Question
JAVA - how to answer part b using code below from part a b: Write another Java application with the same functionality as outlined above,
JAVA - how to answer part b using code below from part a
b: Write another Java application with the same functionality as outlined above, in part a of this question, but this time using Datagram type sockets. Hint: you can use ByteArrayOutputStream and ByteArrayInputStream to populate and read the array associated with the DatagramPacket object. This application does not need to implement a reliable data transfer protocol. The answer only needs to include source code for the server side application. 13 MARKS
Java code for server side has two classes
1.serverside.java
2.ServerThread.java
For proper understanding I have written client side code in Client.java
If client sends input as string"Hi, how r u" server is recieving it and acknowleding it.
Following is the output and code for same.
OUTPUT:
Server starting...
Accepting Connection...
Server recieved this text:hi, how r u
CODE:
serverside.java
import java.io.*;
import java.net.*;
public class serverside {
public static void main (String [] args) throws IOException
{
System.out.println ("Server starting... ");
// Create a server socket that listens for incoming connection
// requests on port 10000.
ServerSocket server = new ServerSocket (10000);
while (true)
{
// Listen for incoming connection requests from client
// programs, establish a connection, and return a Socket
// object that represents this connection.
Socket s = server.accept ();
System.out.println ("Accepting Connection... ");
// Start a thread to handle the connection.
new ServerThread (s).start ();
}
}
}
ServerThread.java
import java.io.*;
import java.net.*;
public class ServerThread extends Thread{
private Socket s;
ServerThread (Socket s)
{
this.s = s;
}
public void run ()
{
BufferedReader br = null;
PrintWriter pw = null;
try
{
// Create an input stream reader that chains to the socket's
// byte-oriented input stream. The input stream reader
// converts bytes read from the socket to characters. The
// conversion is based on the platform's default character
// set.
InputStreamReader isr;
isr = new InputStreamReader (s.getInputStream ());
// Create a buffered reader that chains to the input stream
// reader. The buffered reader supplies a convenient method
// for reading entire lines of text.
br = new BufferedReader (isr);
// Create a print writer that chains to the socket's byte-
// oriented output stream. The print writer creates an
// intermediate output stream writer that converts
// characters sent to the socket to bytes. The conversion
// is based on the platform's default character set.
pw = new PrintWriter (s.getOutputStream (), true);
// Because the client program may send multiple commands, a
// loop is required. Keep looping until the client either
// explicitly requests termination by sending a command
// beginning with letters BYE or implicitly requests
// termination by closing its output stream.
do
{
// Obtain the client program's next command.
String cmd = br.readLine ();
// Exit if client program has closed its output stream.
if (cmd == null)
break;
// Convert command to uppercase, for ease of comparison.
System.out.println ("Server recieved this text:"+cmd);
cmd = cmd.toUpperCase ();
// If client program sends BYE command, terminate.
if (cmd.startsWith ("BYE"))
break;
}
while (true);
}
catch (IOException e)
{
System.out.println (e.toString ());
}
finally
{
System.out.println ("Closing Connection... ");
try
{
if (br != null)
br.close ();
if (pw != null)
pw.close ();
if (s != null)
s.close ();
}
catch (IOException e)
{
}
}
}
}
Client.java
import java.io.*;
import java.net.*;
public class Client {
public static void main (String [] args)
{
String host = "localhost";
// If user specifies a command-line argument, that argument
// represents the host name.
if (args.length == 1)
host = args [0];
BufferedReader br = null;
PrintWriter pw = null;
Socket s = null;
try
{
// Create a socket that attempts to connect to the server
// program on the host at port 10000.
s = new Socket (host, 10000);
// Create an input stream reader that chains to the socket's
// byte-oriented input stream. The input stream reader
// converts bytes read from the socket to characters. The
// conversion is based on the platform's default character
// set.
InputStreamReader isr;
isr = new InputStreamReader (s.getInputStream ());
// Create a buffered reader that chains to the input stream
// reader. The buffered reader supplies a convenient method
// for reading entire lines of text.
br = new BufferedReader (isr);
// Create a print writer that chains to the socket's byte-
// oriented output stream. The print writer creates an
// intermediate output stream writer that converts
// characters sent to the socket to bytes. The conversion
// is based on the platform's default character set.
pw = new PrintWriter (s.getOutputStream (), true);
// Send the command to the server.
pw.println ("hi, how r u");
// Obtain and print the current date/time.
System.out.println (br.readLine ());
}
catch (IOException e)
{
System.out.println (e.toString ());
}
finally
{
try
{
if (br != null)
br.close ();
if (pw != null)
pw.close ();
if (s != null)
s.close ();
}
catch (IOException e)
{
}
}
}
}
3a: Write a network server program in Java where the server waits for incoming client connections using stream type sockets. Once a client connects it sends a String object to the server with a simple query - the server then responds with a text based response. The connection is then terminated. The server should use a separate thread of execution for each new client connection and all interaction between the server and the client should be done within this thread. The answer only needs to include source code for the server side application. 12 MARKSStep by Step Solution
There are 3 Steps involved in it
Step: 1
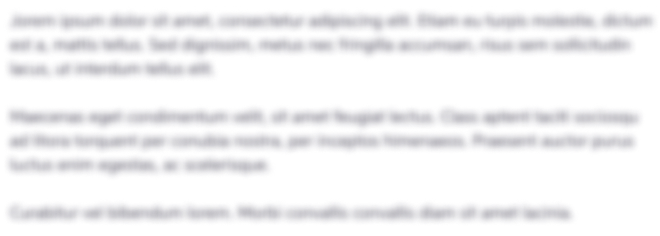
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started