Question
[Java Help] Write a class that represents a credit card. Call this class CreditCard . The class will have the following fields and methods: A
[Java Help] Write a class that represents a credit card. Call this class CreditCard. The class will have the following fields and methods:
A constructor which takes a String to set the card number. Ignore dashes, spaces, and any other non-digit characters.
A public method called toString that returns the credit card number.
Add the following data and operations to CreditCard:
- A public method called getIssuer that returns the String name of the company who issued the credit card. Popular issuers are as follows:
Issuer | Identifier | Card Number Length |
---|---|---|
Diner's Club/Carte Blanche | 300xxx-305xxx, 36xxxx, 38xxxx | 14 |
American Express | 34xxxx, 37xxxx | 15 |
VISA | 4xxxxx | 13, 16 |
MasterCard | 51xxxx-55xxxx | 16 |
Discover | 6011xx | 16 |
- Modify toString to include the issuer's name.
Add the following data and operations to CreditCard:
- A public method called isValid which returns a boolean value representing the validity of the card number.
The algorithm can be described as follows: "For a card with an even number of digits, double every odd-numbered digit and subtract 9 if the product is greater than 9. Add up all the even digits as well as the doubled-odd digits, and the result must be a multiple of 10 or it's not a valid card. If the card has an odd number of digits, perform the same addition doubling the even numbered digits instead."
For example, "[Let's] apply the Luhn check to 4408 0012 3456 7890. (4*2)+4+(0*2)+8+(0*2)+0+(1*2)+2+(3*2)+4+(5*2-9)+6+(7*2-9)+8+(9*2-9)+0 = 8+4+0+8+0+0+2+2+6+4+1+6+5+8+9+0 = 63, which is not a multiple of 10. Therefore we conclude that the number 4408 0012 3456 7890 is an invalid credit card number."
-
Input: sol.java:
378282246310005 371449635398431 378734493671000 5610591081018250 30569309025904 38520000023237 6011111111111117 6011000990139424 3530111333300000 3566002020360505 5555555555554444 5105105105105100 4111111111111111 4012888888881881 4222222222222 76009244561 5019717010103742 6331101999990016 1 338282246310005 374449635398431 378534493671000 5610691081018250 30569709025904 38520080023237 6011111911111117 6011003900139424 3530111331300000 3566002020160505 1555555555554444 5205105105105100 4131111111111111 4014888868881881 4222522222222 76009644571 5019717010103742 6331101899890016 Required Output: 378282246310005 was issued by American Express and is valid. 371449635398431 was issued by American Express and is valid. 378734493671000 was issued by American Express and is valid. 5610591081018250 was issued by Unknown and is valid. 30569309025904 was issued by Diner's Club and is valid. 38520000023237 was issued by Diner's Club and is valid. 6011111111111117 was issued by Discover and is valid. 6011000990139424 was issued by Discover and is valid. 3530111333300000 was issued by Unknown and is valid. 3566002020360505 was issued by Unknown and is valid. 5555555555554444 was issued by MasterCard and is valid. 5105105105105100 was issued by MasterCard and is valid. 4111111111111111 was issued by VISA and is valid. 4012888888881881 was issued by VISA and is valid. 4222222222222 was issued by VISA and is valid. 76009244561 was issued by Unknown and is not valid. 5019717010103742 was issued by Unknown and is valid. 6331101999990016 was issued by Unknown and is valid. 1 was issued by Unknown and is not valid. 338282246310005 was issued by Unknown and is not valid. 374449635398431 was issued by American Express and is not valid. 378534493671000 was issued by American Express and is not valid. 5610691081018250 was issued by Unknown and is not valid. 30569709025904 was issued by Diner's Club and is not valid. 38520080023237 was issued by Diner's Club and is not valid. 6011111911111117 was issued by Discover and is not valid. 6011003900139424 was issued by Discover and is not valid. 3530111331300000 was issued by Unknown and is not valid. 3566002020160505 was issued by Unknown and is not valid. 1555555555554444 was issued by Unknown and is not valid. 5205105105105100 was issued by MasterCard and is not valid. 4131111111111111 was issued by VISA and is not valid. 4014888868881881 was issued by VISA and is not valid. 4222522222222 was issued by VISA and is not valid. 76009644571 was issued by Unknown and is not valid. 5019717010103742 was issued by Unknown and is valid. 6331101899890016 was issued by Unknown and is not valid.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
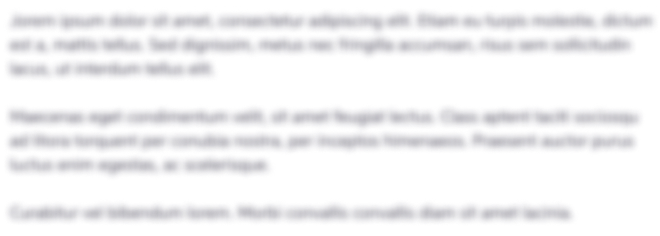
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started