Question
JAVA ILLUMINATED An Active Learning Approach 3 rd Edition. CHAPTER 8 8.10.6 54. Write a method that returns the difference between the largest and smallest
JAVA ILLUMINATED
An Active Learning Approach 3rd Edition.
CHAPTER 8
8.10.6
54. Write a method that returns the difference between the largest and smallest elements in an array of doubles.
public class ArrayDifference { public static void main( String [] args ) { double [] array = { 72.3, 6.7, 12.6, 40.7, 21.8, 49.9 }; System.out.print( "The elements are " ); for ( int i = 0; i < array.length; i++ ) System.out.print( array[i] + " " ); System.out.println( ); System.out.println( "The difference between the largest and " + "smallest elements is " + largestSmallestDifference( array ) ); } // Insert your code below }
58. Write a method that prints all the elements of an array of chars in reverse order.
public class PrintReverseArray { public static void main( String [] args ) { char [] array = { 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h' }; System.out.print( "The elements are " ); for ( int i = 0; i < array.length; i++ ) System.out.print( array[i] + " " ); System.out.println( ); System.out.print( "The elements in reverse order are " ); arrayReverse( array ); System.out.println( ); } // Insert your code below }
59. Write a method that returns an array composed of all the elements in an array of chars in reverse order.
public class ReturnReverseArray { public static void main( String [] args ) { char [] array = { 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h' }; System.out.print( "The elements are " ); for ( int i = 0; i < array.length; i++ ) System.out.print( array[i] + " " ); System.out.println( ); char [] arrayReversed = arrayReverse( array ); System.out.print( "The elements in reverse order are " ); for ( int i = 0; i < arrayReversed.length; i++ ) System.out.print( arrayReversed[i] + " " ); System.out.println( ); } // Insert your code below }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
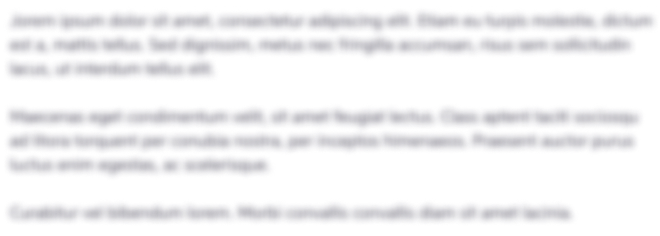
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started