Question
Java II Programming Inheritance Read this entire handout before starting! The description of the assignment sounds worse than the actual work. Files are provided to
Java II Programming Inheritance Read this entire handout before starting! The description of the assignment sounds worse than the actual work. Files are provided to get you started. Purpose: To illustrate class inheritance. Concentrate on creating the bug classes properly. Review use of two-dimensional arrays Consider the UML class diagram for the Bug hierarchy below: Some Driver Program Bug (Abstract Class: Below is the minimal info) Main (args: String[ ]): void # name: String # image: ImageIcon # direction: String # distance: int # row: int # column: int + getName( ): String + getImage( ) : ImageIcon + getDirection( ) : String + getDistance( ): int + getRow( ): int + getColumn( ): int + setName(String name): void + setImage(ImageIcon image) : void + setDirection (String bugDirection) : void + setDistance(int bugDistance): void + setRow(int Row): void + setColumn(int column): void + canMove( GridPanel bugGrid, int newR, int newC) : Boolean + move(GridPanel bugGrid ): void + toString( ) : String Crawler Flyer BigCrawler Notes: Bug is an abstract class. Crawler and Flyer are inherited from Bug. BigCrawler is inherited from Crawler. NOTICE: The methods in the Bug class named canMove( ) and move( ) are abstract methods. This means they will need to be included and defined in the bug classes you create. Files Provided There is a set of files (zipped and in Blackboard) that you can use to get started: BugsDriver: A GUI driver that will display the GUI panel(s) used for this assignment. You are NOT to change this file. This is the file you execute to play the game. GridPanel: A grid layout of an array of buttons (defaults to 8x8 if size is not provided) that will be used for the game board. You are NOT to change this class. BorderPanel: A border layout that includes 3 buttons (play the game, stop the game, move the bugs) in the northern region of the layout and an instance of the GridPanel class in the center region. You WILL need to modify class to instantiate new bugs you create and want to move. Bug: This is a parent class of bugs. Look over this carefully. It contains 2 abstract classes that you will need to include in the bug classes you create which are inherited from Bug. Crawler: This is a child class of Bug. A crawler always moves 1 space left and right (East and West on the grid). 3 images you can use for the bugs: Crawler.png, Flyer.png, and BigCrawler.png You will create the Crawler, Flyer, and BigCrawler classes and modify BorderPanel to place the bugs on the board and move them. Test the files: Compile the five Java files above and run BugsDriver. Review each of the Java files to make sure you understand how they are set up. Pay attention to the Bug class (parent class) and Crawler (a child class of Bug). Look at how they relate to one another. Pay attention to the use of super() in the child class. Look at the BorderPanel. Pay attention to how it instantiates a Crawler object (there are 2 of them) and moves each. You will create a new child class of Bug named Flyer. Once created, you will need to modify BorderPanel to instantiate a Flyer bug and move it. Notice BorderPanel has an illustration of how to display an image on a button (placing a bug on the board). Overview of this Assignment: Think of this assignment as part of a game. There is a playing board with rows and columns of spaces (8x8 for our assignment). This has been provided for you in the GridPanel class. You are not to modify this file. It is an array of JButton objects. Any JButton object can display an image on the face of the button. 0 1 2 3 4 5 6 7 0 1 2 3 4 5 6 7 Bugs will appear on the board at random locations. Several bugs can be on the board at the same time. When a bug is first instantiated, it should be placed on the board at a random location. Use a random number generator to set the location (row and column) for each bug when it is instantiated. (You can refer to the examples for the Crawler bugs.) Each square on the board can hold at most one bug. Some squares will not have any bugs. The canMove() and move() methods in the Bug class are abstract. This means any child class of Bug needs to include these methods to describe how that type of bug moves and if it can move. You can refer to the Crawler class for an example of a child class defining these methods. They are defined in Bug as: public abstract boolean canMove(GridPanel