Question
JAVA- I already did 3 need help with 4 Create a Program and Account class Account class should include two piece of information as attributes:
JAVA- I already did 3 need help with 4
Create a Program and Account class
Account class should include two piece of information as attributes:
- Name (name of the account holder, String, private)
- Balance (current balance of the account holder, double, private)
- Your Account class should have a setter and getter for each attribute.
- Your account name cannot be an empty string (check this condition in setter).
- You will use setter method for balance only in your constructor. So your setter method for balance should be private. Also your setter method should check if the balance is not set to negative. If it is set to negative, then print an error to the screen and keep the old balance.
- Your Account class should have a constructor that receives two parameters: name and balance. You should use setter methods to set name and balance.
- Your Account class should have a deposit method which adds deposit amount to the balance and update the balance. If the deposit amount is negative or zero, the method should print an error to the screen. The method should only update the balance when the deposit amount is valid (positive). Use setter and getter methods to access the previous balance and set the new balance.
- Your Account class should have a withdraw method which subtracts withdraw amount from the balance and update the balance. Your withdraw amount should be positive (cannot be zero or negative). Your withdraw amount cannot be greater than your current balance. Use setter and getter methods to access the previous balance and set the new balance.
- Now perform the following actions in your main method in these order.
- In your main method create two accounts for these people (Baris Manco with -10.95 dollars initial balance and Sezen Aksu with 1000.12 dollars initial balance).
- After creating these two accounts, print their name and balance to the screen using printf. E.g., "Baris Manco balance: $0.00". Make sure you are using two decimals for printing balance.
- Baris would like to deposit 100 dollars to his account. Perform the deposit for Baris and print his new balance to the screen.
- Sezen would like to withdraw 1,500 dollars from her account. Perform the withdraw and print her new balance to the screen.
- Sezen would like to withdraw 200 dollars from her account. Perform the withdraw and print her new balance to the screen.
CODE FOR QUESTION 3
public class Account { //Creates class
private String name; private double balance;
public String getName() { //Creates getName method return name; }
public void setName(String name) { //Creates setName method if (name == "") //sees if this is an empty string { this.name = "Jerry"; //sets a name } else { this.name = name; } }
public Account(String name, double balance) {//Creates a class called Account and has two parameters setName(name); //Setter method setBalance(balance); //Setter method }
public double getBalance() { //getBalance method return balance; //returns balance }
private void setBalance(double balance) { //private method if (balance < 0) { this.balance=0; //Balance equals 0 System.out.println("Error: Balance was Set Tried to Set to Negative. Balance is set to zero"); //Prints error } else { this.balance = balance; // refers to the object } } public void deposit(double depositAmount) { //creates deposit method if(depositAmount <=0){ //if statement to see if its less than 0 System.out.println("Error:Deposit Amount is Negative. So deposit action is ignored."); //Prints error } else { setBalance(getBalance()+ depositAmount); //The balance is the current + the deposit } }
public void withdraw(double withdrawAmount) { //withdraw method if(withdrawAmount <=0){ //withdraw cant be less than 0 System.out.println("Error:Withdraw Amount is Negative. So withdraw action is ignored."); //Prints error } else if (withdrawAmount>getBalance()){ //else if statement System.out.println("Error:Withdraw Amount greater than balance. So withdraw action is ignored."); } //Prints an error else { setBalance(getBalance()- withdrawAmount); //The balance will be the current- the withdraw amount } } }
Question 4 (20 points)
Create a new project and use the existing code from Question 3. You can just copy the project and start from there.
- Add transfer money method to your project. For example, if Baris would like to send 40 dollars to Sezen, you need to have a mechanism to be able to successfully perform the action. If Baris does not have enough money in his account, then it should print an error.
- Bank collects $5 fee for each successful transfer. How do you handle this situation in your transfer method? Would you also create an account for the bank and collect the fees there or do you have a better method to collect these fees? Your system should be able to print the total bank fee collections. So if there are two transfers, you should be able to print $10 to the screen.
- Where do you add this method? Program class, Account class, or another new class? Why do you add it there? Please discuss this in your report.
- Now empty your main method (if anything left from previous exercise) and perform these operations in order.
- In your main method create two accounts for these people (Baris Manco with 500.00 dollars initial balance and Sezen Aksu with 400.00 dollars initial balance).
- Baris would like to transfer 600.00 dollars to Sezen (perform the action).
- Print Baris and Sezen's new balance and print total fees collected so far.
- Sezen would like to transfer 50.52 dollars to Baris (perform the action).
- Print Baris and Sezen's new balance and print total fees collected so far.
- Baris would like to send the money she got from Sezen back to her (perform the action).
- Print Baris and Sezen's new balance and print total fees collected so far.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
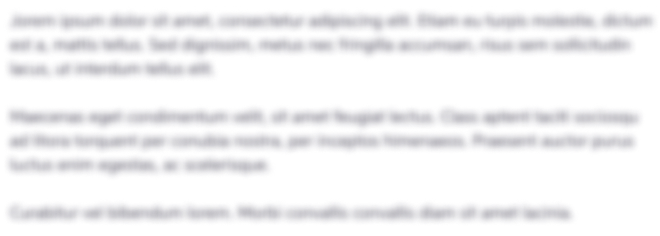
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started