the color button icon to display that color into the circle
public static void main(String[] args){
// create objects for icon and label
// create the object for CircleIcon class
private static CircleIcon icon;
// create the object for JLabel class
private static JLabel label;
// create a frame obect for JFrame class
JFrame frameObj = new JFrame();
// declare and intialize the variable
final int FIELD_WIDTH = 20;
// create the button object and set the text as "Red"
JButton rButton = new JButton("Red");
// set colro of the button as red
rButton.addActionListener(createColorButtonListener(Color.RED));
// create the button object and set the text as "Green"
JButton gButton = new JButton("Green");
// set color of the button as green
gButton.addActionListener(createColorButtonListener(Color.GREEN));
// create the button object and set the text as "blue"
JButton bButton = new JButton("Blue");
// set color of the button as green
bButton.addActionListener(createColorButtonListener(Color.BLUE));
// set the default button color as "Red"
icon = new CircleIcon(50, Color.RED);
// create a label object
label = new JLabel(icon);
// set the layout into frame using the frame object
frameObj.setLayout(new FlowLayout());
// add the button into frame using the frame object
frameObj.add(rButton);
// add the button into frame using the frame object
frameObj.add(gButton);
// add the button into frame using the frame object
frameObj.add(bButton);
// add the label into frame using the frame object
frameObj.add(label);
// set the close option into the frame using the frame object
frameObj.setDefaultCloseOperation(JFrameObj.EXIT_ON_CLOSE);
// size the frame using the frame object
frameObj.pack();
// make a frame as visible, initially it is invisible
frameObj.setVisible(true);
}
// this method handles the action event
public static ActionListener createColorButtonListener(final Color theColor){
return new
ActionListener(){
// this method repaint the label color when icon is changed
public void actionPerformed(ActionEvent event){
// set the color for icon using the icon object
icon.setColr(theColor);
// paint the color for label using the label object
label.repaint();
}
};
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
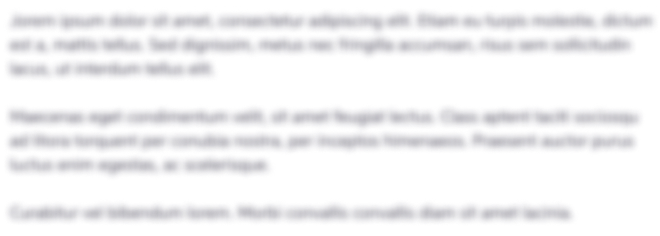
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started