Question
*JAVA* I need help with the logic of my java code. I got it throwing the correct errors, but I need the while loops to
*JAVA* I need help with the logic of my java code. I got it throwing the correct errors, but I need the while loops to work The program also needs to keep taking input while also outputting the correct error message. The question continues at the bottom of the code.
The Stack Class is as follows:
public class ArrayStack {
private int top, size; private E arrS[]; private static final int MAX_STACK_SIZE = 10;
public ArrayStack() { this.arrS = (E[]) new Object[MAX_STACK_SIZE]; this.top = size; this.size = 0; }
public void push(E value) { if (isFull() == true) { throw new ArrayIndexOutOfBoundsException("Stack is Full"); } else this.arrS[this.size++] = value;
}
public E pop() { if (isEmpty() == true) { throw new ArrayIndexOutOfBoundsException("Stack is Empty"); } return this.arrS[--this.size]; }
public boolean isFull() { return (this.size == MAX_STACK_SIZE) ? true : false;
}
public boolean isEmpty() { return (this.size == 0) ? true : false;
}
public int length() { return this.size; }
public E topValue() { if (isEmpty() == true) { throw new IllegalArgumentException(); } return this.arrS[this.size-1]; }
public void clear() { this.size = 0;
}
public String show() { StringBuilder sb = new StringBuilder(); sb.append(" "); for (int i = 0; i < this.size; i++) { sb.append(this.arrS[i]); sb.append(" ");
} sb.append(""); return sb.toString();
}
}
The driver for the stack class:
import java.util.Scanner;
public class a3main {
public static void main(String[] args) { int count = 0; int errors = 0;
Scanner scan = new Scanner(System.in);
do { System.out.println("Enter an algebraic or arithmetic expression: ");
String arg = scan.nextLine();
ArrayStack stack = new ArrayStack();
while (count < arg.length()) { for (int i = 0; i < arg.length(); i++) { count++; char current = arg.charAt(i);
if (current == '{' || current == '[' || current == '(') { try { stack.push(current); } catch (Exception e) { System.out.println("Excessive parenthesis combinatins"); errors++; } }
if ((current == '}' || current == ')' || current == ']')) { if (stack.isEmpty() == true && errors == 0) System.out.println("Excessive right parenthesis"); char last = stack.topValue(); if (current == '}' && last == '{' || current == ')' && last == '(' || current == ']' && last == '[') stack.pop(); }
} if (stack.isEmpty() == true) { System.out.println("Correct parenthesis: match"); } else { System.out.println("Excessive left parenthesis"); } } } while (count > 0); } }
I am getting the correct error while I input the following. (Keep in mind the while loops fail)
input: x=((a-b)+(c-d)
output: Excessive left parenthesis
Next input:x=(a-b))
output: Excessive right parenthesis
Next Input: x= (((((((((((a-b)))))))))))
output: "excessive parenthesis combinations" ///Program crashes
I need to keep it to silent error reporting without crashes. I have spent countless hours on this.
Can somebody explain the logic behind the code and help correct the outputs?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
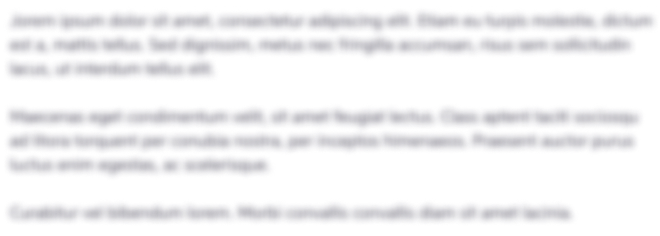
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started