Question
***Java*** Here is my code, but my tax is not calculating properly. This runs properly but the tax is not correct. It is supposed to
***Java***
Here is my code, but my tax is not calculating properly. This runs properly but the tax is not correct. It is supposed to be 7% or .07. I can get the amount to be correct if I assign the variable to the mcTax of .93, but I believe there is a better way to code this. I just want the tax (7%) to be figured on the SubTotal and then for the tax to be added to the Subtotal to reach the Total. There should be a better way to code this in Java. Thank you!
package receipt;
import java.awt.event.KeyEvent;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class Parent_Inheritence {
public JFrame frame;
//Data types
public double Haircut;
public double Trim;
public double Beardtrim;
public double Single;
public double Highlights;
public double Retouch;
public double Scalp;
public double Protein;
public double pHaircut = 20.00;
public double pTrim = 15.00;
public double pBeardtrim = 8.00;
public double pSingle = 50.00;
public double pHighlights = 65.00;
public double pRetouch = 40.00;
public double pScalp = 15.00;
public double pProtein = 20.00;
public double servicescost1;
public double servicescost2;
public double servicescost3;
public double servicescost;
public double TaxCost;
public double GetAmount() {
servicescost1 = Haircut + Trim + Beardtrim;
servicescost2 = Single + Highlights + Retouch;
servicescost3 = Scalp + Protein;
servicescost = servicescost1 + servicescost2 + servicescost3;
return(servicescost);
}
public void iExitSystem() {
frame = new JFrame();
if (JOptionPane.showConfirmDialog(frame, "Confirm if you want to exit", "Receipt Services",
JOptionPane.YES_NO_OPTION)==JOptionPane.YES_NO_OPTION);
System.exit(0);
}
//calculate tax
public double mcTax = .07;
public Double cFindTax(double cAmount) {
double FindTax = cAmount - (cAmount * mcTax);
return(FindTax);
}
//calculate tax
public double GetTax() {
TaxCost = cFindTax(servicescost);
return (TaxCost);
}
//numbers only
public void NumberOnly(java.awt.event.KeyEvent evt) {
char iNumber = evt.getKeyChar();
if(!(Character.isDigit(iNumber)) || (iNumber == KeyEvent.VK_BACK_SPACE) || (iNumber == KeyEvent.VK_DELETE)) {
evt.consume();
}
}
}
package receipt;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.LineBorder;
import java.awt.Color;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.SwingConstants;
import java.awt.Component;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.GridLayout;
import javax.swing.JTextField;
import java.awt.Dimension;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
public class receipt_services {
private JFrame frmHeathersHaircareReceipt;
private JTextField textField_haircut;
private JTextField textField_trim;
private JTextField textField_beardtrim;
private JTextField textField_single;
private JTextField textField_highlights;
private JTextField textField_retouch;
private JTextField textField_scalp;
private JTextField textField_protein;
private JTextField textField_cost_cuts;
private JTextField textField_cost_color;
private JTextField textField_cost_condition;
private JTextField textField_Tax;
private JTextField textField_Subtotal;
private JTextField textField_Total;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
receipt_services window = new receipt_services();
window.frmHeathersHaircareReceipt.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public receipt_services() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frmHeathersHaircareReceipt = new JFrame();
frmHeathersHaircareReceipt.setTitle("Heather's Haircare Receipt");
frmHeathersHaircareReceipt.setBounds(0, 0, 900, 600);
frmHeathersHaircareReceipt.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frmHeathersHaircareReceipt.getContentPane().setLayout(null);
JPanel panel = new JPanel();
panel.setMaximumSize(new Dimension(32500, 32767));
panel.setBorder(new LineBorder(new Color(0, 0, 0), 4));
panel.setBounds(42, 11, 800, 77);
frmHeathersHaircareReceipt.getContentPane().add(panel);
JLabel lblHeathersHairCare = new JLabel("Thank you for choosing Heather's Hair Care!");
