Question
Java (I use Eclipse): Implement the bfs(int s) function. The program should return an integer array that records the minimum distance between every node to
Java (I use Eclipse):
Implement the bfs(int s) function. The program should return an integer array that records the minimum distance between every node to the source s.
Below is the skeleton to modify for the above question/solution.
Please make sure the program works. A screen shot of the working program and code will be helpful!
Thank you.
public class Graph {
public int n; //number of vertice
public int[][] A; //the adjacency matrix
private final int WHITE = 2;
private final int GRAY = 3;
private final int BLACK = 4;
public Graph () {
n = 0;
A = null;
}
public Graph (int _n, int[][] _A) {
this.n = _n;
this.A = _A;
}
/*
* Input: s denotes the index of the source node
* Output: the array dist, where dist[i] is the distance between the i-th node to s
*/
public int[] bfs (int s) {
}
public void print_array (int[] array) {
for (int i = 0; i < array.length; i++)
System.out.println(i + ": " + array[i]);
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
int n = 8;
int[][] A =
{{0, 1, 0, 0, 1, 0, 0, 0},
{1, 0, 0, 0, 0, 1, 0, 0},
{0, 0, 0, 1, 0, 1, 1, 0},
{0, 0, 1, 0, 0, 0, 1, 1},
{1, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 0, 0, 1, 0},
{0, 0, 1, 1, 0, 1, 0, 1},
{0, 0, 0, 1, 0, 0, 1, 0}};
Graph g = new Graph(n, A);
g.print_array(g.bfs(1));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
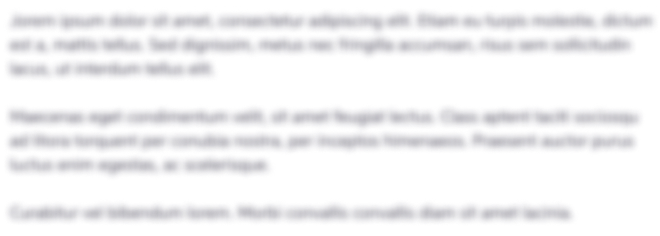
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started