Answered step by step
Verified Expert Solution
Question
1 Approved Answer
java HashMap put method implementation . My code is.. /** * Adds the given key-value pair to the HashMap. * If an entry in the
java HashMap put method implementation. My code is.. /** * Adds the given key-value pair to the HashMap. * If an entry in the HashMap already has this key, replace the entry's * value with the new one passed in. * * In the case of a collision, use linear probing as your resolution * strategy. * * Check to see if the backing array needs to be regrown BEFORE adding. For * example, if my HashMap has a backing array of length 5, and 3 elements in * it, I should regrow at the start of the next add operation (even if it * is a key that is already in the hash map). This means you must account * for the data pending insertion when calculating the load factor. * * When regrowing, increase the length of the backing table by * 2 * old length + 1. Use the resizeBackingTable method. * * Return null if the key was not already in the map. If it was in the map, * return the old value associated with it. * * @param key key to add into the HashMap * @param value value to add into the HashMap * @throws IllegalArgumentException if key or value is null * @return null if the key was not already in the map. If it was in the * map, return the old value associated with it */ @Override public V put(K key, V value) { if (key == null || value == null) { throw new IllegalArgumentException("Key or Value cannot be null"); } if (size + 1 >= (MAX_LOAD_FACTOR * table.length)) { resizeBackingTable(table.length * 2 + 1); } int hash = Math.abs(key.hashCode() % table.length); int curr = 0; int firstDel = table.length; while (true) { if (curr >= table.length) { if (firstDel != table.length) { table[firstDel] = new MapEntry(key, value); return null; } resizeBackingTable(table.length * 2 + 1); curr = 0; } int index = (int) (hash + Math.pow(curr, 2)) % table.length; if (table[index] == null) { table[index] = new MapEntry(key, value); size++; return null; } else if (table[index].isRemoved() && firstDel == table.length) { firstDel = index; } else if (table[index].getKey().equals(key) && table[index].isRemoved()) { table[index] = new MapEntry(key, value); size++; return null; } else if (table[index].getKey().equals(key)) { V retValue = table[index].getValue(); table[index] = new MapEntry(key, value); return retValue; } curr++; } }
and this is result of my test..
Step by Step Solution
There are 3 Steps involved in it
Step: 1
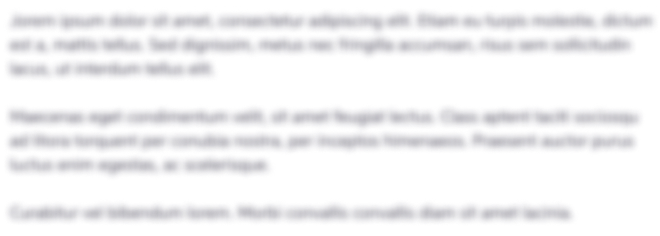
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started