bugGrid, int newRow, int newColumn); public abstract void move(GridPanel bugGrid); The canMove() method simply looks at where the bug is and determines if it can move in its prescribed direction and distance (considering the edges of the board and whether or not another bug occupies the proposed new location). Look at its parameters above: the grid and the new column and new row for a bug. These will help you determine if the bug can move to the new position. The canMove( ) method returns a true value if the bug can move and a false value if it cannot move. It does not actually move the bug. Do not change the purpose of this method. Notice the parameter: the grid. This will help you actually look at a specific location on the grid. The move( ) method will actually move the bug (if canMove() is true). Each class of bug moves about the board in a different fashion. o Crawler objects move East or West (Left or right) one space. When on the edge of the board, this bug reverses direction. o Flyer objects travel North or South (up or down) 1 space. When on the edge of the board or about to fall off, this bug reverses direction. o Flyer objects move diagonally 1 space moving in a NorthEast to SouthWest direction (top right to bottom left). When on the edge of the board, this bug reverses direction (bottom left to top right). Dont forget: when a bug moves, you need to remove the older image from the board update the new position to show the bug update the row/column of the bug If a bug is trying to move to a position where another bug is resting: o The bug who is trying to move just stays where it is This is not a hard assignment because a sample child class has been provided as an example and guide. Program Requirements (Remember this is primarily an assignment on inheritance): CREATE THE NEW CLASSES: 1. Review the Bug class to make sure you understand it. 2. Review the Crawler class to make sure you understand it. 3. Create the Flyer Class using Crawler as an example: Remove legs from the instance data items. We will not assume all flyer bugs have legs. Add another instance data item that would be specific to flying bugs. Make sure the constructors give value to the new instance data item. Do not duplicate code in the parent class. Like the Crawler class, use the super() reference to give values to data items inherited from the parent. Create accessor(s) and mutator(s) for the new instance data items in each class. Create any additional methods you deem necessary. Define canMove() and move() for this class. Here is how Flyer bugs move: o 1 space North or South (depending upon how the bug was instantiated). The flyer bugs do not move into a space occupied by another bug. Compile and debug this class. 4. Modify the BorderPanel class: When the Start Game button is clicked: o All of the bugs should appear on the board in different locations. When the Move Bugs button is clicked: o Each bug moves 1 space as that type of bug should move (unless another bug is in its way) o Make sure the user can see the bug movement on the board. o Make sure the image is removed from each bugs old location (unless the bug couldnt move). o You can click this multiple times to see the bugs continue to move. When the Stop Game button is clicked: o Close the application NOTE: When doing the above, do not forget to: Using the keyword super, issue calls to the parent constructors/methods appropriately. (This is often missed by students.) It is handy especially in the constructors and toString() methods. Use privacy modifiers (public, private, protected) appropriately. (This is often missed by students.) Extra credit (3 points) Create the BigCrawler bug which inherited from Crawler. It move 1 spaces NorthEast to Southwest (both row and column change as it moves diagonally).
BorderPanel.java
//********************************************************************
// BorderPanel.java Java Foundations
//
// A border panel to hold the buttons and the bugs game board.
// This is the main panel that students will use.
//
// THIS IS AN INCOMPLETE CLASS - STUDENTS MODIFY THIS FOR HOMEWORK
// BY INSTANTIATING NEW BUGS, PLACING THEM ON THE BOARD, AND MOVING
// THEM.
//********************************************************************
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
public class BorderPanel extends JPanel
{
//-----------------------------------------------------------------
// Sets up this panel with a button in each area of a border
// layout to show how it affects their position, shape, and size.
