Question
[JAVA] I wrote a program that created a 10x10 ocean grid, added a ship, added a keypressed handler so i could move the ship around
[JAVA]
I wrote a program that created a 10x10 ocean grid, added a ship, added a keypressed handler so i could move the ship around and added N random islands to a map that the ship cannot touch. Please help with the following step. My code is below with the assignment.
See my implementation below:
-- Ocean Explorer.java
import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Rectangle; import javafx.application.Application; import javafx.event.EventHandler; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.input.KeyEvent; import javafx.scene.layout.AnchorPane; import javafx.scene.layout.Pane; import javafx.stage.Stage;
public class OceanExplorer extends Application{
boolean[][] islandMap; Pane root; final int dimensions = 10; final int islandCount = 10; final int scalingFactor = 50; Image shipImage; ImageView shipImageView; OceanMap oceanMap; Scene scene; Ship ship; @Override public void start(Stage mapStage) throws Exception { oceanMap = new OceanMap(dimensions, islandCount); islandMap = oceanMap.getMap(); // Note: We will revisit this in a future class and use an iterator instead of exposing the underlying representation!!! root = new AnchorPane(); drawMap();
ship = new Ship(oceanMap); loadShipImage(); scene = new Scene(root,500,500); mapStage.setTitle("Christopher Columbus Sails the Ocean Blue"); mapStage.setScene(scene); mapStage.show(); startSailing(); } private void loadShipImage(){ Image shipImage = new Image("ship.png",50,50,true,true); shipImageView = new ImageView(shipImage); shipImageView.setX(oceanMap.getShipLocation().x*scalingFactor); shipImageView.setY(oceanMap.getShipLocation().y*scalingFactor); root.getChildren().add(shipImageView); } private void startSailing(){ scene.setOnKeyPressed(new EventHandler
@Override public void handle(KeyEvent ke) { switch(ke.getCode()){ case RIGHT: ship.goEast(); break; case LEFT: ship.goWest(); break; case UP: ship.goNorth(); break; case DOWN: ship.goSouth(); break; default: break; } shipImageView.setX(ship.getShipLocation().x*scalingFactor); shipImageView.setY(ship.getShipLocation().y*scalingFactor); } }); } // Draw ocean and islands public void drawMap(){ for(int x = 0; x
--OceanMap.java--
import java.awt.Point; import java.util.Random;
// This class is responsible for generate a grid representing the island map // and randomly placing islands onto the grid. public class OceanMap { boolean[][] islands; int dimensions; int islandCount; Random rand = new Random(); Point shipLocation; // Constructor // Not adding validation code so make sure islandCount is much less than dimension^2 public OceanMap(int dimensions, int islandCount){ this.dimensions = dimensions; this.islandCount = islandCount; createGrid(); placeIslands(); shipLocation = placeShip(); } // Create an empty map private void createGrid(){ islands = new boolean[dimensions][dimensions]; for(int x = 0; x 0){ int x = rand.nextInt(dimensions); int y = rand.nextInt(dimensions); if(islands[x][y] == false){ islands[x][y] = true; islandsToPlace--; } } } private Point placeShip(){ boolean placedShip = false; int x=0,y=0; while(!placedShip){ x = rand.nextInt(dimensions); y = rand.nextInt(dimensions); if(islands[x][y] == false){ placedShip = true; } } return new Point(x,y); }
public Point getShipLocation(){ return shipLocation; } // Return generated map public boolean[][] getMap(){ return islands; } public int getDimensions(){ return dimensions; } public boolean isOcean(int x, int y){ if (!islands[x][y]) return true; else return false; }
--Ship.java--
import java.awt.Point;
public class Ship { Point currentLocation; OceanMap oceanMap; public Ship(OceanMap oceanMap){ this.oceanMap = oceanMap; currentLocation = oceanMap.getShipLocation(); } public Point getShipLocation(){ return currentLocation; } public void goEast(){ if(currentLocation.x -- Design.ucls --
Step by Step Solution
There are 3 Steps involved in it
Step: 1
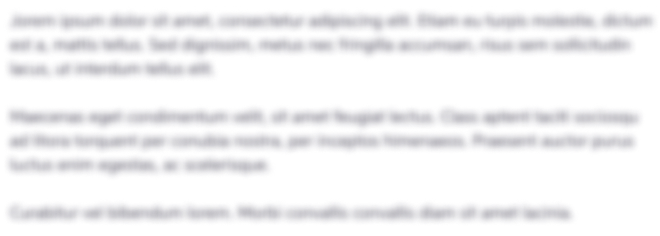
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started