Question
Java help! In this assignment we'll be using object oriented design to tackle the classic 1976 Atari game Breakout, more commonly known today as Brick
Java help!
In this assignment we'll be using object oriented design to tackle the classic 1976 Atari game Breakout, more commonly known today as "Brick Breaker". In Breakout, the player moves a rectangular paddle left and right at the bottom of the screen in order to bounce a ball upward towards a wall of bricks. Bricks have varying strengths, which means that they may require multiple hits from the ball in order to break. The ball will bounce off of the left, top, and right sides of the screen in addition to bouncing off of bricks. The objective of the game is to eliminate all breakable bricks without letting the ball go off the bottom of the screen. If the user does this they have won, if not, they have lost. This game has been re-imagined many times over the years with new features, but the basic mechanics are always the same.
Many games employ a simple object-oriented model of the world to represent the various elements in the game. One key element of this is that the model gets updated incrementally over time by repeated calls to an update or move method. The game GUI will take care of repeatedly calling this method to update the model over and over as time progresses in the game. The tricky part is ensuring that the model maintains accurate information about the state of the "world" at each step.
Essentially, we need to make four new files Ball.java, Brick.java, Paddle.java and Level.java in the same src file which contains BrickBreakerGUI.java, TouchPosition.java and GameState.java. Below is the given starter codes:
BrickBreakerGUI.java
import javafx.animation.AnimationTimer; import javafx.application.Application; import javafx.event.EventHandler; import javafx.scene.input.MouseEvent; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Rectangle; import javafx.scene.text.Font; import javafx.scene.text.Text; import javafx.scene.Cursor; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.control.ButtonType; import javafx.scene.control.ChoiceDialog;
///////////////////////////////////////////////// //DO NOT EDIT THIS FILE. CHANGING THIS CODE //IS NOT REQUIRED BY THE ASSIGNMENT. /////////////////////////////////////////////////
/** * This class provides a GUI interface for the BrickBreaker game. It utilizes * state information provided by a Level object for the underlying game model, * while providing user interactivity through mouse input. * * THIS CLASS IS PROVIDED AND SHOULD NOT BE EDITED. * */ public class BrickBreakerGUI extends Application { /** * The fixed height of the UI screen for the game. */ private static final int GUI_HEIGHT = 440; /** * The fixed width of the UI screen for the game. */ private static final int GUI_WIDTH = 400; /** * The model object used to store all state information about the level * currently being played on this application. */ private Level theLevel; /** * A rectangle that provides the visual component for the Paddle object * inside the Level. */ private Rectangle paddleView; /** * A circle to provide the visual view of the Ball object inside the * Level. */ private Circle ballView; /** * A 2D array of rectangles which is parallel to the Brick objects * stored inside the Level. Used to visually represent the Bricks * on screen. */ private Rectangle[][] bricksView; @Override public void start(Stage theStage) throws Exception { //Ask the user which level they'd like to play ChoiceDialog /** * Skeleton main method as required for launching a JavaFX application. * * @param args Not used. */ public static void main(String[] args) { launch(args); } } TouchPosition.java ///////////////////////////////////////////////// // DO NOT EDIT THIS FILE. CHANGING THIS CODE // IS NOT REQUIRED BY THE ASSIGNMENT. ///////////////////////////////////////////////// /** * This enumerated type represents the possible locations where two objects in the * game are touching one another. * * THIS ENUM DEFINITION IS PROVIDED AND SHOULD NOT BE EDITED. * */ public enum TouchPosition { /** * Used to signal that the Ball has collided with the top of another object. */ TOP, /** * Used to signal that the Ball has collided with the bottom of another object. */ BOTTOM, /** * Used to signal that the Ball has collided with the left side of another object. */ LEFT, /** * Used to signal that the Ball has collided with the right side of another object. */ RIGHT, /** * Used to signal that the Ball has not collided with another object at this time. */ NONE } GameState.java ///////////////////////////////////////////////// // DO NOT EDIT THIS FILE. CHANGING THIS CODE // IS NOT REQUIRED BY THE ASSIGNMENT. ///////////////////////////////////////////////// /** * A simple type to represent the three possible game states in a BrickBreaker * game. * * THIS ENUM DEFINITION IS PROVIDED AND SHOULD NOT BE EDITED. * */ public enum GameState { /** * Represents when the user has lost the game by allow the ball to go off-screen. */ LOST, /** * Represents when the user has one the game by breaking all possible bricks. */ WON, /** * Reprsents when the game is still in progress. */ PLAYING }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
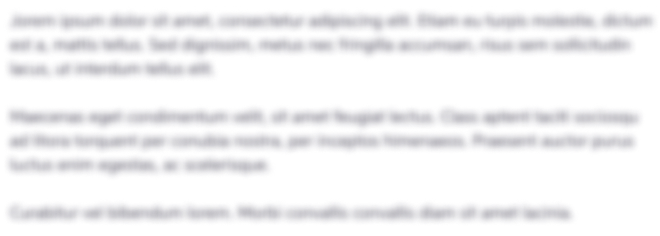
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started