//-----------------------------------------------------------------
private JPanel buttonPanel = new JPanel();
private JButton startButton = new JButton("Start the Game");
private JButton stopButton = new JButton("Stop the Game");
private JButton moveButton = new JButton("Move the Bugs");
private ButtonListener listener = new ButtonListener();
private GridPanel bugGrid;
private ImageIcon flyerImage = new ImageIcon("Flyer.png");
private ImageIcon crawlerImage = new ImageIcon("Crawler.png");
private ImageIcon bigCrawlerImage = new ImageIcon("BigCrawler.png");
private Crawler crawlerBug1;
private Crawler crawlerBug2;
private final static int BOARD_ROWS = 8;
private final static int BOARD_COLUMNS = 8;
public BorderPanel()
{
bugGrid = new GridPanel(BOARD_ROWS, BOARD_COLUMNS);
//--------------------------------------------------
// Create a simple panel for the three buttons
//--------------------------------------------------
stopButton.addActionListener(listener);
startButton.addActionListener(listener);
moveButton.addActionListener(listener);
buttonPanel.add(startButton);
buttonPanel.add(stopButton);
buttonPanel.add(moveButton);
//--------------------------------------------------
// Use a BorderLayout for the game
//--------------------------------------------------
setLayout(new BorderLayout());
setBackground(Color.green);
//--------------------------------------------------
// Add the buttonPanel and the grid to the
// BorderPanel
//--------------------------------------------------
add(buttonPanel, BorderLayout.NORTH);
add(bugGrid, BorderLayout.CENTER);
}
private class ButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
if (event.getSource() == stopButton) {
System.exit (0); }
if (event.getSource() == startButton) {
//------------------------------------------------------------
// Sample of placing 2 Crawler bugs on the grid at a random
// locations.
//------------------------------------------------------------
Random generator = new Random();
crawlerBug1 = new Crawler("Spider", crawlerImage, "East", 8);
int row = generator.nextInt(bugGrid.getBoardRows()) ;
int column = generator.nextInt(bugGrid.getBoardColumns());
//------------------------------------------------------------
// First crawler bug is placed on the board
//------------------------------------------------------------
while (bugGrid.containsToken(row,column))
{ row = generator.nextInt(bugGrid.getBoardRows()) ;
column = generator.nextInt(bugGrid.getBoardColumns()); }
crawlerBug1.setRow(row);
crawlerBug1.setColumn(column);
bugGrid.addImage(crawlerImage,crawlerBug1.getRow(), crawlerBug1.getColumn());
//------------------------------------------------------------
// Second crawler bug is placed on the board
//------------------------------------------------------------
crawlerBug2 = new Crawler("Creepy Crawler", crawlerImage, "West", 22);
row = generator.nextInt(bugGrid.getBoardRows()) ;
column = generator.nextInt(bugGrid.getBoardColumns());
while (bugGrid.containsToken(row,column))
{ row = generator.nextInt(bugGrid.getBoardRows()) ;
column = generator.nextInt(bugGrid.getBoardColumns()); }
crawlerBug2.setRow(row);
crawlerBug2.setColumn(column);
bugGrid.addImage(crawlerImage,crawlerBug2.getRow(), crawlerBug2.getColumn());
}
//------------------------------------------------------------
// You should instantiate and place a flyer bug on the board
// after this comment
//------------------------------------------------------------
if (event.getSource() == moveButton) {
//------------------------------------------------------------
// Sample of moving a bug on the grid
//------------------------------------------------------------
crawlerBug1.move(bugGrid);
crawlerBug2.move(bugGrid);
//------------------------------------------------------------
// You should move the flyer bug after this comment
//------------------------------------------------------------
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
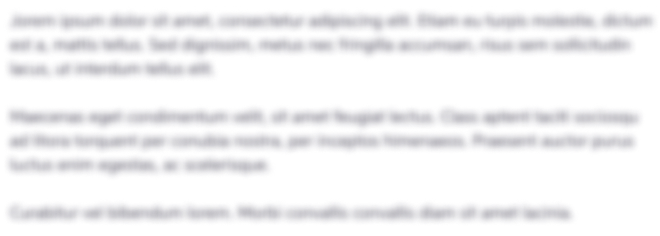
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started