lblHeathersHairCare.setHorizontalAlignment(SwingConstants.CENTER);
lblHeathersHairCare.setFont(new Font("Tahoma", Font.BOLD, 32));
panel.add(lblHeathersHairCare);
JPanel panel_1 = new JPanel();
panel_1.setBorder(new LineBorder(new Color(0, 0, 0), 4));
panel_1.setBounds(10, 99, 625, 343);
frmHeathersHaircareReceipt.getContentPane().add(panel_1);
panel_1.setLayout(null);
JLabel lblHairCut = new JLabel("Hair Cut");
lblHairCut.setFont(new Font("Tahoma", Font.BOLD, 18));
lblHairCut.setBounds(39, 70, 87, 22);
panel_1.add(lblHairCut);
JLabel lblTrim = new JLabel("Trim");
lblTrim.setFont(new Font("Tahoma", Font.BOLD, 18));
lblTrim.setBounds(39, 103, 87, 22);
panel_1.add(lblTrim);
JLabel lblBeardTrim = new JLabel("Beard Trim");
lblBeardTrim.setFont(new Font("Tahoma", Font.BOLD, 18));
lblBeardTrim.setBounds(39, 136, 107, 22);
panel_1.add(lblBeardTrim);
textField_haircut = new JTextField();
//number only
textField_haircut.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_haircut.setHorizontalAlignment(SwingConstants.RIGHT);
textField_haircut.setText("0");
textField_haircut.setBorder(new LineBorder(Color.BLACK, 2));
textField_haircut.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_haircut.setBounds(172, 72, 86, 20);
panel_1.add(textField_haircut);
textField_haircut.setColumns(10);
textField_trim = new JTextField();
//number only
textField_trim.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_trim.setHorizontalAlignment(SwingConstants.RIGHT);
textField_trim.setText("0");
textField_trim.setBorder(new LineBorder(Color.BLACK, 2));
textField_trim.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_trim.setBounds(172, 103, 86, 20);
panel_1.add(textField_trim);
textField_trim.setColumns(10);
textField_beardtrim = new JTextField();
//number only
textField_beardtrim.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_beardtrim.setHorizontalAlignment(SwingConstants.RIGHT);
textField_beardtrim.setText("0");
textField_beardtrim.setBorder(new LineBorder(Color.BLACK, 2));
textField_beardtrim.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_beardtrim.setColumns(10);
textField_beardtrim.setBounds(172, 136, 86, 20);
panel_1.add(textField_beardtrim);
JLabel lblSingle = new JLabel("Single Process");
lblSingle.setFont(new Font("Tahoma", Font.BOLD, 18));
lblSingle.setBounds(301, 70, 130, 22);
panel_1.add(lblSingle);
JLabel lblHighlightslowlights = new JLabel("Highlights/Lowlights");
lblHighlightslowlights.setFont(new Font("Tahoma", Font.BOLD, 18));
lblHighlightslowlights.setBounds(301, 103, 187, 22);
panel_1.add(lblHighlightslowlights);
JLabel lblRetouch = new JLabel("Retouch");
lblRetouch.setFont(new Font("Tahoma", Font.BOLD, 18));
lblRetouch.setBounds(301, 136, 87, 22);
panel_1.add(lblRetouch);
JLabel lblCutsTrim = new JLabel("CUTS & TRIM");
lblCutsTrim.setFont(new Font("Tahoma", Font.BOLD, 22));
lblCutsTrim.setBounds(76, 30, 173, 22);
panel_1.add(lblCutsTrim);
JLabel lblColor = new JLabel("COLOR");
lblColor.setFont(new Font("Tahoma", Font.BOLD, 22));
lblColor.setBounds(357, 30, 94, 22);
panel_1.add(lblColor);
textField_single = new JTextField();
//number only
textField_single.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_single.setHorizontalAlignment(SwingConstants.RIGHT);
textField_single.setText("0");
textField_single.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_single.setColumns(10);
textField_single.setBorder(new LineBorder(Color.BLACK, 2));
textField_single.setBounds(498, 72, 86, 20);
panel_1.add(textField_single);
textField_highlights = new JTextField();
//number only
textField_highlights.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_highlights.setHorizontalAlignment(SwingConstants.RIGHT);
textField_highlights.setText("0");
textField_highlights.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_highlights.setColumns(10);
textField_highlights.setBorder(new LineBorder(Color.BLACK, 2));
textField_highlights.setBounds(498, 105, 86, 20);
panel_1.add(textField_highlights);
textField_retouch = new JTextField();
//number only
textField_retouch.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_retouch.setHorizontalAlignment(SwingConstants.RIGHT);
