Question
[JAVA Help needed] The given program performs statistical analysis of scores for a class of students by printing student scores and calculate the lowest, highest,
[JAVA Help needed]
The given program performs statistical analysis of scores for a class of students by printing student scores and calculate the lowest, highest, & average score of each quiz for every student. The class may have up to 40 students. There are five quizzes during the term. Each student is identified by a four-digit student ID number.
Task I:
create a separate serialized file using ObjectOutputStream for each student. File should contain student's score and statistics for entire class. Using debug mode (with Debug flag) print the contents of the serialized file.
StudentGrade
Statistics
Student
Write a serialized file for each student.
a) naming convention for each filename.
studentid.ser or studentid.dat
b) method for writing and reading serialized file shld in FileIO or Util.
c) When implementing Serialization - implement Serializable interface in following classes
- StudentGrade
- Student
- Statistics
Designing main()
1. Instantiate StudentGrade.
File has already been read (n records read (upto 40)).
2. Prompt a user - to deliver all grades - (n serialized files are written to disk).
When you serialize - good idea to print before serializing and after serializing - good way to confirm - with DEBUG flag turned on
3. Testing part 1 -
Different # of records in the file.
Boundary condition -
Up to 40 records
41 records - > 40 is ignored.
Header -
1 record
15 records
39 records <-- Boundary condition
40 records <-- Boundary condition
..Should be able to run both.
Task II:
Create and implement an interface to:
Print student statistics.
Print scores for a given student id.
implement the Debug flag globally
Use an abstract class to implement all the methods declared in an interface.
Create and implement an interface to:
Print student statistics.
Create and implement an interface to:
Print student statistics.
Print scores for a given student id.
implement the Debug flag globally
Use an abstract class to implement all the methods declared in an interface.
Print scores for a given student id.
implement the Debug flag globally
Use an abstract class to implement all the methods declared in an interface.
Here are the codes for the program:
Student class : package driverstudentquiz;
public class Student { private int SID; private int scores[] = new int[5]; //get and set functions for scores and ID public int getSID(){ return this.SID; } public void setSID(int id){ this.SID= id; } public int[] getScores(){ return this.scores; } public void setScores(int[] s){ this.scores= s; } public void printData(){ //print all data of a student System.out.println("\t\t\t\t"+ SID +"\t"+ scores[0] +"\t"+ scores[1] +"\t"+ scores[2] + "\t"+ scores[3] +"\t"+ scores[4] +" "); } }
Statistics class : package driverstudentquiz;
public class Statistics { private int[] lowscores= new int[5]; private int[] highscores= new int[5]; private float[] avgscores= new float[5]; void findlow(Student[] a, int c){ // finds low score. int i=0; for(i=0;i<5; i++){ int[] temp= a[0].getScores(); int min = temp[i]; for(int j=0; j< c; j++){ int[] s= a[j].getScores(); if(min > s[i]){ min = s[i]; } } lowscores[i]= min; } } void findhigh(Student[] a, int c){ // find high score int i=0; for(i=0;i<5; i++){ int[] temp= a[0].getScores(); int max = temp[i]; for(int j=0; j< c; j++){ int[] s= a[j].getScores(); if(max < s[i]){ max = s[i]; } } highscores[i]= max; } } void findavg(Student[] a, int c){ // find average score. int i=0; for(i=0;i<5; i++){ int sum =0; for(int j=0; j int[] s= a[j].getScores(); sum= sum + s[i]; } avgscores[i]= sum/c; } } public void printStatistics(Student[] a, int c){ // print statistics for all students and all quizes findlow(a,c); findhigh(a,c); findavg(a,c); System.out.println("Lowest score :\t\t"+ lowscores[0] +"\t"+ lowscores[1] +"\t"+ lowscores[2] +"\t"+ lowscores[3] +"\t"+ lowscores[4] ); System.out.println("High score :\t\t"+ highscores[0] +"\t"+ highscores[1] +"\t"+ highscores[2] +"\t"+ highscores[3] +"\t"+ highscores[4] ); System.out.println("Average score :\t\t"+ avgscores[0] +"\t"+ avgscores[1] +"\t"+ avgscores[2] +"\t"+ avgscores[3] +"\t"+ avgscores[4] ); } }
Util class : package driverstudentquiz; import java.io.*; import java.util.StringTokenizer;
public class Util { static int studentCount; static Student [] readFile(String filename, Student [] stu) { //Reads the file and builds student array. int i=0;
try { FileReader file = new FileReader(filename); //Open the file using FileReader Object. BufferedReader buff = new BufferedReader(file); boolean eof = false; while (!eof) { String line = buff.readLine(); //In a loop read a line using readLine method. if (line == null) eof = true; else{ // System.out.println(line); stu[i]= new Student(); StringTokenizer st = new StringTokenizer(line); //Tokenize each line using StringTokenizer Object while(st.hasMoreTokens()){ stu[i].setSID(Integer.parseInt(st.nextToken())); //Value is then saved in the right property of Student Object. int[] arr= new int[5]; for(int j=0;j<5;j++){ arr[j]= Integer.parseInt(st.nextToken()); //Each token is converted from String to Integer using parseInt method } stu[i].setScores(arr); //Value is then saved in the right property of Student Object. } } i++; } buff.close(); } catch (IOException e) { System.out.println("Error -- " + e.toString()); } studentCount = i-1; return stu; } }
Driver class : package driverstudentquiz;
public class DriverStudentQuiz {
public static void main(String[] args) { Student arrStudent[] = new Student[40]; int studentCount=0; arrStudent = Util.readFile("Data.txt", arrStudent); studentCount= Util.studentCount; // find number of lines from file. which will show number of students in array. Statistics s = new Statistics(); for(int i = 0; i arrStudent[i].printData(); } s.printStatistics(arrStudent,studentCount); // print statistics of students } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
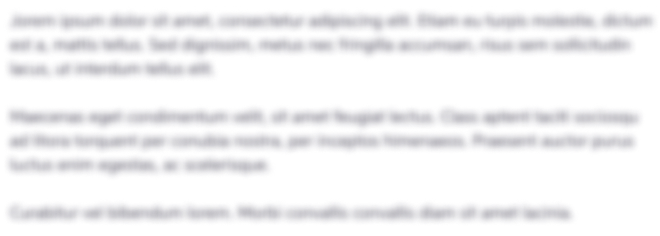
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started