Question
JAVA- How do I update this code to add the new guidelines? Add the following modifications to these two Java Files: Now we are going
JAVA- How do I update this code to add the new guidelines?
Add the following modifications to these two Java Files:
Now we are going to add to our Account Business Class. We need to add 2 methods:
- deposit(amt); // adds the amt to the balance in the object and
// also updates the balance in the DB
- withdraw(amt); //same as deposit above, except subtract
Account a1 = new Account();
//so to find an account in the database, call selectDB().
a1.selectDB(3001);
// so now to make a deposit we simply
a1.deposit(300.00);
// this method will display the new account balance
a1.display();
Part II Now build an AccountList Business Class. This class will have a list of Account Objects. You can use an Array or an ArrayList to store this list. I would also have a count property to keep track of how many Accounts are on the list. Methods should include a display() method, and an add (Account a1) method.
Part III Now add a new Property to the Customer class.
AccountList aList;
Make it so that when an User calls the selectDB(3001) method(and passes a custid), the Customer class will go look in the database for all accounts that belong to this customer and put them in the use the aList object. So the Customer class will contain a list of Accounts that the customer owns.
HINT: You do not need buttons for all the numbers 1,2,3,4,5,6,7,8,9,0. If you make your calculator like this, it becomes much more complex. Keep your calculator simple by following the instructions above.
*************************
CustomerBusiness.java
package com.bank;
import java.util.List;
public class CustomerBusiness {
private int CustId;
private String CustPassword;
private String CustFirstName;
private String CustLastName;
private String CustAddress;
private String CustEmail;
public int getCustId() {
return CustId;
}
public void setCustId(int custId) {
CustId = custId;
}
public String getCustPassword() {
return CustPassword;
}
public void setCustPassword(String custPassword) {
CustPassword = custPassword;
}
public String getCustFirstName() {
return CustFirstName;
}
public void setCustFirstName(String custFirstName) {
CustFirstName = custFirstName;
}
public String getCustLastName() {
return CustLastName;
}
public void setCustLastName(String custLastName) {
CustLastName = custLastName;
}
public String getCustAddress() {
return CustAddress;
}
public void setCustAddress(String custAddress) {
CustAddress = custAddress;
}
public String getCustEmail() {
return CustEmail;
}
public void setCustEmail(String custEmail) {
CustEmail = custEmail;
}
public CustomerBusiness(int custId, String custPassword, String custFirstName, String custLastName,
String custAddress, String custEmail) {
super();
CustId = custId;
CustPassword = custPassword;
CustFirstName = custFirstName;
CustLastName = custLastName;
CustAddress = custAddress;
CustEmail = custEmail;
}
public CustomerBusiness(){
super();
CustId = 0;
CustPassword = "";
CustFirstName = "";
CustLastName = "";
CustAddress = "";
CustEmail = "";
}
public void insertDb(int custId, String custPassword, String custFirstName, String custLastName,
String custAddress, String custEmail){
}
public String deleteDb(int custId){
return null;
}
public List
return null;
}
}
***********************
AccountBusiness.java
package com.bank;
public class AccountBusiness {
public String deposit(double amt){
return null;
}
public String withdraw(double amt){
return null;
}
public double getBalance(int acctid){
return 0;
}
}
Customer.java
package com.bank;
import java.util.List;
public class Customer {
CustomerBusiness c1 = new CustomerBusiness();
AccountList alist;
public boolean validatePassword(int custid,String passwordUser){
List
if(!mylist.isEmpty()){
String password = mylist.get(0).getCustPassword();
if(password.equals(passwordUser)){
//valid log in
return true;
}else{
return false;
//invalid login
}
}
return false;
}
}
Account.java
package com.bank;
import java.util.List;
public class Account {
private int acctId;
private double Balance;
private int custId;
public int getAcctId() {
return acctId;
}
public void setAcctId(int acctId) {
this.acctId = acctId;
}
public double getBalance() {
return Balance;
}
public void setBalance(double balance) {
Balance = balance;
}
public int getCustId() {
return custId;
}
public void setCustId(int custId) {
this.custId = custId;
}
AccountBusiness ab = new AccountBusiness();
public List
ab.deposit(100.00);
return null;
}
public void Display(){
System.out.println("display all balances");
}
}
AccountList.java
package com.bank;
public class AccountList {
public void addAcount(Account a1){
}
public void display(int custid){
System.out.println("arraylist to display all");
}
}
Loginpage.jsp
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
LoginServlet.java
package com.bank;
import java.io.IOException;
import javax.servlet.Servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServlet;
public class LoginServlet implements Servlet{
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public ServletConfig getServletConfig() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getServletInfo() {
// TODO Auto-generated method stub
return null;
}
@Override
public void init(ServletConfig arg0) throws ServletException {
// TODO Auto-generated method stub
}
@Override
public void service(ServletRequest request, ServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
int custid = Integer.parseInt(request.getParameter("custid"));
String password = request.getParameter("password");
Customer c1=new Customer();
if(c1.validatePassword(custid,password)){
}else{
}
}
}
web.xml
Now we are going to add to our Account Business Class. We need to add 2 methods:
deposit(amt); // adds the amt to the balance in the object and
// also updates the balance in the DB
withdraw(amt); //same as deposit above, except subtract
Account a1 = new Account();
//so to find an account in the database, call selectDB().
a1.selectDB(3001);
// so now to make a deposit we simply
a1.deposit(300.00);
// this method will display the new account balance
a1.display();
Part II Now build an AccountList Business Class. This class will have a list of Account Objects. You can use an Array or an ArrayList to store this list. I would also have a count property to keep track of how many Accounts are in the list. Methods should include a display() method, and an add(Account a1) method.
Part III Now add a new Property to the Customer class.
AccountList aList;
Make it so that when an User calls the selectDB(3001) method(and passes a custid), the Customer class will go look in the database for all accounts that belong to this customer and put them in the use the aList object. So the Customer class will contain a list of Accounts that the customer owns.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
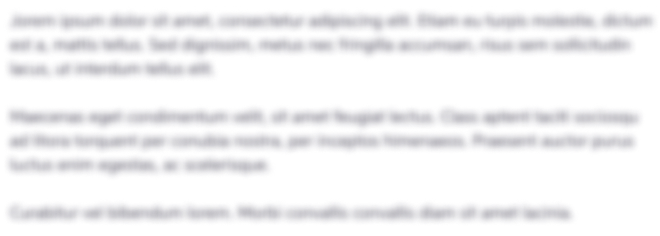
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started