textField_retouch.setText("0");
textField_retouch.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_retouch.setColumns(10);
textField_retouch.setBorder(new LineBorder(Color.BLACK, 2));
textField_retouch.setBounds(498, 138, 86, 20);
panel_1.add(textField_retouch);
JLabel lblCondition = new JLabel("DEEP CONDITIONER");
lblCondition.setFont(new Font("Tahoma", Font.BOLD, 22));
lblCondition.setBounds(192, 209, 238, 22);
panel_1.add(lblCondition);
JLabel lblScalpTreatment = new JLabel("Scalp Treatment");
lblScalpTreatment.setFont(new Font("Tahoma", Font.BOLD, 18));
lblScalpTreatment.setBounds(171, 251, 167, 22);
panel_1.add(lblScalpTreatment);
JLabel lblProteinTreatment = new JLabel("Protein Treatment");
lblProteinTreatment.setFont(new Font("Tahoma", Font.BOLD, 18));
lblProteinTreatment.setBounds(171, 284, 167, 22);
panel_1.add(lblProteinTreatment);
textField_scalp = new JTextField();
//number only
textField_scalp.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_scalp.setHorizontalAlignment(SwingConstants.RIGHT);
textField_scalp.setText("0");
textField_scalp.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_scalp.setColumns(10);
textField_scalp.setBorder(new LineBorder(Color.BLACK, 2));
textField_scalp.setBounds(374, 251, 86, 20);
panel_1.add(textField_scalp);
textField_protein = new JTextField();
//number only
textField_protein.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
Child_Inheritence JustNumbers = new Child_Inheritence();
JustNumbers.NumberOnly(e);
}
});
textField_protein.setHorizontalAlignment(SwingConstants.RIGHT);
textField_protein.setText("0");
textField_protein.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField_protein.setColumns(10);
textField_protein.setBorder(new LineBorder(Color.BLACK, 2));
textField_protein.setBounds(374, 282, 86, 20);
panel_1.add(textField_protein);
JPanel panel_2 = new JPanel();
panel_2.setBorder(new LineBorder(new Color(0, 0, 0), 4));
panel_2.setBounds(645, 99, 226, 111);
frmHeathersHaircareReceipt.getContentPane().add(panel_2);
panel_2.setLayout(null);
JLabel lblCostOfCuts = new JLabel("Cost of Cuts");
lblCostOfCuts.setFont(new Font("Tahoma", Font.BOLD, 14));
lblCostOfCuts.setBounds(10, 11, 107, 20);
panel_2.add(lblCostOfCuts);
JLabel lblCostOfColor = new JLabel("Cost of Color");
lblCostOfColor.setFont(new Font("Tahoma", Font.BOLD, 14));
lblCostOfColor.setBounds(10, 42, 107, 20);
panel_2.add(lblCostOfColor);
JLabel lblCostOfCondition = new JLabel("Cost of Condition");
lblCostOfCondition.setFont(new Font("Tahoma", Font.BOLD, 14));
lblCostOfCondition.setBounds(10, 73, 126, 20);
panel_2.add(lblCostOfCondition);
textField_cost_cuts = new JTextField();
textField_cost_cuts.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_cost_cuts.setColumns(10);
textField_cost_cuts.setBorder(new LineBorder(Color.BLACK, 2));
textField_cost_cuts.setBounds(137, 13, 76, 20);
panel_2.add(textField_cost_cuts);
textField_cost_color = new JTextField();
textField_cost_color.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_cost_color.setColumns(10);
textField_cost_color.setBorder(new LineBorder(Color.BLACK, 2));
textField_cost_color.setBounds(137, 42, 76, 20);
panel_2.add(textField_cost_color);
textField_cost_condition = new JTextField();
textField_cost_condition.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_cost_condition.setColumns(10);
textField_cost_condition.setBorder(new LineBorder(Color.BLACK, 2));
textField_cost_condition.setBounds(137, 75, 76, 20);
panel_2.add(textField_cost_condition);
JPanel panel_3 = new JPanel();
panel_3.setBorder(new LineBorder(new Color(0, 0, 0), 4));
panel_3.setBounds(645, 213, 226, 111);
frmHeathersHaircareReceipt.getContentPane().add(panel_3);
panel_3.setLayout(null);
JLabel labelTax = new JLabel("Tax");
labelTax.setFont(new Font("Tahoma", Font.BOLD, 14));
labelTax.setBounds(10, 11, 107, 20);
panel_3.add(labelTax);
textField_Tax = new JTextField();
textField_Tax.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_Tax.setColumns(10);
textField_Tax.setBorder(new LineBorder(Color.BLACK, 2));
textField_Tax.setBounds(137, 13, 76, 20);
panel_3.add(textField_Tax);
JLabel lblSubtotal = new JLabel("Subtotal");
