Answered step by step
Verified Expert Solution
Question
1 Approved Answer
( Java ) implement the radix sort that can sort a file contains either all positive integers or a file contains mixture of positive and
Java implement the radix sort that can sort a file contains either all positive integers or a file
contains mixture of positive and negative integers.
Input: one input txt file. for hard code file name.
inFile use args : a text file contains a list of integers may contain negative numbers
Outputs:
a outFileargs :
The input data, one data per textline.
The entire hash table after each iteration move from one hash table to the other hash table.
Print the result of the sorted data, one data per textline.
b deBugFile use args : For all debugging prints.
Data Structures
listNode class
int data
listNode next
Methods:
constructor datacreate a listNode node for data and make sure assign nodes next null
LLQueue class
listNode head
listNode tail
Methods:
constructor
insertQ table index, newNode insert the newNode after the tail of hashTabletableindex
hashTabletableindextail.next newNode
hashTabletableSizetail newNode
listNode deleteQ if Q is not empty, deletes and returns the node after the dummy
bool isEmpty Returns true if tail head, returns false otherwise.
printQueue whichTable index, File File can be outFile or deBugFile.
Print to File the entire linked list in hashTablewhichTableindex
For example, if whichTable is and index is then print
Table : NULL NULL
A RadixSort class:
int tableSize set to
LLQueue hashTabletableSizeD arrays size of of linked listqueues with dummy nodes.
Initially, create a new listNode for each hashTableij to point to and set hashTableijs head and
tail point to the dummy node.
int data
int currentTable either or
int previousTable either or
int maxLength the number of digits of the largest integer to control the number of iterations of Radix sort
int offSet if there is no negative integer in the data, offSet will be zero; otherwise, the offset is the absolute
value of the smallest negative integer in the data;
the offSet will add to each data before radix sort and subtract off afterward.
int digitPosition The digit position of data that is currently processing, initialize to
int currentDigit the digit which is currently used for sorting.
Methods:
constructor Creates hashTabletableSize
Use loops to create LLQueue for each hashTableij i to and j to where
make a new listNode as dummy node for each hashTableij point to and initially, head and tail are
also point to dummy node.
preProcessing Read from input file; determine the largest and smallest integers in the file
and establishes offset. See algorithm below. You may include this method in constructor!
RSort Performs Radix sort; sorts data dogot from righttoleft. See algorithm below.
The algorithm is exactly the same as taught in class.
int getLength data Determines and returns the length of data.
suggestion: convert data to string, then returns string length.
int getIndex data positionThis method is the hash function that determines and returns the digit at the
position of data, which is the index of the hash table for insertion. For example,
if data is length is ; data position is ; is ; is ; is and is
printTable whichTable File On your own!
Call printQueue to print each none empty queue in hashTablewhichTable out to File.
printSortedData whichTable outFile Output the final result of sorted data.
Print nodes data in none empty queue in hashTablewhichTableindex index to tableSize
numbers per textline with space in between two numbers.
make sure to subtract offSet before printing the data.
main
Step : inFile open via args
outFile open via args
deBugFile open via args
hashTabletableSize create by RadixSort constructor
Step : preProcessing inFile deBugFile
Step : close inFile
reopen inFile.
Step : RSort inFile outFile deBugFile
Step : close all files
preProcessing inFile deBugFile
Step : deBugFile Entering preProcessing
negativeNum
positiveNum
Step : data read one integer from inFile
if data negativeNum
negativeNum data
if data positiveNum
positiveNum data
Step : repeat step until inFile is empty
Step : if negativeNum
offSet abs negativeNum
else
offSet
Step : positiveNum positiveNum offset
maxLength getLength positiveNum
Step : deBugFile In preProcessing : negativeNum offSet positiveNum maxDigits print values
Step : deBugFile Leaving preProcessing
Step by Step Solution
There are 3 Steps involved in it
Step: 1
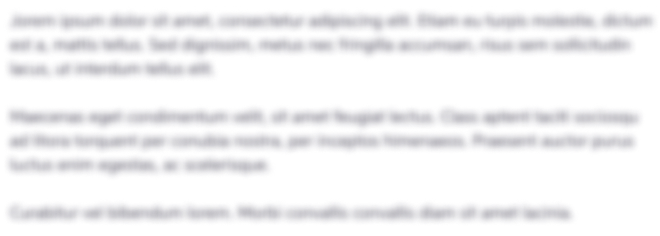
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started