Question
JAVA i need write java program I want to add products or add products to cart buy or delete from cart purchases I want to
JAVA
i need write java program
I want to add products or add products to cart buy or delete from cart purchases I want to show the products that I bought
use ArrayList
Object Classes: designing Shopping Cart
//BookInterface.java
public interface BookInterface extends ProductInterface{ public String getPublisher(); public void setPublisher(String publisher); public int getYearPublished(); public void setYearPublished(int yearPublished); }
//ElectronicsInterface.java
public interface ElectronicsInterface { public String getManufacture(); }
//ProductInterface.java
public interface ProductInterface { public double computeSalePrice(); public double getRegularPrice(); public void setRegularPrice(double regularPrice); }
//Book.java
public class Book extends Product implements BookInterface{ private String publisher; private int yearPublished; public Book(double regularPrice,String publisher,int yearPublished) { super(regularPrice); this.publisher=publisher; this.yearPublished=yearPublished; } @Override public String getPublisher() { return publisher; }
@Override public void setPublisher(String publisher) { this.publisher =publisher; }
@Override public int getYearPublished() { return yearPublished; }
@Override public void setYearPublished(int yearPublished) { this.yearPublished=yearPublished; }
@Override public double computeSalePrice() { double saleprice; //add 20% of regular price to the regular price to calculate the saleprice saleprice=this.getRegularPrice()+(this.getRegularPrice()*20/100); return saleprice; }
}
//Cartoon.java
public class Cartoon extends Book{ private String characterName; public Cartoon(double regularPrice,String publisher,int yearPublished,String characterName) { super(regularPrice,publisher,yearPublished); this.characterName=characterName; } public double computeSalePrice() { double saleprice; //add 5% of regular price to the regular price to calculate the saleprice saleprice=this.getRegularPrice()+(this.getRegularPrice()*5/100); return saleprice; } }
//ChildrenBook.java
public class ChildrenBook extends Book{ private int age; public ChildrenBook(double regularPrice, String publisher, int yearPublished,int age) { super(regularPrice, publisher, yearPublished); this.age=age; } public double computeSalePrice() { double saleprice; //add 15% of regular price to the regular price to calculate the saleprice saleprice=this.getRegularPrice()+(this.getRegularPrice()*15/100); return saleprice; } }
//Electronics.java
public class Electronics extends Product implements ElectronicsInterface{
private String manufacturer; public Electronics(double regularPrice,String manufacturer) { super(regularPrice); this.manufacturer=manufacturer; } @Override public String getManufacture() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer=manufacturer; } }
//MP3Player.java
public class MP3Player extends Electronics{ private String color;
public MP3Player(double regularPrice, String manufacturer,String color) { super(regularPrice, manufacturer); this.color=color; } public double computeSalePrice() { double saleprice; //add 40% of regular price to the regular price to calculate the saleprice saleprice=this.getRegularPrice()+(this.getRegularPrice()*40/100); return saleprice; } public String getColor() { return color; } public void setColor(String color) { this.color=color; } }
//Product.java
public class Product implements ProductInterface{ private double regularPrice; public Product(double regularPrice) { this.regularPrice=regularPrice; } @Override public double computeSalePrice() { double saleprice; //add 10% of regular price to the regular price to calculate the saleprice saleprice=regularPrice+(regularPrice*10/100); return saleprice; }
@Override public double getRegularPrice() { return regularPrice; }
@Override public void setRegularPrice(double regularPrice) { this.regularPrice=regularPrice; } }
//TV.java
public class TV extends Electronics{ private int size; public TV(double regularPrice, String manufacturer,int size) { super(regularPrice, manufacturer); this.size=size; } public double computeSalePrice() { double saleprice; //add 50% of regular price to the regular price to calculate the saleprice saleprice=this.getRegularPrice()+(this.getRegularPrice()*50/100); return saleprice; } }
//Main.java
public class Main { public Main() { } public static void main(String[] args) { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
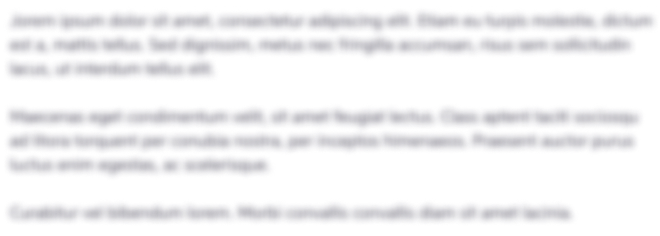
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started