lblSubtotal.setFont(new Font("Tahoma", Font.BOLD, 14));
lblSubtotal.setBounds(10, 42, 107, 20);
panel_3.add(lblSubtotal);
textField_Subtotal = new JTextField();
textField_Subtotal.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_Subtotal.setColumns(10);
textField_Subtotal.setBorder(new LineBorder(Color.BLACK, 2));
textField_Subtotal.setBounds(137, 44, 76, 20);
panel_3.add(textField_Subtotal);
JLabel lblTotal = new JLabel("Total");
lblTotal.setFont(new Font("Tahoma", Font.BOLD, 14));
lblTotal.setBounds(10, 73, 107, 20);
panel_3.add(lblTotal);
textField_Total = new JTextField();
textField_Total.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField_Total.setColumns(10);
textField_Total.setBorder(new LineBorder(Color.BLACK, 2));
textField_Total.setBounds(137, 75, 76, 20);
panel_3.add(textField_Total);
JPanel panel_4 = new JPanel();
panel_4.setBorder(new LineBorder(new Color(0, 0, 0), 4));
panel_4.setBounds(645, 331, 226, 111);
frmHeathersHaircareReceipt.getContentPane().add(panel_4);
panel_4.setLayout(null);
JButton btnTotal = new JButton("Total");
btnTotal.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
Child_Inheritence cost_of_services = new Child_Inheritence();
double iTax, iSubtotal, iTotal;
cost_of_services.Haircut = cost_of_services.pHaircut * Double.parseDouble(textField_haircut.getText());
cost_of_services.Trim = cost_of_services.pTrim * Double.parseDouble(textField_trim.getText());
cost_of_services.Beardtrim = cost_of_services.pBeardtrim * Double.parseDouble(textField_beardtrim.getText());
cost_of_services.Single = cost_of_services.pSingle * Double.parseDouble(textField_single.getText());
cost_of_services.Highlights = cost_of_services.pHighlights * Double.parseDouble(textField_highlights.getText());
cost_of_services.Retouch = cost_of_services.pRetouch * Double.parseDouble(textField_retouch.getText());
cost_of_services.Scalp = cost_of_services.pScalp * Double.parseDouble(textField_scalp.getText());
cost_of_services.Protein = cost_of_services.pProtein * Double.parseDouble(textField_protein.getText());
iSubtotal = cost_of_services.GetAmount();
iTax = cost_of_services.GetTax();
iTotal = iSubtotal + iTax;
String Subtotal = String.format("$%.2f", iSubtotal);
textField_Subtotal.setText(Subtotal);
String Tax = String.format("$%.2f", iTax);
textField_Tax.setText(Tax);
String Total = String.format("$%.2f", iTotal);
textField_Total.setText(Total);
//Set Text
String iCuts = String.format("$%.2f", cost_of_services.servicescost1);
textField_cost_cuts.setText(iCuts);
String iColor = String.format("$%.2f", cost_of_services.servicescost2);
textField_cost_color.setText(iColor);
String iCondition = String.format("$%.2f", cost_of_services.servicescost3);
textField_cost_condition.setText(iCondition);
}
});
btnTotal.setFont(new Font("Tahoma", Font.BOLD, 18));
btnTotal.setBounds(27, 9, 174, 23);
panel_4.add(btnTotal);
JButton btnReset = new JButton("Reset");
btnReset.addActionListener(new ActionListener() {
//reset button event handler
public void actionPerformed(ActionEvent arg0) {
textField_haircut.setText("0");
textField_trim.setText("0");
textField_beardtrim.setText("0");
textField_single.setText("0");
textField_highlights.setText("0");
textField_retouch.setText("0");
textField_scalp.setText("0");
textField_protein.setText("0");
textField_cost_cuts.setText(null);
textField_cost_color.setText(null);
textField_cost_condition.setText(null);
textField_Tax.setText(null);
textField_Subtotal.setText(null);
textField_Total.setText(null);
}
});
btnReset.setFont(new Font("Tahoma", Font.BOLD, 18));
btnReset.setBounds(27, 43, 174, 23);
panel_4.add(btnReset);
JButton btnExit = new JButton("Exit");
btnExit.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
Child_Inheritence qExit = new Child_Inheritence();
qExit.iExitSystem();
}
});
btnExit.setFont(new Font("Tahoma", Font.BOLD, 18));
btnExit.setBounds(27, 77, 174, 23);
panel_4.add(btnExit);
}
}
package receipt;
public class Child_Inheritence extends Parent_Inheritence{
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
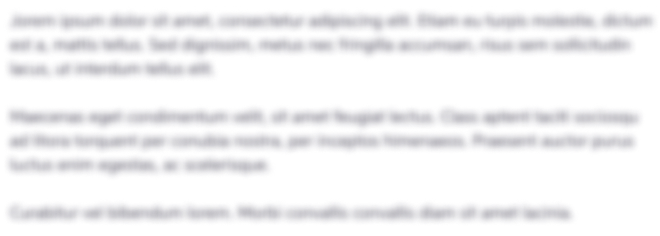
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started